How to Generate PDF Certificates from HTML in Python
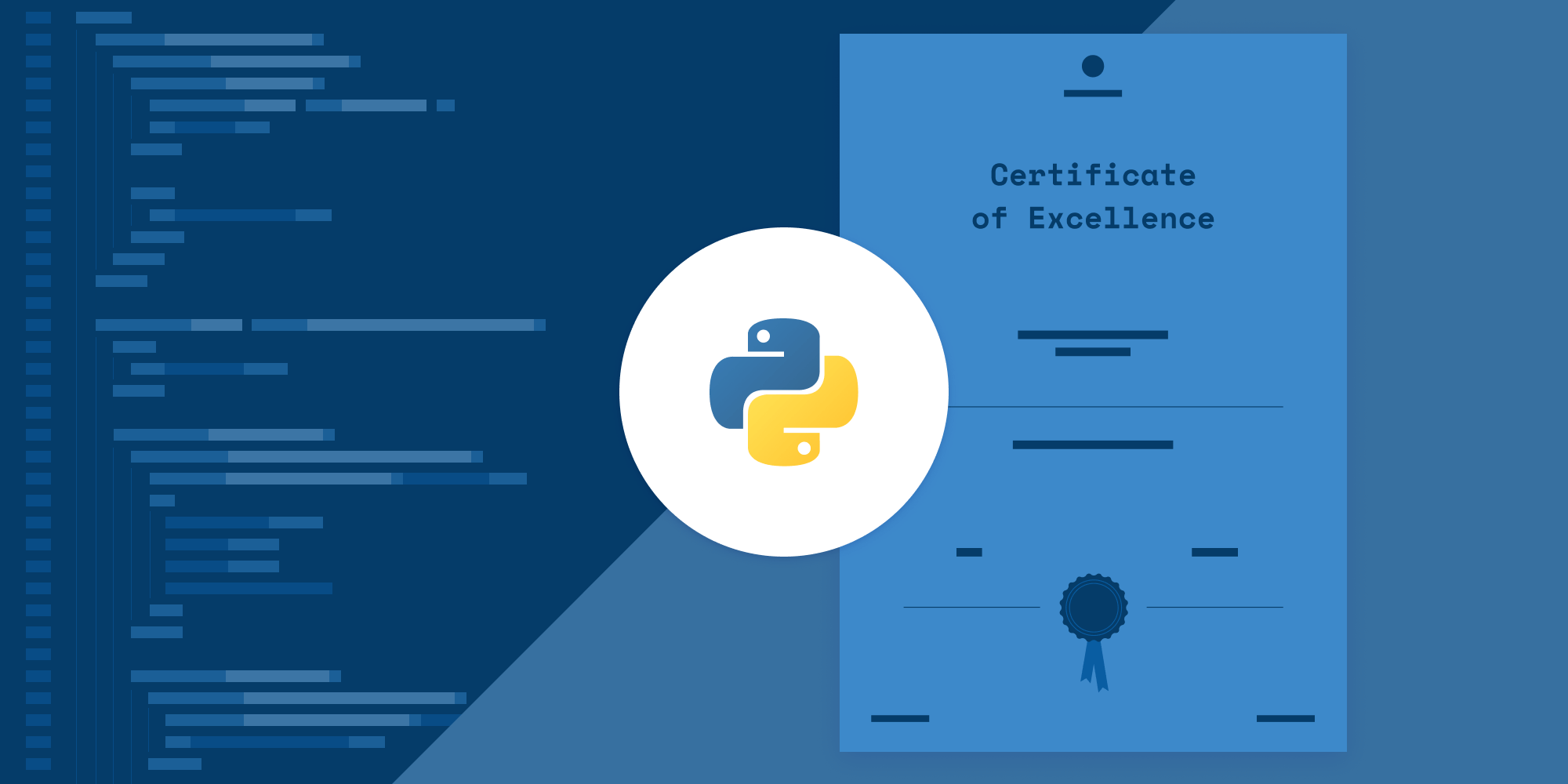
In this post, you’ll learn how to generate PDF certificates from HTML using our Python PDF Generator API. With our API, you can generate 100 PDF certificates per month for free. To access your API key, sign up for a free account.
