How to Open a PDF in React Native Using the Document Picker
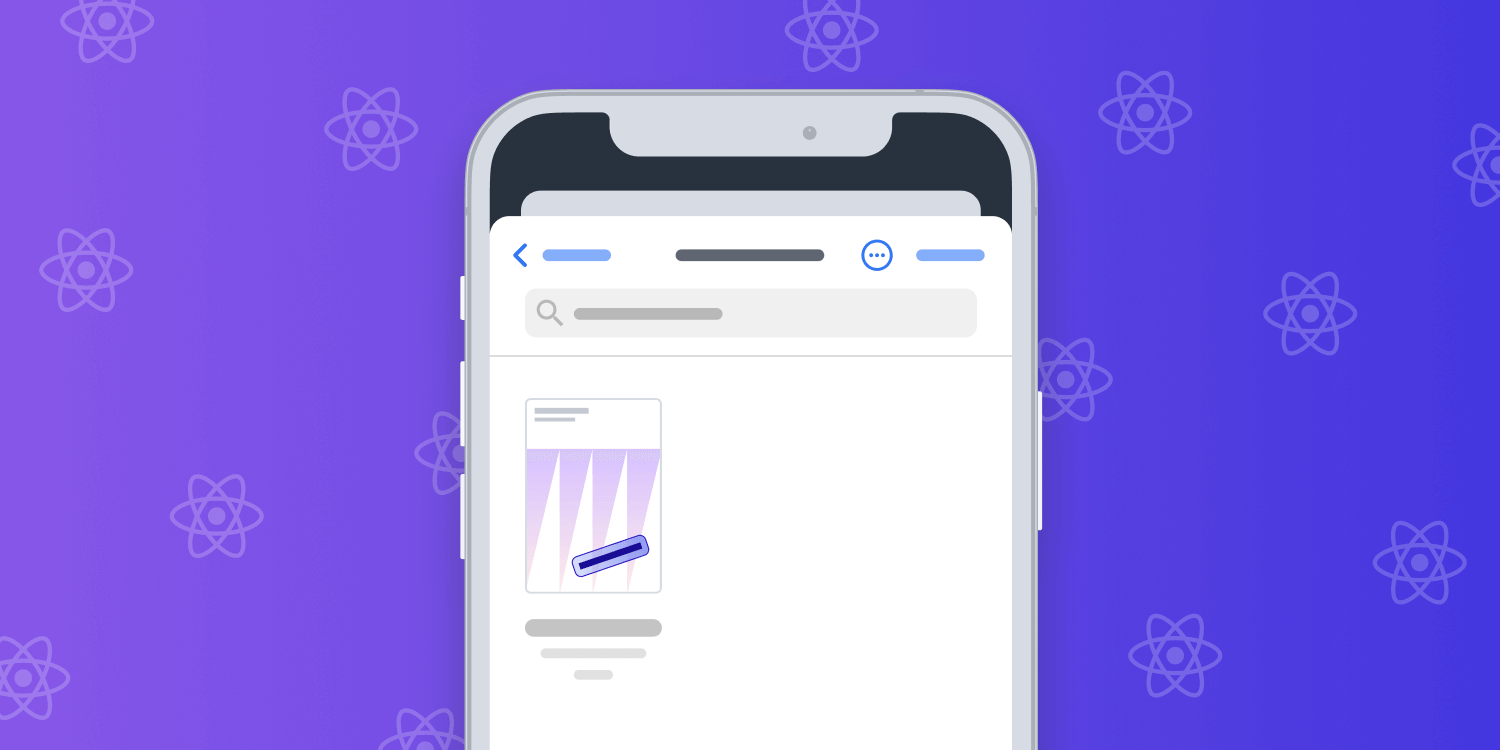
Our React Native library enables you to open a PDF document by providing a document URL. In this article, we’ll go through all the steps of creating a React Native project that allows end users to open PDFs via the system document browser.
Using the system document browser to allow your end users to open any document from their device in your application — including files located in cloud storage providers like Google Drive and iCloud Drive — can be very useful. For example, a user can download a PDF onto their mobile device and later open and view it directly in your app.
The Use Case
This tutorial uses a project in which we integrated the PSPDFKit for React Native and the React Native Document Picker (react-native-document-picker
) dependencies. These allow our end users to open documents located on their devices using the system document browser.
In the video below, you can see how we can open a PDF file from an iOS device’s local storage:
Now let’s take a look at the steps involved!
Step 1: Get a Running React Native Project
This post assumes you’re familiar with React Native and that you’ve already integrated PSPDFKit into your app. If that isn’t the case, you can use our getting started guides to create a new React Native project that uses PSPDFKit. Or, if you’re in a hurry, you can download our ready-to-run example project.
Step 2: Add the Required Dependencies
-
In the terminal app, change the location of the current working directory inside the project:
cd path/to/YourProject
-
Add the document picker dependency:
yarn add react-native-document-picker
-
Install all the dependencies for the project:
yarn install
Step 3: Open a PDF Document Using the Document Picker
Next, make sure you have a document on your device or simulator. If you’re using an Android emulator or iOS simulator, you can drag the PDF document into it. You can use this Quickstart Guide PDF as an example.
And finally, replace the entire contents of your App.js
file with the following code snippet:
import React, { Component } from 'react'; import { AppRegistry, Button, NativeModules, StyleSheet, View } from 'react-native'; import DocumentPicker from 'react-native-document-picker'; import PSPDFKitView from 'react-native-pspdfkit'; export default class DocumentBrowserExample extends Component<Props> { pdfRef: React.RefObject<PSPDFKitView>; const PSPDFKit = NativeModules.PSPDFKit; PSPDFKit.setLicenseKey(null); constructor(props) { super(props); this.pdfRef = React.createRef(); this.state = { document: null, }; } render() { return ( <View style={{ flex: 1 }}> {this.state.document == null ? ( <View style={styles.container}> <Button onPress={async () => { try { const file = await DocumentPicker.pick({ type: [DocumentPicker.types.pdf], copyTo: 'documentDirectory', }); this.setState({ document: decodeURI( file.fileCopyUri.replace('file://', ''), ), }); } catch (error) { if (DocumentPicker.isCancel(error)) { // The user canceled the document picker. } else { throw error; } } }} title="Open a PDF Document..." /> </View> ) : ( <PSPDFKitView ref={this.pdfRef} // Set the document. document={this.state.document} // Show the back button on Android. showNavigationButtonInToolbar={true} // Show the back button on iOS. showCloseButton={true} // The configuration is optional. configuration={{ showThumbnailBar: 'scrollable', }} // Set the document to `null` on Android. onNavigationButtonClicked={(event) => { this.setState({ document: null }); }} // Set the document to `null` on iOS. onCloseButtonPressed={(event) => { this.setState({ document: null }); }} style={{ flex: 1 }} /> )} </View> ); } } const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center', backgroundColor: '#F5FCFF', }, }); AppRegistry.registerComponent( 'DocumentBrowserExample', () => DocumentBrowserExample, );
That’s all! Your app now supports opening PDF documents from your device’s local storage using the system document browser!
Conclusion
We hope this post will help you implement opening PDF documents using the system document browser in your React Native project. If you have any questions about PSPDFKit for React Native, please don’t hesitate to reach out to us. We’re happy to help!