How to Build an ASP.NET PDF Viewer with PSPDFKit
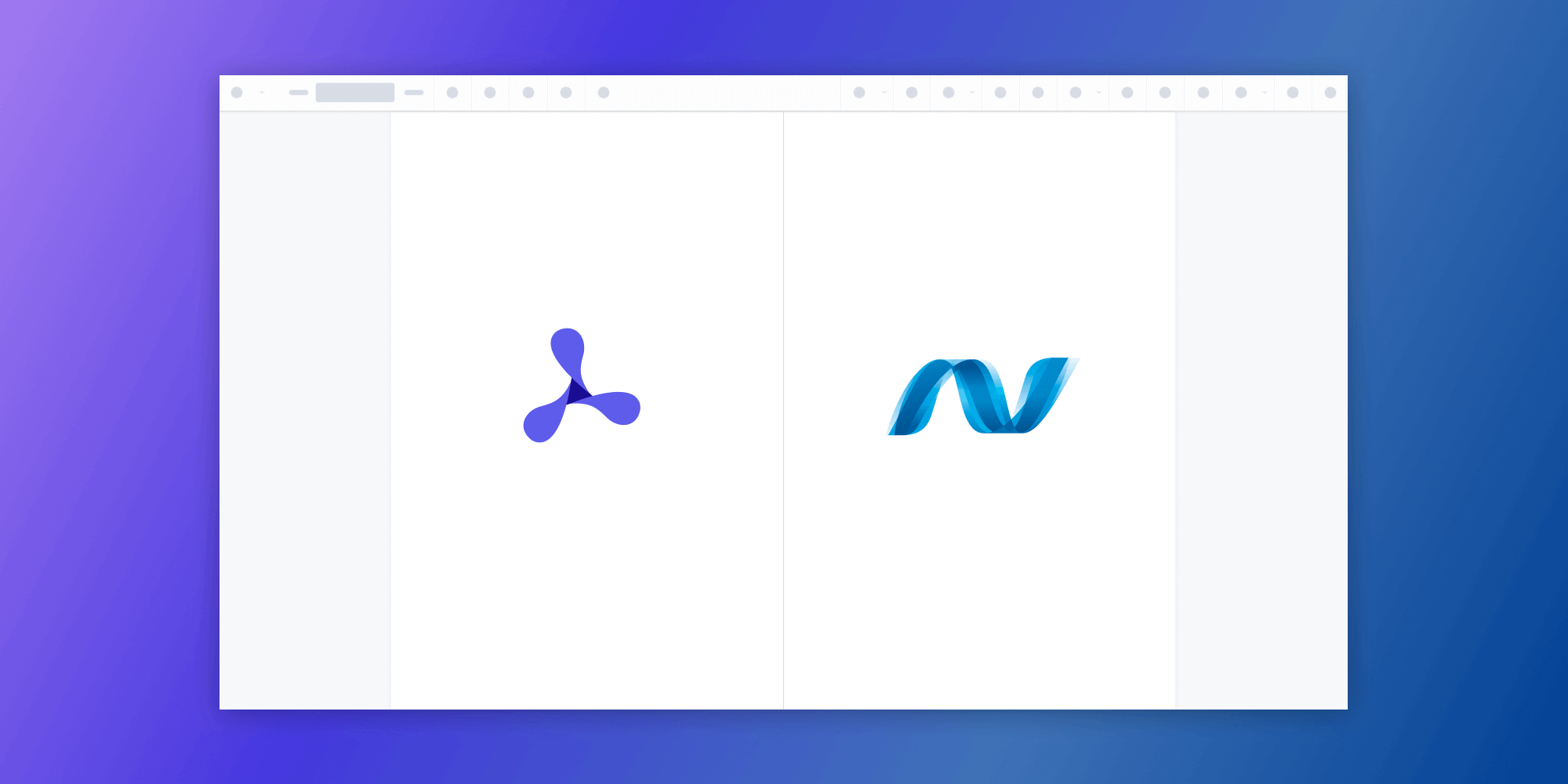
In this post, we provide you with a step-by-step guide outlining how to deploy PSPDFKit’s ASP.NET PDF viewer.
ASP.NET is an open source web framework used to build modern web apps and services with .NET, and it was created by Microsoft. ASP.NET Core is the open source version of ASP.NET, and it runs on macOS, Windows, Linux, and Docker.
ASP.NET Core MVC is a framework used to build web applications and APIs using the Model-View-Controller (MVC) design pattern, which will be the focus of this tutorial.
MVC is an architectural pattern that promotes separation of concerns. It allows you to separate your code into logical units and then build a web application that can easily be maintained and extended.
What Is an ASP.NET PDF Viewer?
An ASP.NET PDF viewer lets you render and view PDF documents in a web browser without the need to download it to your hard drive or use an external application like a PDF reader.
PSPDFKit ASP.NET PDF Viewer
We offer a commercial ASP.NET PDF viewer library that can easily be integrated into your web application. It comes with 30+ features that let you view, annotate, edit, and sign documents directly in your browser. Out of the box, it has a polished and flexible UI that you can extend or simplify based on your unique use case.
