How to Build a React.js PDF Viewer with react-pdf
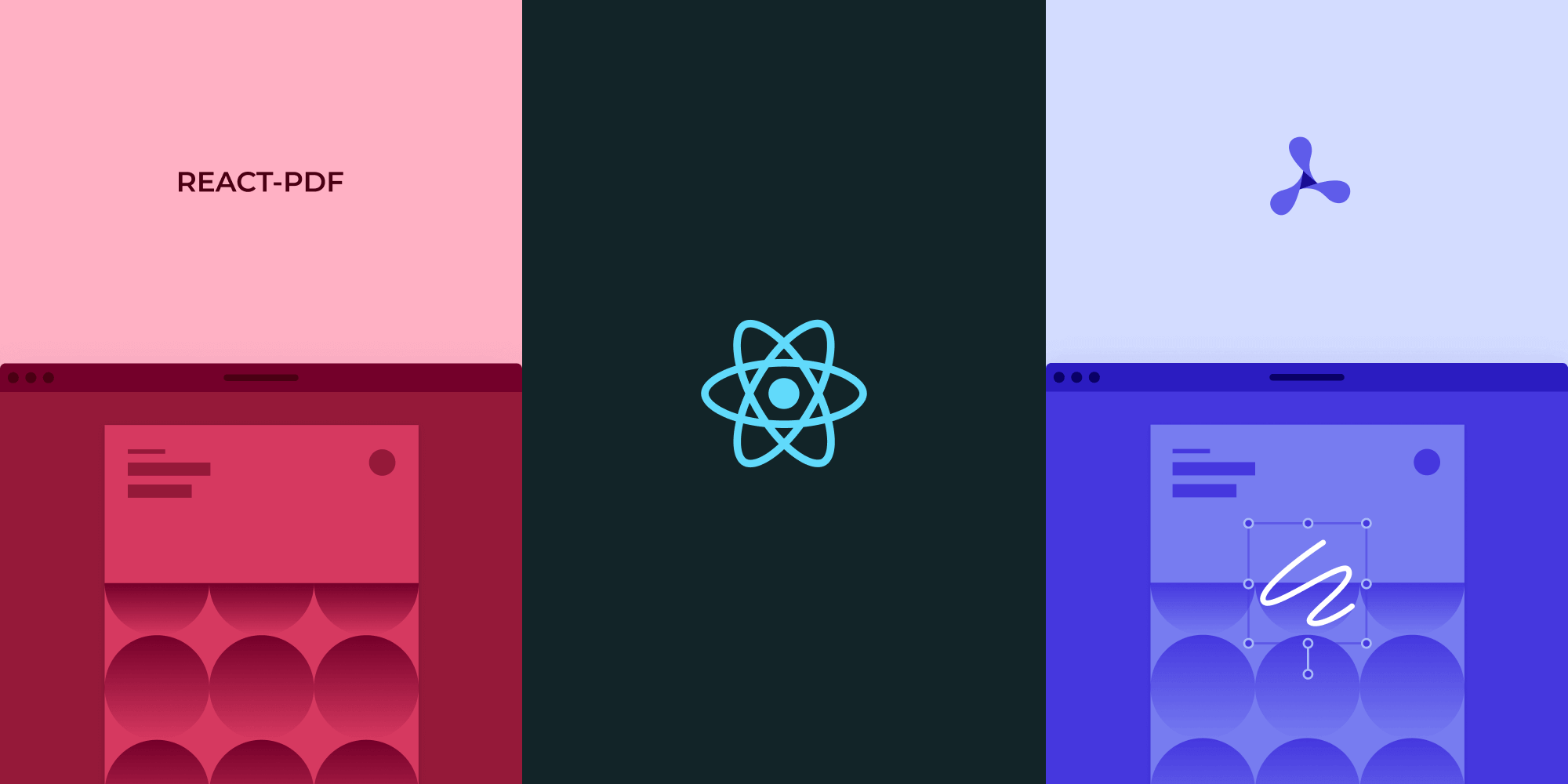
![]()
This article was first published in September 2021 and was updated in May 2024.
In this blog post, you’ll learn how to build a PDF viewer using React.js and the react-pdf
library. React.js is the leading JavaScript library for building user interfaces (UIs). It was created and is maintained by Meta, formerly Facebook. Meta, Instagram, Netflix, Slack, Airbnb, and many other companies use React.js, and it can be used to create a variety of different applications. In this blog post, you’ll use it to create a PDF viewer.