Certificates are most commonly generated by educational institutions, online course platforms, and corporate training programs. PDF certificates can automatically be generated when a student or employee successfully completes their program. To further streamline the process, a student’s name, program, and completion date can be pulled from a database and dynamically inserted into the certificate.
To help you get started, we’ve provided a free certificate template in HTML and CSS that can be customized to meet your specific requirements. You can easily style your certificate by updating the CSS file with your own custom images and fonts.
Requirements
To get started, you’ll need:
To access your PSPDFKit API key, sign up for a free account. Your account lets you generate 100 documents for free every month. Once you’ve signed up, you can find your API key in the Dashboard > API Keys section.
Python is a programming language, and pip is a package manager for Python, which you’ll use to install the requests
library. Requests is an HTTP library that makes it easy to make HTTP requests.
Install the requests
library with the following command:
python -m pip install requests
Setup
Download the certificate template and extract the contents of the ZIP file into a folder. You’ll get an HTML file, Inter fonts, a Space Mono font, SVG logos, and a README file.
Creating a CSS File
If you open the index.html
file, you’ll see the styles between the opening and closing <style>
tag. Copy the styles to a new file called style.css
, and save it in the same folder:
@font-face { font-family: 'Inter'; src: url('Inter-Regular.ttf') format('truetype'); font-weight: 400; font-style: normal; } @font-face { font-family: 'Inter'; src: url('Inter-Medium.ttf') format('truetype'); font-weight: 500; font-style: normal; } @font-face { font-family: 'Inter'; src: url('Inter-Bold.ttf') format('truetype'); font-weight: 700; font-style: normal; } @font-face { font-family: 'Space Mono'; src: url('SpaceMono-Regular.ttf') format('truetype'); font-weight: 400; font-style: normal; } body { font-size: 0.875rem; font-family: 'Inter', sans-serif; font-weight: 400; color: #000000; text-align: center; margin: 0 auto; position: relative; } #pspdfkit-header { font-size: 0.625rem; text-transform: uppercase; letter-spacing: 2px; font-weight: 700; color: #717885; margin-top: 2.5rem; margin-bottom: 2.5rem; } .logo { margin-top: 2.5rem; height: 1.5rem; width: auto; } h1 { font-family: 'Space Mono', monospace; font-size: 2.25rem; font-weight: 400; margin-top: 0; } h4 { font-family: 'Space Mono', monospace; font-size: 1rem; font-weight: 400; } .page { margin-left: 5rem; margin-right: 5rem; } .form { display: flex; flex-direction: column; margin-top: 6rem; } .border-bottom { border: 1px solid #000000; border-top: none; border-left: none; border-right: none; } .signer { display: flex; justify-content: space-between; align-items: center; margin: 2rem 0 2rem 0; } .signer-item { flex-grow: 1; } input { color: #4537de; font-family: 'Space Mono', monospace; font-size: 1rem; text-align: center; margin-top: 4rem; height: 4rem; width: 100%; box-sizing: border-box; } input#name { font-size: 1.5rem; } #pspdfkit-footer { font-size: 0.5rem; text-transform: uppercase; letter-spacing: 1px; font-weight: 500; color: #717885; margin-top: 2.5rem; bottom: 2.5rem; position: absolute; width: 100%; } .footer-columns { display: flex; justify-content: space-between; padding-left: 2.5rem; padding-right: 2.5rem; }
To access the styles from index.html
, use the <link>
tag. While referring to the stylesheet file, just use the name of the file and don’t create nested paths:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="IE=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Certificate</title> <link rel="stylesheet" href="style.css" /> </head> </html>
Interacting with the API
Now, import requests
and json
to the pspdfkit.py
file. Place the pspdfkit.py
file in the root directory of your project:
import requests import json
Preparing the Payload
Create a dictionary to hold the data you want to send to the API. However, later you’ll need to convert the dictionary to a JSON object using the json.dumps
function.
