Open a PDF in an Android App
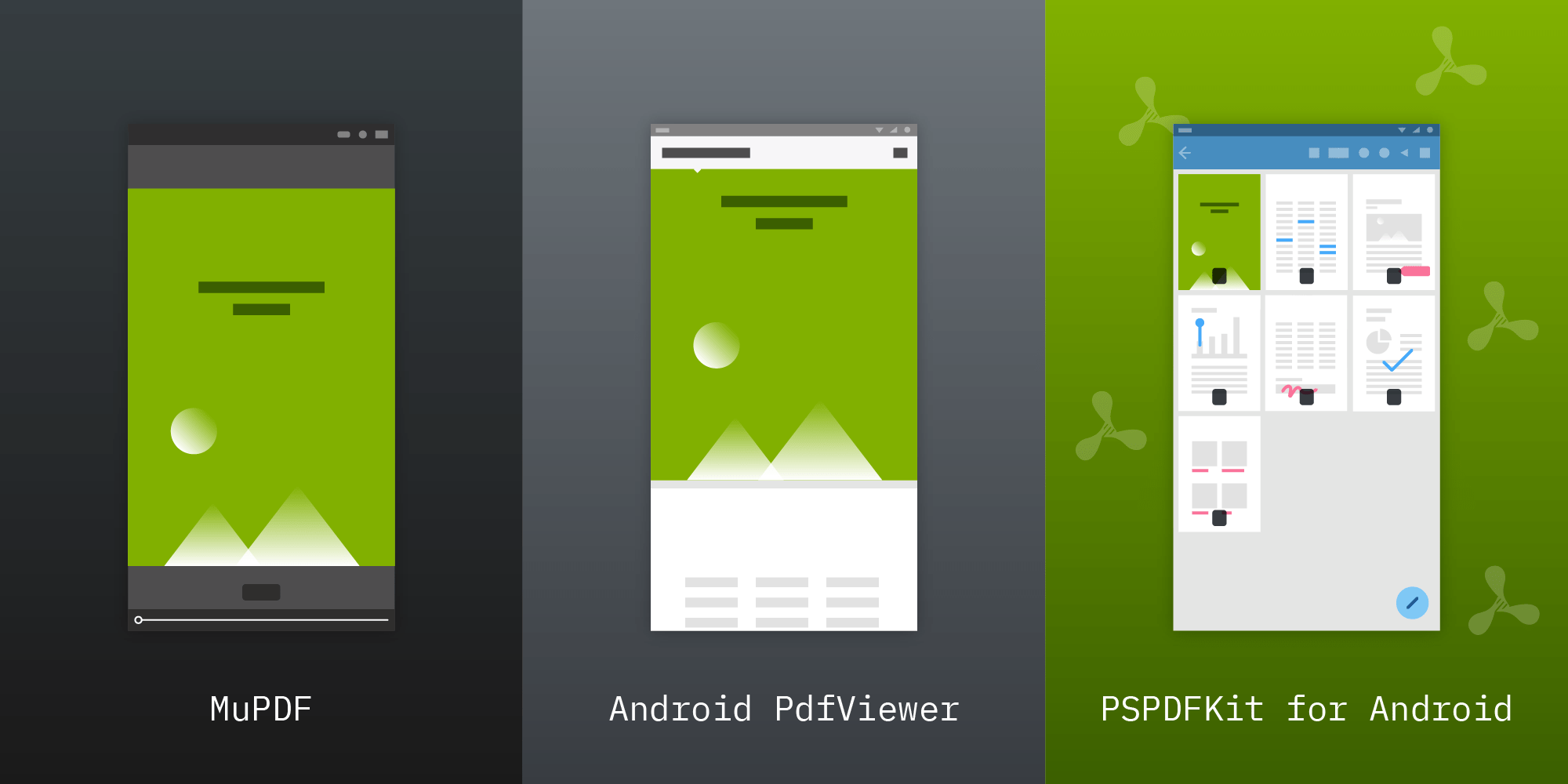
PDF documents are the de facto standard for archiving, transferring, and presenting documents online. Android developers frequently need to display PDF documents within their applications, so in this article, we’ll look at different solutions for doing exactly that.
We’ll begin with the basic PDF support provided by the Android SDK library, move on to MuPDF, and finally look at rendering PDFs with the Android PdfViewer library.
