Creating and Filling Out Forms Programmatically on Android
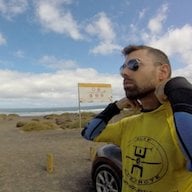
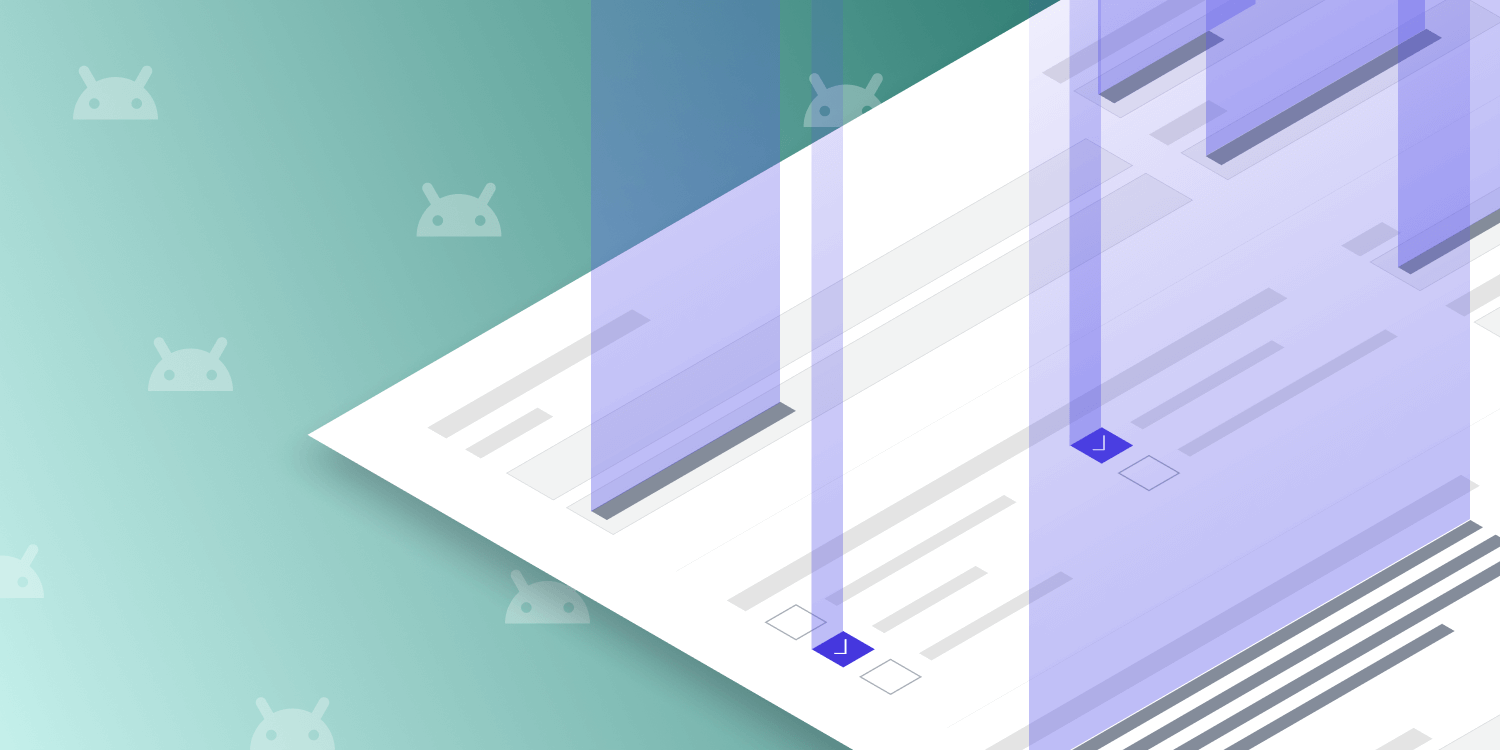
Embedding forms into a PDF can be helpful for people who need to collect data in documents, and there are many different types of form fields that can be used, as defined by the PDF specification. They cover a wide range of standards and inputs — from simple checkboxes and text fields, to more complex lists and signature fields.
