How to Convert HTML to PDF Using wkhtmltopdf and C#
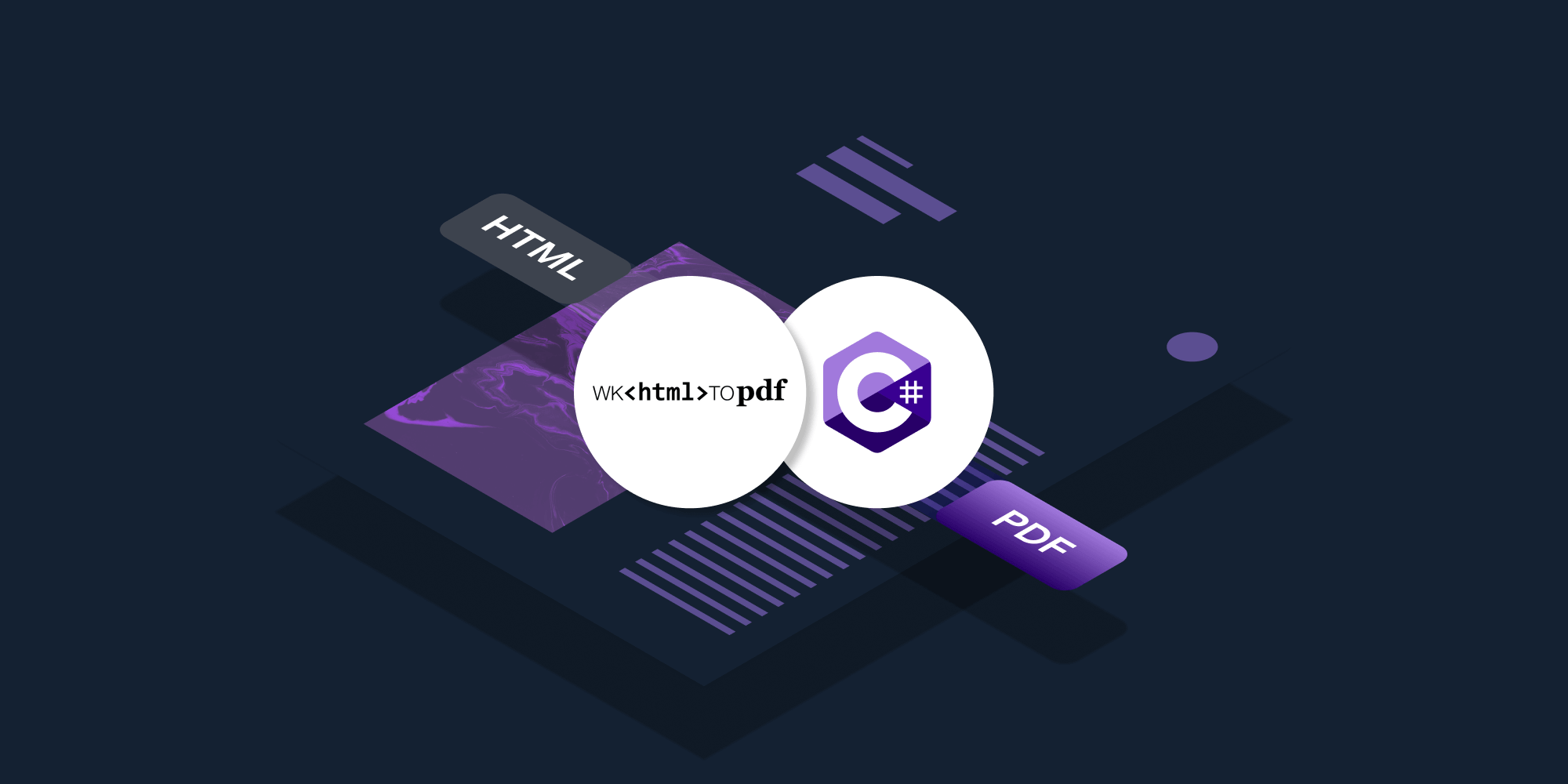
In this blog post, we’ll go over the steps necessary to convert HTML to PDF using wkhtmltopdf and C#. wkhtmltopdf is an open source command-line tool for converting HTML to PDF using the WebKit rendering engine. It’s a powerful tool that can be used to generate high-quality PDFs with various options for styling and formatting. In this tutorial, we’ll cover how to install wkhtmltopdf, how to set up a C# project, and how to integrate it with wkhtmltopdf to convert HTML to PDF.
