Conditional Compilation for Apple’s Yearly Updates
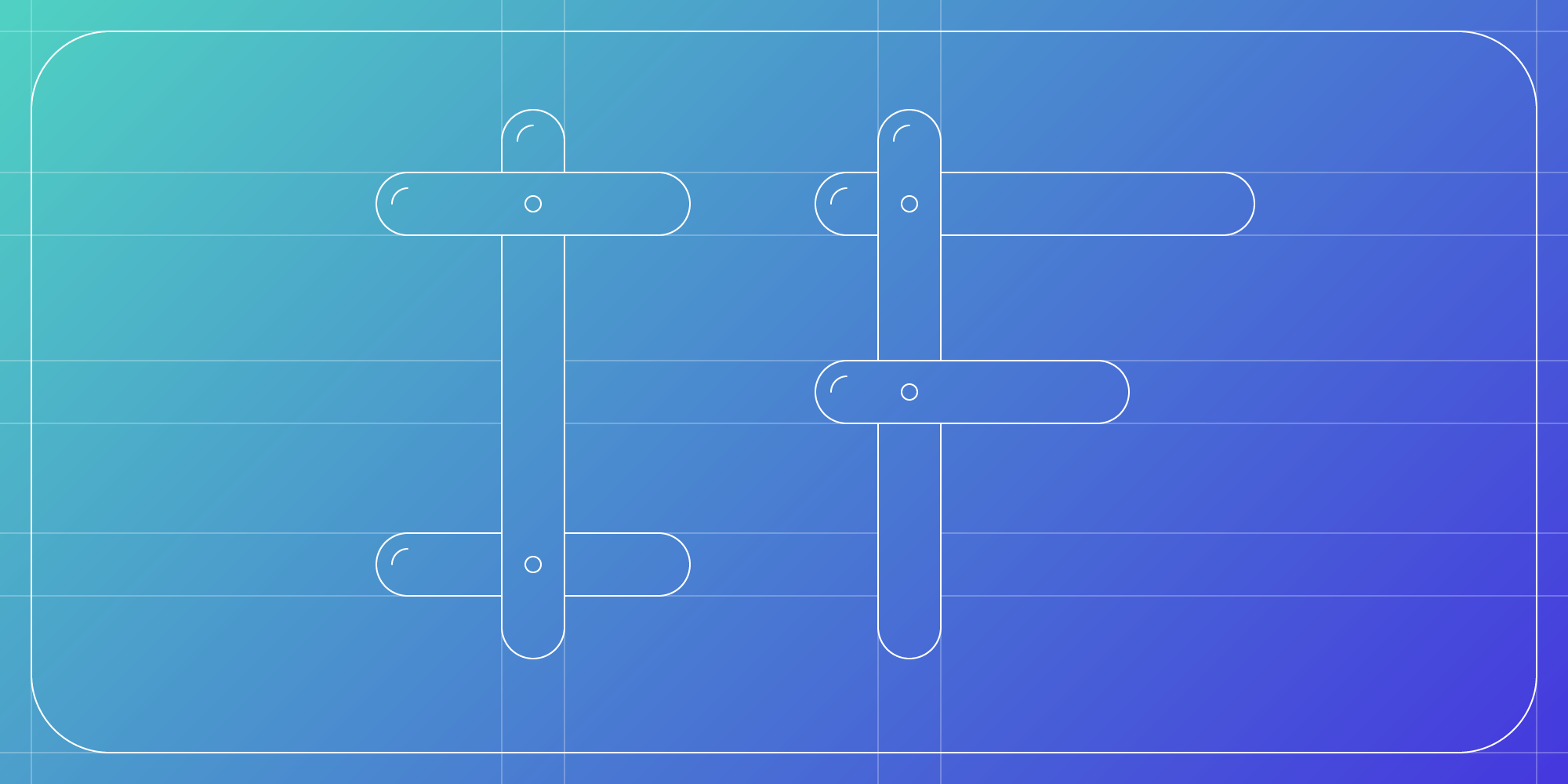
Over the last several years, Apple has settled into a consistent cycle with updates to its platforms. The company won’t publicly commit to future plans, but as a software developer on Apple platforms, it’s important to know what to expect to plan your work. Apple doesn’t release all its yearly operating system updates at the same time, so some special considerations are needed for cross-platform apps — which is what this article will explore.
This post comes from our perspective of having a codebase using UIKit and SwiftUI with the iOS SDK and the Mac Catalyst SDK. While I won’t mention the macOS SDK (for AppKit and SwiftUI), the issues and solution outlined in this post are also applicable there. If your codebase only supports one platform, then the situation is a little simpler for you.
Expectations of Apple
This is what we can expect, based on Apple’s pattern from the previous few years:
-
In June, Apple is expected to release the first betas of iOS 17, macOS 14 and Xcode 15, which will use the iOS 17 and Mac Catalyst 17 SDKs. Meanwhile, Xcode 14 will remain the stable release of Xcode, which uses the iOS 16 and Mac Catalyst 16 SDKs.
-
In September, Apple is expected to release the public version of iOS 17 and Xcode 15.0, which will continue using the iOS 17 SDK. However, crucially, Apple won’t release the public version of macOS 14, and correspondingly, Xcode 15.0 will revert to using the Mac Catalyst 16 SDK.