Displaying PDFs Using the Android SDK
The Android SDK has had basic support for PDF files since API level 21 (Android 5.0). This API resides in a package, android.graphics.pdf
, and it supports basic low-level operations, such as creating PDF files and rendering pages to bitmaps. The Android SDK doesn’t provide a UI for interacting with PDF documents, so you’ll need to write your own UI to handle user interaction if you wish to use it as a basis for a PDF viewer in your app.
The main API entry point is PdfRenderer
, which provides an easy way to render single pages into bitmaps:
// Create the page renderer for the PDF document. val fileDescriptor = ParcelFileDescriptor.open(documentFile, ParcelFileDescriptor.MODE_READ_ONLY) val pdfRenderer = PdfRenderer(fileDescriptor) // Open the page. val page = pdfRenderer.openPage(pageNumber) // Render the page to the bitmap. val bitmap = Bitmap.createBitmap(page.width, page.height, Bitmap.Config.ARGB_8888) page.render(bitmap, null, null, PdfRenderer.Page.RENDER_MODE_FOR_DISPLAY) // Use the rendered bitmap. ... // Close the page when you're done with it. page.close() ... // Close the `PdfRenderer` when you're done with it. pdfRenderer.close()
You can now set these rendered bitmaps into an ImageView
or build your own UI that handles multi-page documents, scrolling, and zooming.
Now, we’ll introduce two libraries that support basic, ready-to-use UIs for displaying documents.
Displaying PDFs with MuPDF
One of the most widely known PDF libraries is MuPDF. It’s available either as a commercial solution or under an open source (AGPL) license.
MuPDF provides a small and fast viewer with a basic UI. Embedding it in your app is simple:
repositories { jcenter() maven { url 'http://maven.ghostscript.com' } ... } dependencies { compile 'com.artifex.mupdf:viewer:1.12.+' ... }
You can now open the MuPDF viewer activity by launching an intent with a document URI:
import com.artifex.mupdf.viewer.DocumentActivity; ... val intent = Intent(this, DocumentActivity::class.java) intent.action = Intent.ACTION_VIEW intent.data = Uri.fromFile(documentFile) startActivity(intent)
Using DocumentActivity
is enough for most basic use cases. MuPDF ships with its entire source code, so you can build any required functionality on top of it.
ℹ️ Note: MuPDF also supports other document formats on top of PDF, such as XPS, CBZ, EPUB, and FictionBook 2.
Rendering PDFs with the Android PdfViewer Library
Android PdfViewer is a library for displaying PDF documents. It has basic support for page layouts, zooming, and touch gestures. It’s available under the Apache License, Version 2.0 and, as such, is free to use, even in commercial apps.
Integration is simple. Start with adding a library dependency to your build.gradle
file:
compile 'com.github.barteksc:android-pdf-viewer:3.1.0-beta.1'
Then add the main PDFView
to the layout where you wish to view the PDFs:
... <com.github.barteksc.pdfviewer.PDFView android:id="@+id/pdfView" android:layout_width="match_parent" android:layout_height="match_parent"/> ...
Now you can load the document in your activity:
...
val pdfView = findViewById<PdfView>(R.id.pdfView)
pdfView.fromUri(documentUri).load()
...
PSPDFKit for Android
The above solutions are free or open source libraries with viewer capabilities. However, the PDF spec is fairly complex, and it defines many more features on top of the basic document viewing capabilities. We at PSPDFKit offer a comprehensive PDF solution for Android and other platforms, along with first-class support included with every plan. PSPDFKit comes with a fully featured document viewer with a modern customizable user interface and a range of additional features such as:
-
Annotation editing
-
Interactive forms with JavaScript support
-
Digital signatures
-
Indexed search
-
Document editing (both programmatically and through the UI)
-
Redaction
-
And much more
If you’re interested in our solution, visit the Android product page to learn more and download a free trial.
Conclusion
In this post, we outlined some of the available free PDF viewer libraries for Android that can help you render PDFs. However, if you want to add more advanced features to your PDF viewer — such as PDF annotation support, interactive PDF forms, and digital signatures — you should consider looking into commercial alternatives.
At PSPDFKit, we offer a commercial, feature-rich, and completely customizable Android PDF library that’s easy to integrate and comes with well-documented APIs to handle complex use cases. Try our PDF library using our free trial and check out our demos to see what’s possible.