How to Build a Cordova PDF Viewer
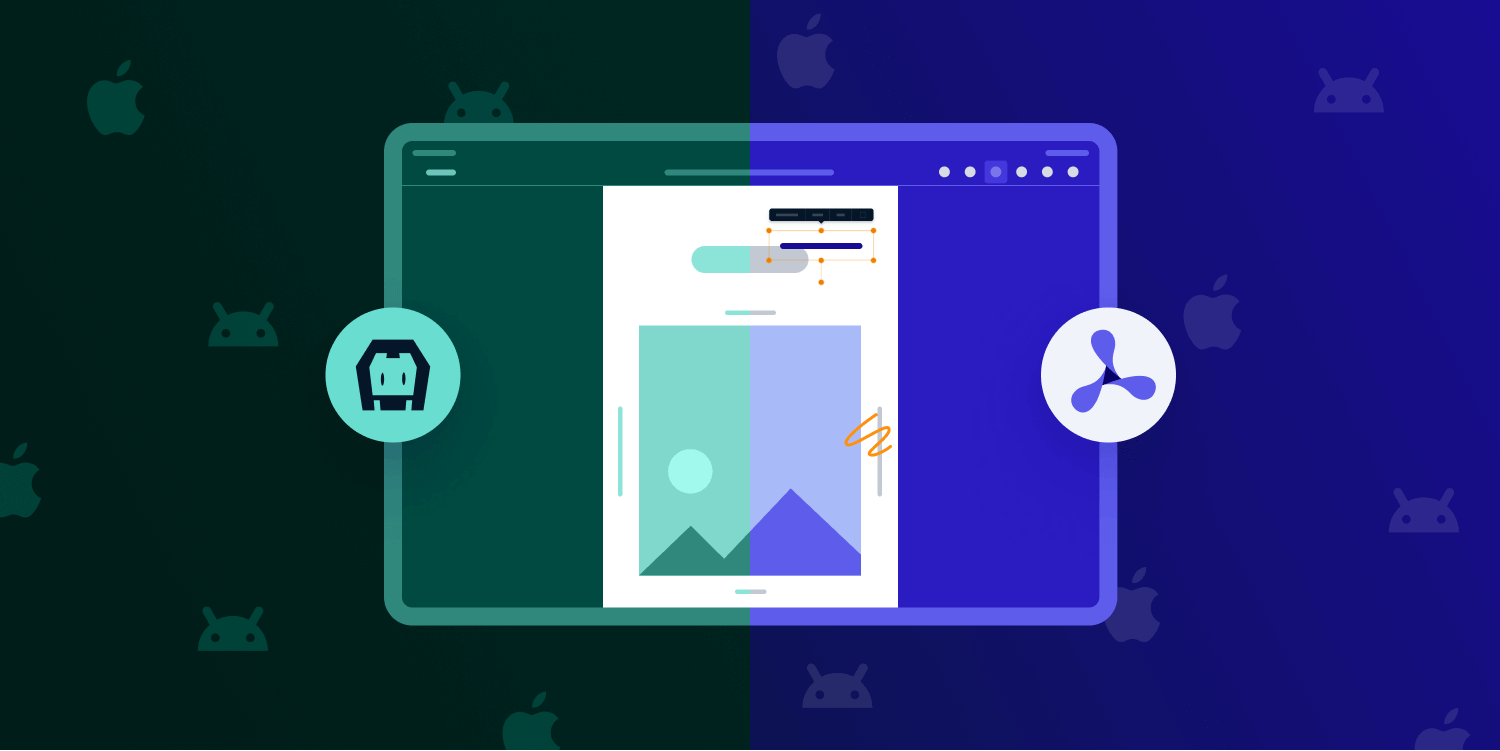
Cordova is a hybrid technology that allows you to create cross-platform apps using a single codebase in JavaScript. In this article, we’ll use Cordova and a few plugins to add support for creating a new PDF viewer application that allows opening and showing PDFs directly in your Android or iOS app.
First, we’ll look at using the open source File Opener Plugin. It can open different file types, including PDFs, and show them onscreen.
Later, we’ll dive into using PSPDFKit as an alternative approach to adding more PDF features — like the ability to annotate and edit documents — for more advanced use cases and increased functionality.
Step 1 — Installing the Prerequisites
Using Cordova requires a few tools to be installed before we can start creating a new app. Here are instructions on what tools you need and how to install them:
-
A development environment set up for running Cordova projects
Additional tools vary depending on if you want to run the app on Android, iOS, or both.