In the first part of this blog post, you’ll look at how to create the PDF viewer with an open source library, and in the second part, you’ll be able to follow along with a guide on how to integrate the PSPDFKit React.js PDF Viewer Library into a React.js project.
Open Source React.js PDF Viewer Libraries
There are a couple of open source React PDF viewer libraries. One of the most popular is the @react-pdf/renderer
library, with 510K weekly downloads on npm. It’s used for creating PDF files in the browser, on the server, or on mobile devices. If you want to work with this library, please refer to our How to Create a PDF with React.js blog post.
Another popular library is react-pdf
by Wojciech Maj, with around 860K weekly downloads on npm. It’s used to display existing PDFs. This library will be used in this blog for the purpose of creating a PDF viewer.
react-pdf
provides a React component API for opening PDF files and rendering them using PDF.js. PDF.js is an open source JavaScript library for rendering PDF files.
Here’s an overview of some of this library’s features:
- A prebuilt and polished UI for an improved user experience
- 15+ prebuilt annotation tools to enable document collaboration
- Support for more file types with client-side PDF, MS Office, and image viewing
- Dedicated support from engineers to speed up integration
Requirements to Get Started
To get started, you’ll need:
-
A package manager compatible with npm. This guide contains usage examples for Yarn and the npm client (installed with Node.js by default). Make sure the
npm
version is 5.6 or greater.
Building a React.js PDF Viewer with react-pdf
Start by creating a React.js project with vite
:
npm create vite@latest react-pdf-example -- --template react
After the project is created, change the directory into the project folder:
cd react-pdf-example
Adding react-pdf
-
Now, you can install the npm package for the
react-pdf
library from the terminal:
npm install react-pdf
-
Place the file you want to render inside the
public
directory of thereact-pdf-example
project. You can use our demo document as an example; you just need to rename it todocument.pdf
.
Displaying a PDF
-
react-pdf
comes with two components:Document
andPage
.Document
is used to open a PDF and is mandatory. Within the document, you can mount pages, which are used to render the PDF page. To integrate this into your example project, opensrc/App.js
and replace its contents with the following:
import { useState } from 'react'; import { Document, Page } from 'react-pdf'; import { pdfjs } from 'react-pdf'; // Text layer for React-PDF. import 'react-pdf/dist/Page/TextLayer.css'; // Importing the PDF.js worker. pdfjs.GlobalWorkerOptions.workerSrc = new URL( 'pdfjs-dist/build/pdf.worker.min.js', import.meta.url, ).toString(); const App = () => { const [numPages, setNumPages] = useState(null); const [pageNumber, setPageNumber] = useState(1); const onDocumentLoadSuccess = ({ numPages }) => { setNumPages(numPages); }; const goToPrevPage = () => setPageNumber(pageNumber - 1 <= 1 ? 1 : pageNumber - 1); const goToNextPage = () => setPageNumber( pageNumber + 1 >= numPages ? numPages : pageNumber + 1, ); return ( <div> <nav> <button onClick={goToPrevPage}>Prev</button> <button onClick={goToNextPage}>Next</button> <p> Page {pageNumber} of {numPages} </p> </nav> <Document file="document.pdf" onLoadSuccess={onDocumentLoadSuccess} > <Page pageNumber={pageNumber} /> </Document> </div> ); }; export default App;
For react-pdf
to work, a PDF.js worker needs to be provided. You can do this in a couple of ways. For most cases, importing the worker will work as was done in the src/App.js
file:
import { pdfjs } from 'react-pdf'; pdfjs.GlobalWorkerOptions.workerSrc = new URL( 'pdfjs-dist/build/pdf.worker.min.js', import.meta.url, ).toString(); // Rest of code.
You can read more about how to configure PDF.js worker in your project here.
-
Now, start the application by running the following:
npm run dev
One of the disadvantages of using react-pdf
is that it doesn’t come with a UI. In this example, you’ve rendered two buttons to navigate between pages and showed the total number of pages.
![]()
You can access the full code on GitHub.
Overall, react-pdf
is a great open source project, but it has some disadvantages:
- It doesn’t come with a user interface out of the box. If you need a UI to help users navigate through a PDF, you’ll need to build it from scratch.
- Text selection doesn’t work properly. If you try to select text in a PDF, you’ll see that it’s not a good user experience.
Building a React.js PDF Viewer with PSPDFKit
We offer a commercial React.js PDF library that can easily be integrated into your web application. It comes with 30+ features that let you view, annotate, edit, and sign documents directly in your browser. Out of the box, it has a polished and flexible UI that you can extend or simplify based on your unique use case.