-
In October, Apple is expected to release the public version of macOS 14 and Xcode 15.1, which will bring back using the Mac Catalyst 17 SDK.
This table summarises what happened last year:
iOS SDK | Mac Catalyst SDK | Swift Version | |
---|---|---|---|
Xcode 13.4 | 15.5 | 15.5 | 5.6 |
Xcode 14.0 beta | 16.0 | 16.0 | 5.7 |
Xcode 14.0 RC | 16.0 | 15.5 | 5.7 |
Xcode 14.1 | 16.0 | 16.0 | 5.7 |
The Problem
If we started using APIs that were added in the new iOS SDK when the beta version of Xcode was available, we’d get a nasty surprise when the final .0
version of Xcode came out: Our code would stop compiling for Mac. We’d also no longer be able compile with the old version of Xcode, due to new APIs being missing from the old SDK.
Between June and October, we want to achieve the following:
-
We want to use new APIs introduced in the iOS 17 SDK to benefit from new iOS 17 features. We want to start testing these before the public release of iOS 17.
-
We want our codebase to continue to compile and run with the older version of Xcode so that we can reproduce issues reported in production and ship updates.
-
We don’t want to require all our personnel to update Xcode at precisely the same time. This includes not just our iOS team, but also our Core team, Hybrids team, Support team and more.
-
We don’t want the overhead of managing different branches in our version control system for different versions of Xcode.
The Solution
To use new APIs, we need two different types of checks:
-
A compile-time check that the APIs are available in the SDK our code is being linked with.
-
A runtime check that the APIs are available in the version of the OS our code is running on.
Compile-Time Check
The compile-time check is the trickier part, so let’s look at that first. Swift’s conditional compilation blocks support these conditions:
-
os
— Target operating system platform -
arch
— CPU architecture -
swift
— Swift language version -
compiler
— Swift compiler version -
canImport
— Whether a framework/module is available -
targetEnvironment
— Whether the target is a simulator or is Mac Catalyst
There’s no check for the version of the SDK that the code is being linked with.
We considered if we could use the Swift version. This would work if iOS is your only platform, but it doesn’t work for the Mac where the SDK version is reverted in the final .0
Xcode release but the Swift version isn’t.
Since each new version of Apple’s SDKs tends to include several new frameworks (modules), we can use the workaround of checking whether one of these modules is available. Last year, in 2022, we picked RoomPlan. It’s incredibly cool tech, but we don’t actually use or plan to use RoomPlan anywhere in our codebase, which will make it easy to search for when we can remove these checks later on:
#if canImport(RoomPlan) // We're linking with the new version of the SDK, but we might be running on either a new or old version of the OS. #else // We're linking with the old version of the SDK. #endif
If we compiled using the old version of Xcode or the final .0
new version on Mac, RoomPlan wouldn’t be available, so only the code between #else
and #endif
would be compiled. In other scenarios, our code would be linked with the new SDK, meaning RoomPlan would be available, so the first block of code would be complied.
Runtime Check
To check the version of the OS our code is running on, we use a Swift availability condition like this:
if #available(iOS 16.0, *) { // Use new APIs. } else { // New APIs aren't available. }
Putting These Together
Putting these two checks together, we ended up with this code for last year’s updates (in 2022):
#if canImport(RoomPlan) if #available(iOS 16.0, *) { // Use new APIs. } else { // Provide fallback behavior when compiling with the newer Xcode but running on an older version of the OS. } #else // Provide fallback behavior when compiling with an Xcode version using the old SDK. #endif
In most cases, the fallback code will be identical. Unfortunately, there’s no way to structure this to avoid repeating a small amount of code.
Here’s a trivial example showing using the preferredMenuElementOrder
property of UIButton
that was added in the iOS 16 SDK. We use this new API, if it’s available, to ask the menu to show its actions with the first action at the top, even if the menu appears upward from the button. On older versions, we can implement this behaviour by manually reversing the order of our menu actions:
var actions: [UIAction] = ... let button = UIButton() button.showsMenuAsPrimaryAction = true #if canImport(RoomPlan) // iOS 16.0 SDK if #available(iOS 16.0, *) { button.preferredMenuElementOrder = .fixed } else { actions.reverse() } #else actions.reverse() #endif button.menu = UIMenu(children: actions)
With this, all our goals are met, and we could even leave these checks in our codebase indefinitely. Once all our personnel update to the .1
of the new Xcode version, the #else
block will simply never be compiled, so we can clean this up at a time that suits us.
What about Objective-C?
These checks are more obvious in Objective-C because we can directly check the version of the SDK being linked with:
#if __IPHONE_16_0 if (@available(iOS 16.0, *)) { // Use new APIs. } else { // Provide fallback behavior when compiling with the newer Xcode but running on an older version of the OS. } #else // Provide fallback behavior when compiling with an Xcode version using the old SDK. #endif
Conclusion
In this post, we’ve seen the problem of supporting new features in Apple’s OS updates while still having our code compile with the stable or older version of Xcode. We need both a compile-time check and a runtime check. To make the compile-time check in Swift, we use the canImport
condition with an arbitrary module that was added in the newer SDK version. This allows everyone on the team to use whichever version of Xcode they need for a particular task. That way, there’s no need to worry about version control conflicts or working on the wrong branch.