The instructions
object references files by the name of their parts that are appended in the form data as files:
instructions = { 'parts': [ { 'html': 'index.html', 'assets': [ "style.css", "Inter-Regular.ttf", "Inter-Medium.ttf", "Inter-Bold.ttf", "SpaceMono-Regular.ttf", "logo.svg", "ribbon.svg", ], } ] }
To access the requests
library, use the request
method.
Now, make a POST
request to the https://api.pspdfkit.com/build
endpoint with the instructions
object as the payload. Then, save the resulting PDF as result.pdf
in the same folder as the Python file.
Don’t forget to replace YOUR_API_KEY
with your API key:
response = requests.request( 'POST', 'https://api.pspdfkit.com/build', headers={ 'Authorization': 'Bearer {YOUR_API_KEY}', # Replace with your API key. }, files={ 'index.html': open('index.html', 'rb'), 'style.css': open('style.css', 'rb'), 'Inter-Regular.ttf': open('Inter-Regular.ttf', 'rb'), 'Inter-Medium.ttf': open('Inter-Medium.ttf', 'rb'), 'Inter-Bold.ttf': open('Inter-Bold.ttf', 'rb'), 'SpaceMono-Regular.ttf': open('SpaceMono-Regular.ttf', 'rb'), 'logo.svg': open('logo.svg', 'rb'), 'ribbon.svg': open('ribbon.svg', 'rb'), }, data={ 'instructions': json.dumps(instructions) }, stream=True ) if response.ok: with open('result.pdf', 'wb') as fd: for chunk in response.iter_content(chunk_size=8096): fd.write(chunk) else: print(response.text) exit()
Generating the PDF
Now, run the Python application by executing the following command:
python3 pspdfkit.py
# Or for Python 2
python pspdfkit.py
You can see the full code below:
import requests import json instructions = { 'parts': [ { 'html': 'index.html', 'assets': [ "style.css", "Inter-Regular.ttf", "Inter-Medium.ttf", "Inter-Bold.ttf", "SpaceMono-Regular.ttf", "logo.svg", "ribbon.svg", ], } ] } response = requests.request( 'POST', 'https://api.pspdfkit.com/build', headers={ 'Authorization': 'Bearer {YOUR_API_KEY}' # Replace with your API key. }, files={ 'index.html': open('index.html', 'rb'), 'style.css': open('style.css', 'rb'), 'Inter-Regular.ttf': open('Inter-Regular.ttf', 'rb'), 'Inter-Medium.ttf': open('Inter-Medium.ttf', 'rb'), 'Inter-Bold.ttf': open('Inter-Bold.ttf', 'rb'), 'SpaceMono-Regular.ttf': open('SpaceMono-Regular.ttf', 'rb'), 'logo.svg': open('logo.svg', 'rb'), 'ribbon.svg': open('ribbon.svg', 'rb'), }, data={ 'instructions': json.dumps(instructions) }, stream=True ) if response.ok: with open('result.pdf', 'wb') as fd: for chunk in response.iter_content(chunk_size=8096): fd.write(chunk) else: print(response.text) exit()
Conclusion
In this post, you generated a PDF certificate from an HTML template using our Python PDF generation API. We created similar PDF certificate generation blog posts using sample code from other programming languages:
In addition to templates for generating certificates, we created free templates for other commonly used documents, like receipts, invoices, and reports. If you’re interested in generating other types of documents in Python, check out the following posts:
- Generating PDF receipts using Python
- Generating PDF invoices using Python
- Generating PDF reports using Python
All our templates are available for you to download on our PDF Generator API page. Feel free to customize or add any CSS to the template to fit your use case or help reflect your company’s brand.