- A prebuilt and polished UI
- 15+ annotation tools
- Support for multiple file types
- Dedicated support from engineers
Creating a New React Project
-
Use
vite
to scaffold out a simple React application:
npx create vite@latest pspdfkit-react-example -- --template react
-
Change to the created project directory:
cd pspdfkit-react-example
Adding PSPDFKit to Your Project
-
Now, add PSPDFKit for Web as a dependency:
npm install pspdfkit
-
As you’re using the WebAssembly build of PSPDFKit for Web, the final setup step is to copy files the browser will need from the
npm
module:
cp -R ./node_modules/pspdfkit/dist/pspdfkit-lib public/pspdfkit-lib
The code above will copy the pspdfkit-lib
directory from within node_modules/
into the public/
directory to make it available to the SDK at runtime.
-
Make sure your
public
directory contains apspdfkit-lib
directory with the PSPDFKit library assets.
Displaying a PDF
-
Add the PDF document you want to display to the public directory. You can use our demo document as an example.
-
Add a component wrapper for the PSPDFKit library and save it as
components/ViewerComponent.jsx
:
import { useEffect, useRef } from 'react'; export default function ViewerComponent(props) { const containerRef = useRef(null); useEffect(() => { const container = containerRef.current; let PSPDFKit; (async function () { PSPDFKit = await import('pspdfkit'); PSPDFKit.unload(container); await PSPDFKit.load({ // Container where PSPDFKit should be mounted. container, // Use dark theme. theme: PSPDFKit.Theme.DARK, // The document to open. document: props.document, // Use the public directory URL as a base URL. PSPDFKit will download its library assets from here. baseUrl: `${window.location.protocol}//${ window.location.host }/${import.meta.env.BASE_URL}`, }); })(); return () => PSPDFKit && PSPDFKit.unload(container); }, [props.document]); return ( <div ref={containerRef} style={{ width: '100%', height: '100vh' }} /> ); }
To load PSPDFKit for Web into the ViewerComponent
, use PSPDFKit.load
when the component has mounted.
-
Now, all that’s left is to render the
ViewerComponent
inApp.jsx
by loading the PDF you downloaded earlier:
import ViewerComponent from './components/ViewerComponent'; function App() { return ( <div className="App" style={{ width: '100vw' }}> <div className="App-viewer"> <ViewerComponent document={'document.pdf'} /> </div> </div> ); } export default App;
-
You can also open a different PDF file by adding a button and adding a handler to the
onClick
event:
// App.jsx import { useState } from 'react'; import ViewerComponent from './components/ViewerComponent'; function App() { const [document, setDocument] = useState('document.pdf'); const handleOpen = () => setDocument('another-example.pdf'); return ( <div className="App"> <button className="App-button" onClick={handleOpen}> Open another document </button> <div className="App-viewer"> <ViewerComponent document={document} /> </div> </div> ); } export default App;
You can use this example document as a second example. Rename it to another-example.pdf
, and then add it to the /public
folder.
When you click Open another document, it loads the another-example.pdf
file.
-
Your project structure will now look like this:
pspdfkit-react-example ├── public │ ├── pspdfkit-lib │ └── document.pdf │ └── another-example.pdf ├── src │ ├── components │ | └── ViewerComponent.jsx | └── App.js └── package.json
-
Start the app and run it in your default browser:
npm run dev
You’ll now see your PDF rendered in the container
element. Try using the toolbar to navigate through, annotate, and search in the document. Note that because PSPDFKit is a commercial product, you’ll see a PSPDFKit for Web Evaluation notice on the document. To get a license key, contact Sales.
![]()
You can find the finished code on GitHub.
If you hit any snags, don’t hesitate to reach out to our Support Team for help.
Adding Even More Capabilities
Once you’ve deployed your viewer, you can start customizing it to meet your specific requirements or easily add more capabilities. To help you get started, here are some of our most popular React.js guides:
- Adding annotations
- Editing documents
- Filling PDF forms
- Adding signatures to documents
- Real-time collaboration
- Redaction
- UI customization
Conclusion
In this post, you first saw how to build a React.js PDF viewer with the react-pdf
library. In the second half, you walked through how to deploy the PSPDFKit React.js PDF viewer.
You can also deploy our vanilla JavaScript PDF viewer or use one of our many web framework deployment options like Vue.js, Angular, and jQuery. To see a list of all web frameworks, start your free trial. Or, launch our demo to see our viewer in action.