Since PSPDFKit for Android 4.4, we’ve offered support for programmatic form field creation in a document. So in this post, we’ll delve into the APIs exposed by PSPDFKit for Android for creating and filling out forms programmatically.
Creating Forms
This example showcases how to add a text field to a document.
The first step for creating a new form is to implement a custom activity that subclasses the PdfActivity
.
The minimum amount of information required for the creation of a text form configuration is the page index and the annotation bounding box that will contain the signature form element; adding text is optional. Once built, it can be added to a document using FormProvider#addFormElementToPage
:
override fun onDocumentLoaded(document: PdfDocument) { super.onDocumentLoaded(document) ... val rectF = RectF(30f, 750f, 200f, 720f) val textFormConfiguration = TextFormConfiguration.Builder(0, rectF) .setText("Alphanumeric Text 1234") // Setting the text field as required will draw a red border around the field. .setRequired(true) .build() document.formProvider?.addFormElementToPage("textfield-1", textFormConfiguration)
@UiThread @Override public void onDocumentLoaded(@NonNull final PdfDocument document) { super.onDocumentLoaded(document); ... RectF rectF = new RectF(30f, 750f, 200f, 720f); TextFormConfiguration textFormConfiguration = new TextFormConfiguration.Builder(0, rectF) .setText("Alphanumeric Text 1234") // Setting the text field as required will draw a red border around the field. .setRequired(true) .build(); document.getFormProvider().addFormElementToPage("textfield-1", textFormConfiguration);
The code above shows how to use the addFormElementToPage
method for adding a new text form field once the document is loaded.
For more information on the difference between a form field and a form element, please see the introduction to forms guide.
You can add any kind of form field to a document, apart from signature form fields. Check out the Android documentation for more information about the Forms API.
If you want to see more examples of how to create form fields, please see FormCreationExample.kt
and FormCreationExample.java
in our Catalog app.
Filling Out Forms
PSPDFKit for Android supports the AcroForm standard, and the API can be easily accessed from PdfFragment
and PdfActivity
.
For example, you can fill out forms programmatically:
val document: PdfDocument = ... // This code shouldn't run on the main thread. Alternatively, use `getFormFieldsAsync()` to do this // processing in the RxJava chain. val formFields = document.formProvider.formFields formFields.filter { field -> field.type == FormType.TEXT } .forEach { field -> (field.formElement as TextFormElement).setText("Test ${field.name}") } formFields.filter { field -> field.type == FormType.CHECKBOX } .forEach { field -> (field.formElement as CheckBoxFormElement).toggleSelection() }
PdfDocument document = ... // This code shouldn't run on main thread. Alternatively, use `getFormFieldsAsync()` to do this // processing in the RxJava chain. List<FormField> formFields = document.getFormProvider().getFormFields(); for (FormField formField : formFields) { if (formField.getType() == FormType.TEXT) { TextFormElement textFormElement = (TextFormElement) formField.getFormElement(); textFormElement.setText("Test " + textFormElement.getName()); } else if (formField.getType() == FormType.CHECKBOX) { CheckBoxFormElement checkBoxFormElement = (CheckBoxFormElement)formField.getFormElement(); checkBoxFormElement.toggleSelection(); } }
The code example above shows how to fill out forms programmatically, applying different actions based on the field type.
If you want to see more examples of how to fill out form fields, please see FormFillingExample.kt
and FormFillingExample.java
in our Catalog app.
Conclusion
In this post, you saw how to create and fill out a form programmatically. These features can help with streamlining verbose operations for data gathering and distribution. Using the portability and flexibility of PDFs allows document creators to publish documents that can be supported on many different platforms and still behave in the same way.