- A prebuilt and polished UI for an improved user experience
- 15+ prebuilt annotation tools to enable document collaboration
- Support for more file types with client-side PDF, MS Office, and image viewing
- Dedicated support from engineers to speed up integration
Example of Our ASP.NET PDF Viewer
To demo our ASP.NET PDF viewer, upload a PDF, JPG, PNG, or TIFF file by clicking Open Document under the Standalone option (if you don’t see this option, select Choose Example from the dropdown). Once your document is displayed in the viewer, try drawing freehand, adding a note, or applying a crop or an e-signature.
Requirements to Get Started
To get started, you’ll need:
-
.NET 6.0, which is the current Long Term Support (LTS) version of .NET.
You can download it from Microsoft’s website.
Creating a New ASP.NET Project
-
For this tutorial, you’ll install the ASP.NET web application (Model-View-Controller) template using the .NET CLI (command-line interface). Open your terminal, navigate to the directory where you want to create your project, and run the following command:
dotnet new mvc -o pspdfkit-aspnet-example
This command creates your ASP.NET MVC project and places it in a new directory called pspdfkit-aspnet-example
inside your current location.
-
Change your directory to the newly created project:
cd pspdfkit-aspnet-example
mvc
is a template that creates the initial files and directory structure for an ASP.NET MVC project. Here’s the file structure of the project:
-
Controllers
— Handles the request flow and delegate information between the Model and the View. The Controller will get the data information from the Model and then pass that data into the View. -
Models
— Handles data logic and represents how data is stored. -
Views
— Presents the user interface to the user. View templates are created using Razor. These templates have a.cshtml
extension. -
Views/Shared
— Contains the shared components and stylesheets. -
Properties/launchSettings.json
— Located in theProperties
folder and holds the development environment configuration. -
wwwroot
— Contains the public static assets. You’ll store the PDF file and PSPDFKit artifacts in this folder. -
Program.cs
— The entry point of the web application. -
pspdfkit-aspnet-example.csproj
— Defines the app project and its dependencies.
Adding PSPDFKit to Your Project
PSPDFKit for Web library files are distributed as an archive that can be extracted manually.
-
Download the framework here. The download will start immediately and will save a
.tar.gz
archive likePSPDFKit-Web-binary-2022.2.1.tar.gz
to your computer. -
Once the download is complete, extract the archive and copy the entire contents of its
dist
folder to your project’swwwroot/lib/
folder. -
Make sure your
wwwroot/lib/
folder contains thepspdfkit.js
file and apspdfkit-lib
directory with the library assets.
Displaying a PDF
-
Add the PDF document you want to display to the
wwwroot
directory. You can use our demo document as an example. -
Navigate to the
Views/Shared/_Layout.cshtml
file. You’ll only keep the@RenderBody()
placeholder in this file. This will help you display the contents of the view-specific pages in the@RenderBody()
placeholder:
<!DOCTYPE html> <html lang="en"> <head> <title>@ViewData["Title"] - pspdfkit_aspnet_example</title> @* Removed for brevity *@ </head> <body> @RenderBody() </body> </html>
-
Now, you’ll start working on the
Home
route. Navigate to your project’sViews/Home/Index.cshtml
Razor file.
-
Add an empty
<div>
element with a defined height to where PSPDFKit will be mounted:
<div id="pspdfkit" style="height: 100vh"></div>
-
Include
pspdfkit.js
. The path is relative to thewwwroot
directory:
<script src="/lib/pspdfkit.js"></script>
-
Load the PSPDFKit SDK before the
</body>
tag:
<script> PSPDFKit.load({ container: "#pspdfkit", document: "document.pdf", }) .then(function(instance) { console.log("PSPDFKit loaded", instance); }) .catch(function(error) { console.error(error.message); }); </script>
You can see the full code for the Views/Home/Index.cshtml
file here:
@{ ViewData["Title"] = "Home Page"; } <div id="pspdfkit" style="height: 100vh"></div> <script src="/lib/pspdfkit.js"></script> <script> PSPDFKit.load({ container: "#pspdfkit", document: "document.pdf", }) .then(function(instance) { console.log("PSPDFKit loaded", instance); }) .catch(function(error) { console.error(error.message); }); </script>
Serving the Application
-
Start the app in the root directory of your project:
dotnet watch run
The server will start and automatically restart the application when changes are made to the project.
![]()
You can access the source code for this tutorial on GitHub. If you're using Visual Studio, follow our getting started guide for Visual Studio.
Adding Even More Capabilities
Once you’ve deployed your viewer, you can start customizing it to meet your specific requirements or easily add more capabilities. To help you get started, here are some of our most popular ASP.NET guides:
- Adding annotations
- Editing documents
- Filling PDF forms
- Adding signatures to documents
- Real-time collaboration
- Redaction
- UI customization
Conclusion
You should now have our ASP.NET PDF viewer up and running in your web application. If you hit any snags, don’t hesitate to reach out to our Support team for help.
You can also deploy our vanilla JavaScript PDF viewer or use one of our many web framework deployment options like React.js, Vue.js, jQuery, and Angular.
To see a list of all web frameworks, start your free trial. Or, launch our demo to see our viewer in action.