Development Considerations
Before getting started, it’s important to note that wkhtmltopdf is a command-line tool, and it doesn’t have a built-in graphical user interface (GUI). It’s also an open source tool, created in 2008 by Jakob Truelsen, and it isn’t being consistently maintained at this time.
If you’re considering a commercial solution, PSPDFKit offers some options for you:
-
HTML to PDF in C# API — A REST API/hosted solution that gives you 100 free conversions per month and offers additional packages for a per-document fee.
-
HTML to PDF in C# — Our self-hosted solution backed by our GdPicture.NET library.
Our solutions are regularly maintained, with releases occurring multiple times throughout the year. We also offer one-on-one support to handle any issues or challenges that you encounter.
Requirements
-
Visual Studio Code installed on your machine
-
The C# extension for Visual Studio Code
Installing wkhtmltopdf
To install wkhtmltopdf, follow the steps for your machine below.
On Windows
-
Download the wkhtmltopdf binary from the official website.
-
Once the download is complete, extract the files to a directory of your choice.
-
Add the directory containing the wkhtmltopdf binary to your system’s
PATH
environment variable. This will allow you to run the wkhtmltopdf command from anywhere on your system. -
Verify that wkhtmltopdf is installed correctly by running the following command in your terminal:
where wkhtmltopdf
The wkhtmltopdf executable will be located in the installation directory — by default, it’s C:\Program Files\wkhtmltopdf\bin
.
On macOS
-
You can install wkhtmltopdf using Homebrew:
brew install --cask wkhtmltopdf
-
Or, you can download the binary from the official website.
If you choose to download the binary from the official website, you may see an error message that says the following:
“wkhtmltox-0.12.6-2.macos-cocoa.pkg” cannot be opened because it is from an unidentified developer.
This means your Mac’s security settings are preventing you from installing the package.
To install the package, you’ll need to change your Mac’s security settings to allow installation of packages from unidentified developers. Follow the steps below:
-
Right-click the
wkhtmltox-0.12.6-2.macos-cocoa.pkg
file and select Open. -
A warning message will appear. Click the Open button.
-
The package installer will open. Follow the prompts to complete the installation.
-
Verify that wkhtmltopdf is installed correctly by running the following command in your terminal:
which wkhtmltopdf
The wkhtmltopdf executable will be located in the installation directory — by default, it’s /usr/local/bin
.
On Ubuntu
If you’re using Ubuntu, you can install wkhtmltopdf by running the following command:
sudo apt-get install wkhtmltopdf
Setting Up a .NET Console Project in Visual Studio Code
Once it’s installed, follow the steps below to set up a project.
-
Create a new directory for your project and open it in Visual Studio Code.
-
Open the terminal (press Control or Command + backtick `).
-
Run the following command to create a new .NET console project:
dotnet new console --framework net7.0
-
Replace the contents of
Program.cs
with the following code:
class Program { static void Main(string[] args) { Console.WriteLine("Hello, World!"); } }
Integrating wkhtmltopdf with C#
-
Add the following namespace to the top of your
Program.cs
file:
using System.Diagnostics
The System.Diagnostics
namespace provides classes and methods that allow you to interact with system processes, event logs, and performance counters.
-
In your Main method, create a new instance of the
ProcessStartInfo
class and set theFileName
property to the path of the wkhtmltopdf binary:
var processStartInfo = new ProcessStartInfo { // Pass the path of the wkhtmltopdf executable. FileName = "/usr/local/bin/wkhtmltopdf", };
You can search for the executable in the file explorer. By default, it’s located in C:\Program Files\wkhtmltopdf\bin
on Windows and in /usr/local/bin
on Mac.
If you’ve installed wkhtmltopdf using a package manager like apt-get
, yum
, or brew
, you can check the path using these commands:
-
On Linux, type
whereis wkhtmltopdf
-
On macOS, type
brew info wkhtmltopdf
Another solution is to use the full path of the executable. You can find the full path of the wkhtmltopdf executable by running the following command in your terminal:
find / -name wkhtmltopdf 2>/dev/null
This will search for the executable in the entire file system and print its location.