Find and follow the instructions for installing tools for running on Android here:
-
The Android NDK
-
An Android Virtual Device or a hardware device
Find and follow the instructions for installing tools for running on iOS here:
Step 2 — Creating a New Cordova App
To create our PDF viewer in Cordova, we first need to create a new Cordova project using the following command:
cordova create CordovaPDFViewer com.cordovapdfviewer.app CordovaPDFViewer
For the rest of the tutorial, we’ll work inside the CordovaPDFViewer
directory:
cd CordovaPDFViewer
Step 3 — Setting Up Dependencies
Next, we’ll set up the dependencies that we’ll use for our PDF viewer application. Open config.xml
to enable AndroidX and change the deployment target to iOS 14 or later:
... <platform name="android"> + <preference name="AndroidXEnabled" value="true" /> <allow-intent href="market:*" /> </platform> <platform name="ios"> <allow-intent href="itms:*" /> <allow-intent href="itms-apps:*" /> + <preference name="deployment-target" value="14.0" /> ... </platform> ...
Then, add the Android and iOS platforms to the project:
cordova platform add android cordova platform add ios
After, add the File Opener Plugin, along with a plugin for easier support for handling file paths:
cordova plugin add cordova-plugin-file-opener2 cordova plugin add cordova-plugin-file
Finally, add the PDF document you want to display in your project’s www
directory. You can use this magazine document as an example:
cp ~/Downloads/Document.pdf www/Document.pdf
Step 4 — Writing the App
Now that our project is set up and everything is configured correctly, we can start writing code to implement the PDF viewer.
Loading Documents
Open the index.js
file, which is used as the main entry point of the app:
open www/js/index.js
Next, specify the document path, and open the PDF using the File Opener Plugin. Modify the onDeviceReady
function, like so:
onDeviceReady: function() { this.receivedEvent('deviceready'); const documentPath = cordova.file.applicationDirectory + "www/Document.pdf" cordova.plugins.fileOpener2.open( documentPath, 'application/pdf', { error: function(e) { console.log('Error status: ' + e.status + ' - Error message: ' + e.message); }, success: function() { console.log('file opened successfully'); } } ); }
Now, run the app. You can deploy the app to the Android or iOS simulator using the following commands.
For running on Android:
cordova emulate android
For launching the app in the iOS simulator:
cordova emulate ios
This results in the app opening and the PDF being presented, as shown here in the iOS simulator:
As you can see, there are some limitations to this approach, namely that the File Opener Plugin only supports the ability to show PDFs, and you can’t edit, search, or programmatically process them.
That said, this solution is a good idea if you want to have simple support for showing PDFs, without any functionality beyond displaying documents.
Opening a PDF with PSPDFKit
To offer a more feature-rich PDF experience in your app, you can also use PSPDFKit.
To do this, remove all the platforms from your project to properly propagate the new plugin addition throughout the project, add the PSPDFKit Cordova plugin, and re-add the platforms, like so:
cordova platform remove android cordova platform remove ios cordova plugin add https://github.com/PSPDFKit/PSPDFKit-Cordova.git cordova platform add android cordova platform add ios
Then, modify the onDeviceReady
function in the index.js
file to replace the File Opener Plugin with PSPDFKit:
onDeviceReady: function() { this.receivedEvent('deviceready'); const documentPath = cordova.file.applicationDirectory + "www/Document.pdf" PSPDFKit.present(documentPath); }
This will change the PDF presentation method to use PSPDFKit, which already comes with more features — like annotating and table of contents support — out of the box:
The Cordova library for PSPDFKit also supports additional customization options, like changing the page transition mode and changing the background color:
PSPDFKit.present(documentPath, { pageTransition: 'scrollContinuous', backgroundColor: 'black', });
It’s also possible to programmatically switch pages by setting a specific page index:
PSPDFKit.setPage(3, true);
Conclusion
As you saw, adding PDF support to your app using the File Opener Plugin isn’t difficult. However, in doing so, you’re missing out on a lot of functionality. Meanwhile, PSPDFKit ships with many features out of the box, providing your users with a better user experience. PSPDFKit also comes with great customer support, so please reach out to us if you have any questions about our Cordova integration.
Feel free to download and explore the source code for the entire project. To run the project, you’ll need to enable the Android or iOS platform, or both, which is done with the cordova platform add
command shown earlier in the post.