How to Convert DOCX to WebP Using Python
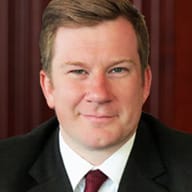
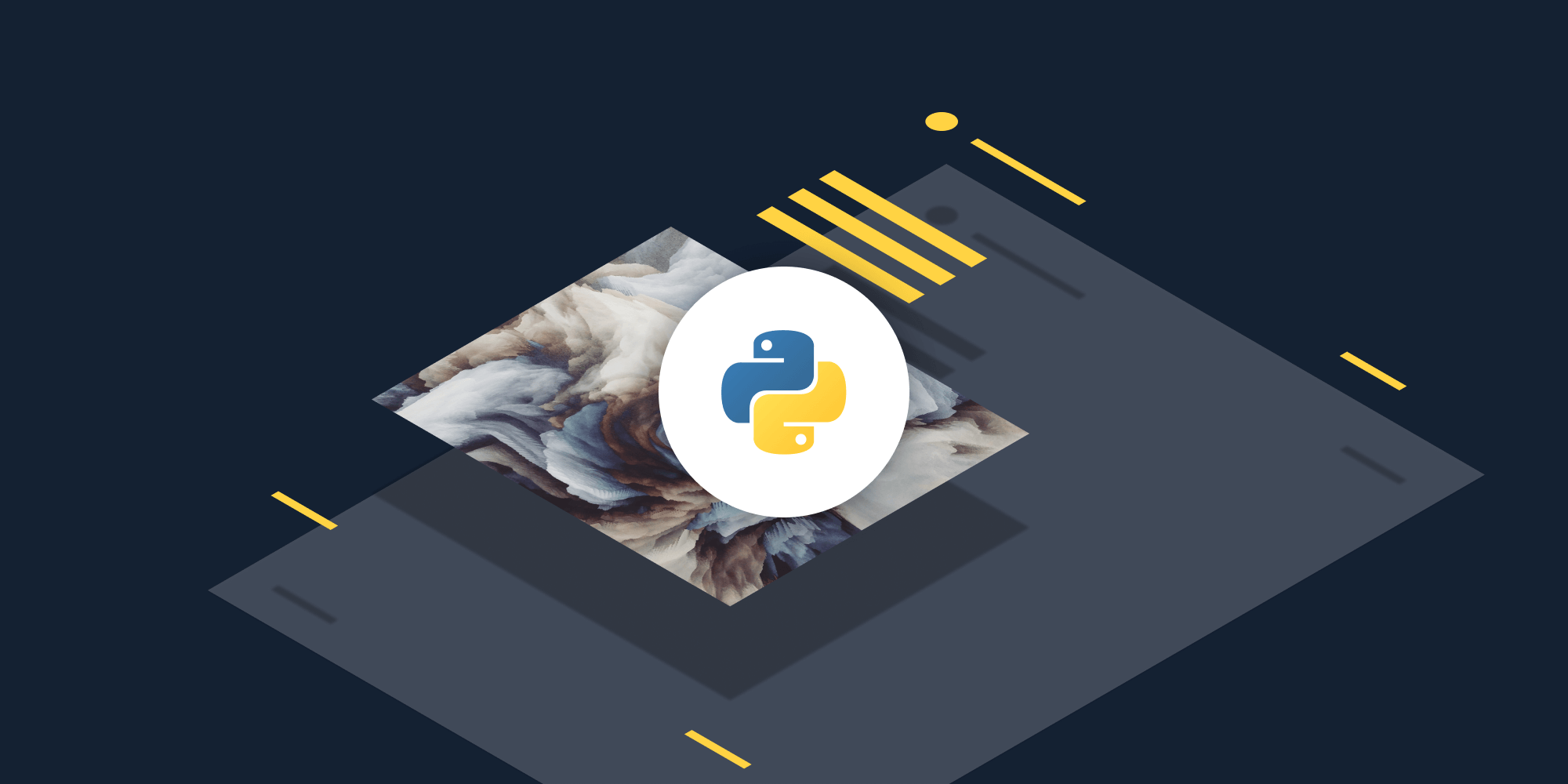
In this post, you’ll learn how to convert DOCX files to WebP in your Python application using PSPDFKit’s DOCX to WebP API. With our API, you receive 100 credits with the free plan. Different operations on a document consume different amounts of credits, so the number of PDF documents you can generate may vary. All you need to do is create a free account to get access to your API key.
PSPDFKit API
PSPDFKit offers 30+ PDF API tools that you can combine to create complex document processing workflows. You’ll be able to generate PDFs or convert various file formats into PDFs and then:
-
Merge, split, delete, flatten, and duplicate PDF documents.
-
Watermark PDFs with both text and images.
-
Carry out complex operations such as signing, OCRing, and extracting tables from PDFs.
Once you create your account, you’ll be able to access all our PDF API tools.
Step 1 — Creating a Free Account on PSPDFKit
Go to our website, where you’ll see the page below, prompting you to create your free account.
After creating your account, navigate to Pricing and Plans to see an overview of your current plan.
As you can see in the bottom-left corner, you’ll start with 100 credits, and you’ll be able to access all our PDF API tools.
Step 2 — Obtaining the API Key
After you’ve verified your email, you can get your API key from the dashboard. In the menu on the left, click API Keys. You’ll see the following page, which is an overview of your keys.
Copy the Live API Key, because you’ll need this for the DOCX-to-WebP conversion.
Step 3 — Setting Up Your Files and Folders
Now, create a folder called docx_to_webp
and open it in a code editor. You’ll use VS Code as your primary code editor for this tutorial. Next, create two folders inside docx_to_webp
and name them input_documents
and processed_documents
.
Then, in the root folder, docx_to_webp
, create a file called processor.py
. This is the file where you’ll keep your code.
Now, put the DOCX file in the input_documents
folder. You can use our demo document as an example.
Your folder structure will look like this:
docx_to_webp ├── input_documents ├── processed_documents └── processor.py
Step 4 — Writing the Code
Open the processor.py
file and paste in the code below:
import requests import json instructions = { 'parts': [ { 'file': 'document' } ], 'output': { 'type': 'image', 'format': 'webp', 'dpi': 500 } } response = requests.request( 'POST', 'https://api.pspdfkit.com/build', headers = { 'Authorization': 'Bearer pdf_live_Uir6WHjDeFDf8jrUY43Hv2NNJt0VKkV7LQkSLZT3J7S' }, files = { 'document': open('input_documents/document.docx', 'rb') }, data = { 'instructions': json.dumps(instructions) }, stream = True ) if response.ok: with open('processed_documents/image.webp', 'wb') as fd: for chunk in response.iter_content(chunk_size=8096): fd.write(chunk) else: print(response.text) exit()
Code Explanation
In the code above, you first import the requests
and json
dependencies. After that, you create the instructions for the API call.
You then use the requests
module to make the API call, and if the response is successful, the result is stored in the processed_documents
folder.
Output
To execute the code, use the command below:
python3 processor.py
Once the code has been successfully executed, you’ll see a new file in the processed_documents
folder called image.webp
:
docx_to_webp ├── input_documents ├── processed_documents | └── image.webp └── processor.py
Final Words
In this post, you learned how to seamlessly integrate our DOCX to WebP API into your Python application.
In addition to document conversion, you can integrate multiple API tools into your existing applications and perform other operations, such as merging several documents into a single PDF, adding watermarks, and more. To get started with a free trial, sign up here.