Once you’ve found the path to the wkhtmltopdf executable, you can use it in the ProcessStartInfo
class to run the process.
-
Set the
Arguments
property to include the input HTML file and the output PDF file, in that order:
var inputHtml = "/Users/<username>/Desktop/<your-project-name>/bin/Debug/net7.0/input.html"; var outputPdf = "output.pdf"; var processStartInfo = new ProcessStartInfo { FileName = "/usr/local/bin/wkhtmltopdf", Arguments = $"{inputHtml} {outputPdf}", };
The input.html
file must be located in the same directory as the wkhtmltopdf executable. Make sure to use an absolute path to specify the location of the input HTML file and the output PDF file.
For example, you created the input.html
file in the /Users/<username>/Desktop/<your-project-name>/bin/Debug/net7.0/input.html
directory.
-
Set the
UseShellExecute
property tofalse
andRedirectStandardOutput
totrue
:
var processStartInfo = new ProcessStartInfo { // pass the path of the wkhtmltopdf executable FileName = "/usr/local/bin/wkhtmltopdf", Arguments = $"{inputHtml} {outputPdf}", UseShellExecute = false, RedirectStandardOutput = true, WorkingDirectory = AppDomain.CurrentDomain.BaseDirectory };
UseShellExecute = false
allows the process to be started without creating a new window, and RedirectStandardOutput = true
enables the output of the process to be read.
The WorkingDirectory
property is used to set the current directory of the process.
AppDomain.CurrentDomain.BaseDirectory
is used to set the current directory as the base directory of the application’s domain. This is necessary to ensure that the input and output files are located in the correct directory within the project.
-
Start the process using the
Process.Start
method, and wait for it to complete using theWaitForExit
method:
using (var process = Process.Start(processStartInfo)) { process?.WaitForExit(); if (process?.ExitCode == 0) { Console.WriteLine("HTML to PDF conversion successful!"); } else { Console.WriteLine("HTML to PDF conversion failed!"); Console.WriteLine(process?.StandardOutput.ReadToEnd()); } }
Once the process exits, check the ExitCode
property to see if the conversion was successful. If the ExitCode
is 0
, it means the conversion was successful, and a success message is printed. If the ExitCode
isn’t 0
, it means the conversion failed and a failure message is printed, along with the standard output of the process, using the StandardOutput.ReadToEnd()
method.
Find the complete code below:
// Program.cs // See https://aka.ms/new-console-template for more information. using System.Diagnostics; class Program { static void Main(string[] args) { var inputHtml = "/Users/<username>/Desktop/<your-project-name>/bin/Debug/net7.0/input.html"; var outputPdf = "output.pdf"; if (!System.IO.File.Exists(inputHtml)) { Console.WriteLine($"{inputHtml} file not found!"); return; } var processStartInfo = new ProcessStartInfo { // Pass the path of the wkhtmltopdf executable. FileName = "/usr/local/bin/wkhtmltopdf", Arguments = $"{inputHtml} {outputPdf}", UseShellExecute = false, RedirectStandardOutput = true, WorkingDirectory = AppDomain.CurrentDomain.BaseDirectory }; Console.WriteLine($"Starting process with FileName: {processStartInfo.FileName} and Arguments: {processStartInfo.Arguments}"); using (var process = Process.Start(processStartInfo)) { process?.WaitForExit(); if (process?.ExitCode == 0) { Console.WriteLine("HTML to PDF conversion successful!"); } else { Console.WriteLine("HTML to PDF conversion failed!"); Console.WriteLine(process?.StandardOutput.ReadToEnd()); } } } }
Running the Project
Run the project using the dotnet run
command in the terminal.
Conclusion
In this tutorial, you learned how to convert HTML to PDF using wkhtmltopdf and C#. With this tool, you can easily convert HTML files to high-quality PDFs with various options for styling and formatting.
It’s important to note that wkhtmltopdf is a command-line tool and it doesn’t have a built-in GUI. However, it can be integrated with other tools or libraries, such as web browsers, .NET libraries, and Python libraries to provide a graphical interface for users.
If you’re looking to add more robust PDF capabilities, PSPDFKit offers commercial options that can easily be integrated into your application:
Get started with our API product for free, or integrate a free trial of our .NET PDF library.