Ways to Create a PDF on Android
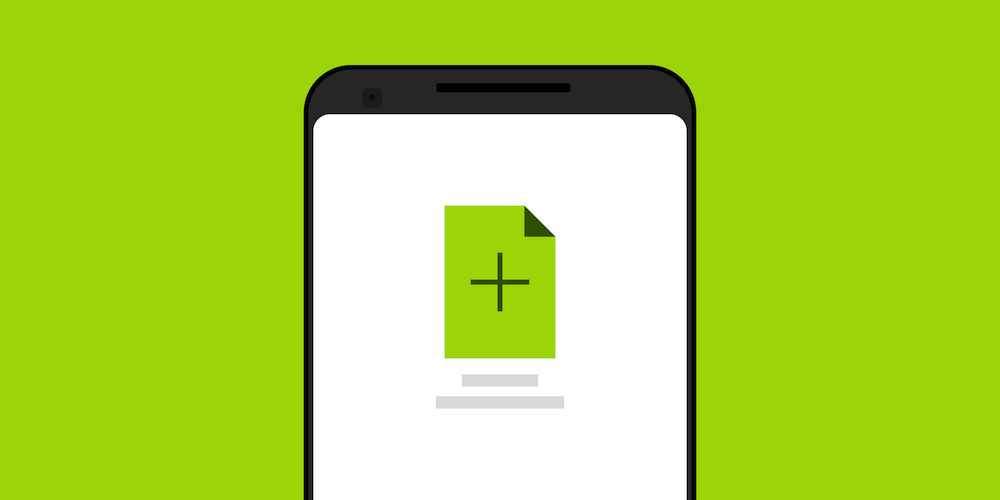
You can use our Android PDF SDK for viewing and editing already existing PDF documents, but have you wondered how to create a PDF on Android?
In this blog post, we’ll first look at two ways users can create PDFs in other apps. Then, we’ll see how your app can use the PdfProcessor
class from PSPDFKit to generate blank PDFs and customize their content.
Exporting a PDF from an App That Supports It
Many apps allow you to export a PDF. For example, both Google Docs and Microsoft Word can do this.
Using the Print Feature
The Android print feature allows you to save any printable content as a PDF.
To create a PDF from the print preview, select Save as PDF as the target printer.
The image below shows what this will look like.
Now that we’ve looked at the options a user has for creating PDFs, let’s look at some ways you can programmatically create PDFs in your apps.
Creating a Blank PDF with PSPDFKit’s Android PDF Library
With our Android PDF Generation library, it’s easy to create a PDF with empty pages. The page can have a patterned background or even an image background. An example of this can be found in our free PDF Viewer app by tapping the + button in the document browser.
Here’s how to create a PDF while programmatically specifying the page size and appearance:
val targetFile: File val newPage = NewPage.emptyPage(Size(595f, 842f)) .backgroundColor(Color.LTGRAY) .build() val pdfProcessorTask = PdfProcessorTask(newPage) try { val processor = PdfProcessor.processDocument(pdfProcessorTask, targetFile) } catch (ex: Exception) { // Couldn't create PDF file. }
To let the user choose the page size and appearance using the same user interface as PDF Viewer, use NewPageDialog
:
NewPageDialog.show(supportFragmentManager,null, object : NewPageDialog.Callback { override fun onDialogConfirmed(newPage: NewPage) { // Use `PdfProcessor` like above. } override fun onDialogCancelled() { // User didn't select a template. } })
Creating a PDF by Drawing onto a Canvas
For maximum flexibility, you can also write your own drawing code to generate PDFs. When doing this, you need to take care to correctly handle line and page breaks.
Here’s a simple example that draws a line directly across the document:
val targetFile: File val pageSize = Size(595f, 842f) val newPage = NewPage.emptyPage(pageSize) .backgroundColor(Color.LTGRAY) .build() val pdfProcessorTask = PdfProcessorTask(newPage) pdfProcessorTask.addCanvasDrawingToPage(PageCanvas(pageSize) { canvas -> val paint = Paint() // Will draw a line from one corner of the page to the other. canvas.drawLine(0f, 0f, pageSize.width, pageSize.height, paint) }, 0) try { val processor = PdfProcessor.processDocument(pdfProcessorTask, targetFile) } catch (ex: Exception) { // Couldn't create PDF file. }
If you already have some code that draws onto a canvas, you can reuse that drawing code (with some noted exceptions).
Conclusion
In this post, you saw how users can create PDFs in existing apps by using the export and print features. You also learned how you can add PDF creation as a feature in your own app.
If your app requires advanced PDF functionality, such as adding digital signatures, filling PDF forms, and working with PDF annotations, you should look at using a commercial library.
Our Android PDF library comes with more than 30 out-of-the-box features and has well-documented APIs to handle complex use cases. Try our PDF library using our free trial, and check out our demos to see what’s possible.