PSPDFKit 3.4 for Flutter Adds PDF Generation
PSPDFKit 3.4 for Flutter has shipped! We’re pleased to announce that PDF Generation is now supported in our Flutter PDF Library. With this new component, you can programmatically create new PDF documents from images, templates, and HTML.
Generate PDFs from Images
Generate PDF files from images by creating a NewPage
object from a PdfImagePage
. Then, pass the image uri
and image position
as creation parameters for the PdfImagePage
:
// Image file to generate the PDF from. File img = File('<readable-image-path>'); // File path where the generated PDF will be saved. String outputPath = '<writable-output-file-path>'; // A list of PDF pages from the image URI. List<NewPage> pages = [ NewPage.fromImage( PdfImagePage.fromUri(img.uri, PagePosition.center)), ]; // Generate a PDF from an image and save it at `[outputPath]`. String? generatedFilePath = await PspdfkitProcessor.instance.generatePdf( pages, outputPath);
Generate PDFs from Templates
There are two ways to generate a PDF from a template.
-
From a
PagePattern
object. PSPDFKit provides a list of predefined page patterns you can choose from, or you can use a custom tiled PDF document, as shown in the code snippet below:
// Custom tiled PDF document. File patternTilesDocument = File('<readable-tiled-document-path'); // Output location to save the generated PDF. String outputPath = '<writable-file-path>'; List<NewPage> pages = [ // PSPDFKit predefined patterns. NewPage.fromPattern(PagePattern.blank), NewPage.fromPattern(PagePattern.grid5mm), NewPage.fromPattern(PagePattern.line5mm), NewPage.fromPattern(PagePattern.dots5mm), // Page from a custom tiled PDF document. NewPage.fromPattern( PagePattern.fromDocument(patternTilesDocument.uri, 0)), ]; // Generate a PDF from a page template and save it at `[outputPath]`. String? generatedFilePath = await PspdfkitProcessor.instance.generatePdf(pages, outputPath);
-
From an existing PDF document page represented by the
PdfPage
class. This can be a PDF page with form fields or any other desired PDF page contents. Generate a PDF from another PDF document page withNewPage.fromPdfPage
, passing aPdfPage
instance created with the source document URI and the page index, as demonstrated in the code snippet below:
// Source document file. File sourceDocument = File('readabl-output-path'); // Output location to save the generated PDF. String outputPath = '<writable-output-path>'; List<NewPage> pages = [ // New page from an existing document page. NewPage.fromPdfPage( PdfPage( sourceDocumentUri: sourceDocument.uri, pageIndex: 4, )) ]; String? generatedFilePath = await PspdfkitProcessor.instance.generatePdf(pages, outputPath);
Generate PDFs from HTML
PSPDFKit utilizes the full power of WebKit on iOS and WebView on Android to convert HTML to PDF. The HTML can be from a string, a local file URI, or even a remote URL.
-
From an HTML string:
// HTML string. const html = ''' <html> <head> <style type="text/css"> h1 { color: red; } </style> </head> <body> <h1>Hello, world!</h1> </body> </html> '''; // Writable output path. final outputFilePath = '<writable-output-file-path>' // Generate the PDF from the HTML string. String? generatedPdf = await PspdfkitProcessor.instance .generatePdfFromHtmlString(html, outputFilePath); if (generatedPdf == null) { print('Failed to generate PDF from HTML string.'); return; } // Display the generated PDF. await Pspdfkit.present(generatedPdf);
-
From an HTML URI:
// HTML Uri from URL. final htmlUri = Uri.parse('https://pspdfkit.com'); // Output path for the generated PDF. final outputFilePath = <writable-output-path>; // Generate the PDF from the HTML URL. String? generatedPdf = await PspdfkitProcessor.instance .generatePdfFromHtmlUri(htmlUri, outputFilePath); if (generatedPdf == null) { print('Failed to generate PDF from HTML URI.'); return; } // Display the generated PDF. await Pspdfkit.present(generatedPdf);
Flutter 3.3 Compatibility
PSPDFKit 3.4 for Flutter also addresses the breaking changes from the Flutter SDK 3.3 update. This means that if you’re upgrading to Flutter 3.3 and above, you should also upgrade to PSPDFKit 3.4 for Flutter.
Check out our changelog for a full list of these changes.
If you’re interested in PSPDFKit for Flutter, please contact our Sales team. For a complete list of features, please visit our product page. We’d love to hear about your use case and discuss how to best implement it.
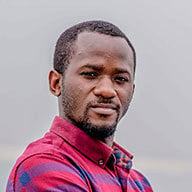
Julius joined PSPDFKit in 2021 as an Android engineer and is now the Cross-Platform Team Lead. He has a passion for exploring new technologies and solving everyday problems. His daily tools are Dart and Flutter, which he finds fascinating. Outside of work, he enjoys movies, running, and weightlifting.