PDF Form Field Date and Time Picker
PSPDFKit supports a user’s ability to easily input dates and times into form fields through a browser’s native picker UI, without the need to manually type them in.
Any form field configured to format its content using the AFDate_Format
, AFDate_FormatEx
, AFTime_Format
, or AFTime_FormatEx
function will automatically show a picker icon if supported by the user’s browser.
If the browser doesn’t support it, then the form field will present the user with placeholder text so they can see the expected format.
Picker Types
PSPDFKit supports three types of pickers, which will be automatically selected based on the expected format of the field.
Date
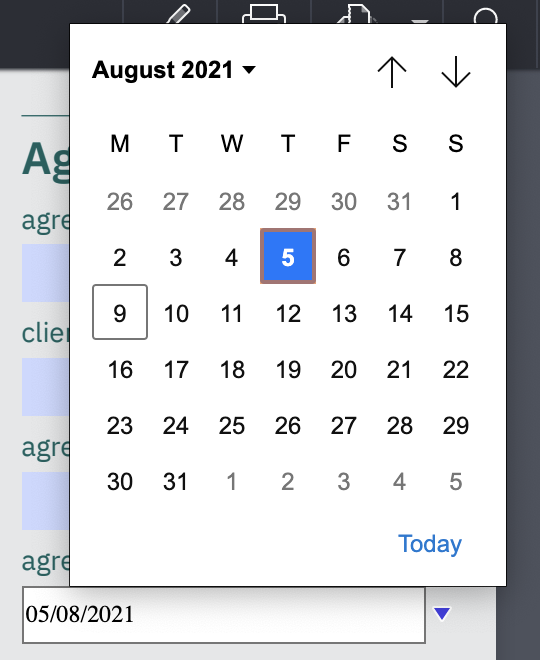
Time
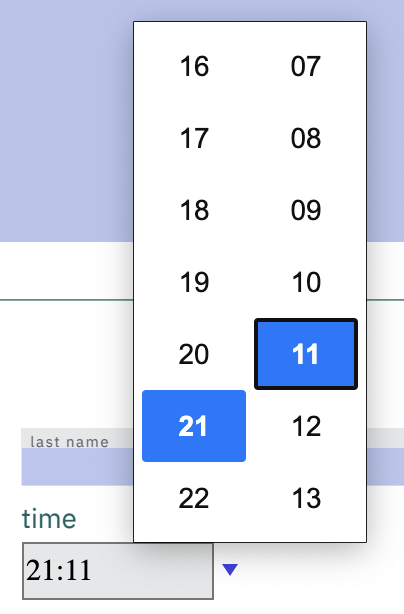
Date and Time

The support from each browser will determine which of these pickers can be used.
Browser Support
Chrome, Edge, and mobile browsers have full support for this feature.
Firefox and desktop Safari will only show the picker if the form field expects a date only.
IE 11 and Legacy Edge don’t support this feature and will only show the placeholder text.
![]()
Internet Explorer 11 is no longer supported in our Web SDK as of version 2022.5. Edge 18 is no longer supported in our Web SDK as of version 2023.2.
Enabling the Picker with PSPDFKit
To use the picker with your own form fields, attach a formatting action, which calls any of the following methods, to the form’s widget:
Below is an example where we create a field that accepts a date in the dd/mm/yyyy
format:
const widget = new PSPDFKit.Annotations.WidgetAnnotation({ id: PSPDFKit.generateInstantId(), pageIndex: 0, formFieldName: "date", boundingBox: new PSPDFKit.Geometry.Rect({ left: 50, top: 75, width: 150, height: 60, }), additionalActions: { onFormat: new PSPDFKit.Actions.JavaScriptAction({ script: 'AFDate_FormatEx("mm/dd/yy")', }), }, }); const formField = new PSPDFKit.FormFields.TextFormField({ name: "date", annotationIds: PSPDFKit.Immutable.List([widget.id]), }); instance.create([widget, formField]);
Make sure when formatting for a date to use the AFDate
function, and when formatting for a time, use the AFTime
function. This is important, because using the wrong function will result in the picker not displaying. Additionally, validation will fail, thereby preventing the user from filling the form.
Styling and Internationalization
Since the picker is handled by the browser, there’s currently no way to customize the style or the internationalization.
JavaScript and Server Support
PSPDFKit doesn’t need JavaScript enabled for the PDF to show the picker. However, if you want to validate the input, you’ll need to enable JavaScript support on PSPDFKit for Web Standalone.
The picker will also work for PSPDFKit for Web Server-Backed, but it won’t be able to validate the input.