Generate PDF Reports Using JavaScript
With PSPDFKit, you can use templates to create a PDF report in a user’s browser and without a server. This is useful in business intelligence or where you want to generate a PDF representation of data on a website.
With a combination of features like the Document Editor and annotations, PSPDFKit can create professional reports based on a template and include custom cover and final pages.
Setting Up the Pages
First, set up the report’s pages. You can do this with a template document from which you only preserve the first and last pages to use as the cover and final pages.
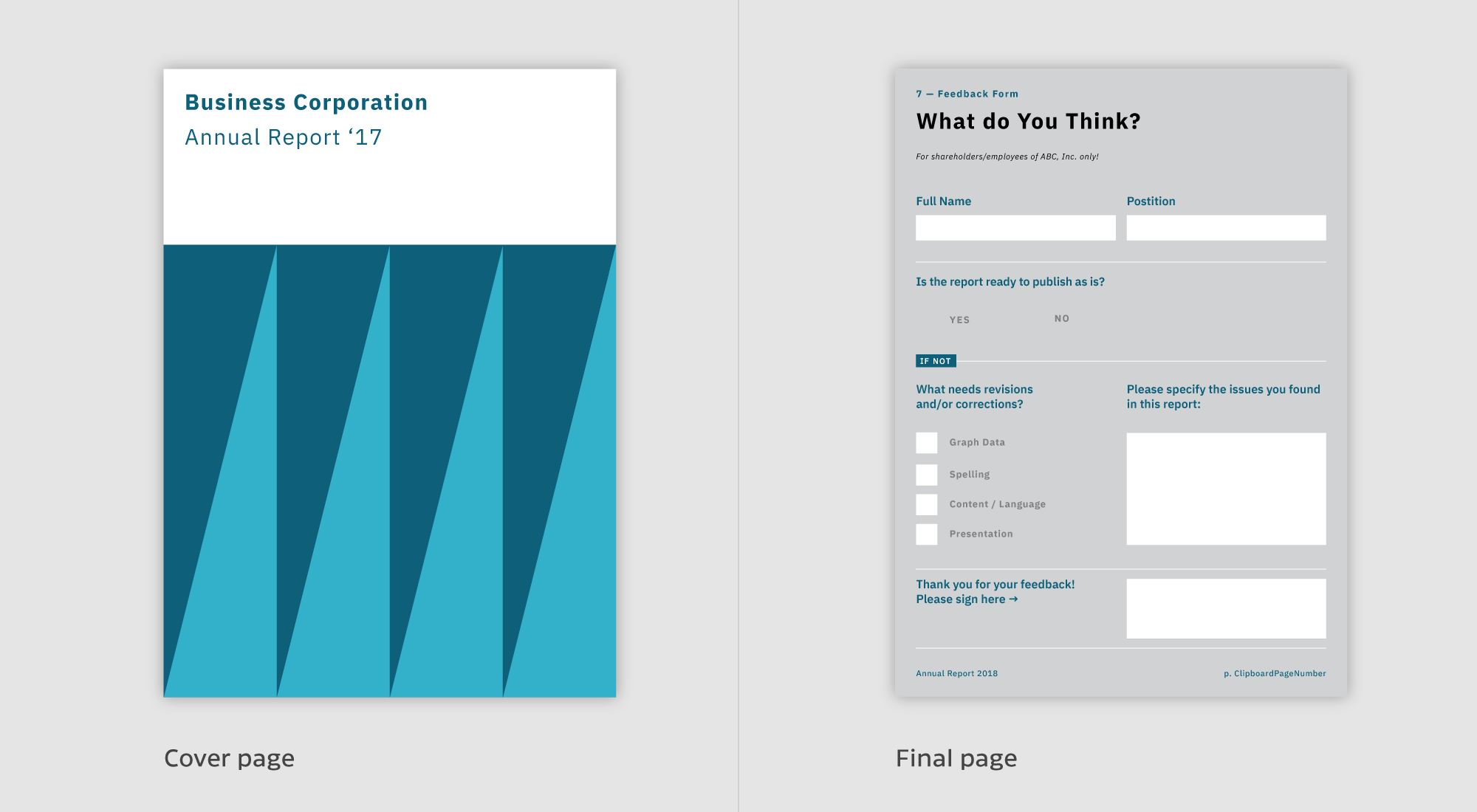
Then, add a new empty page with a pattern, and to finish, insert a page from another document. This is how it looks in code:
const instance = await PSPDFKit.load({ headless: true, document: document, }) // Keep only the first and last page of the original document. let pageIndexesToRemove = [...Array(instance.totalPageCount).keys()]; pageIndexesToRemove.shift() let exportedPDFArrayBuffer = await instance.exportPDFWithOperations([ { type: "removePages", pageIndexes: pageIndexesToRemove }, // Add a new page with a pattern grid as the third page of the report. { type: "addPage", afterPageIndex: 2, backgroundColor: PSPDFKit.Color.WHITE, pageWidth: 500, pageHeight: 750, rotateBy: 0, }, // Add a page from an existing document. { type: "importDocument", document: otherDocument, importedPageIndexes: [7] } })
Adding Text and Images
To add text and images, use text and stamp annotations, like so:
// Create a free text annotation. const freeTextAnnotation = new PSPDFKit.Annotations.TextAnnotation({ boundingBox: new PSPDFKit.Geometry.Rect({ left: 228, top: 924, width: 600, height: 80 }), fontSize: 40, text: { format: "plain", value: "Welcome to\nPSPDFKit", }, pageIndex: 0 }) // Create an image stamp annotation. const imageStampAnnotation = new PSPDFKit.Annotations.StampAnnotation({ stampType: 'Custom' title: 'Example Stamp', subtitle: 'Example Stamp Annotation', boundingBox: new PSPDFKit.Geometry.Rect({ left: 60, top: 400, width: 600, height: 80 }), pageIndex: 0 }) // Add the annotations to the document. await instance.create([freeTextAnnotation, imageStampAnnotation]);
See our guide on programmatically creating annotations for more details.
Adding Watermarks
Next, draw a “Generated for John Doe. Page X” watermark on every page, like this:
PSPDFKit.load({ container: "#pspdfkit", document: "document.pdf", renderPageCallback: function (ctx, pageIndex, pageSize) { ctx.beginPath(); ctx.moveTo(0, 0); ctx.lineTo(pageSize.width, pageSize.height); ctx.stroke(); ctx.font = "30px Comic Sans MS"; ctx.fillStyle = "red"; ctx.textAlign = "center"; ctx.fillText( `Generated for John Doe. Page ${pageIndex + 1}`, pageSize.width / 2, pageSize.height / 2 ); } });
See the guide on adding watermarks for more details.
The image below shows how the resulting page now appears.
Adding Form Elements
PSPDFKit enables you to add, remove, and modify interactive form elements to allow things like user input or requesting a signature. These elements include text input, radio buttons, and checkboxes:
const radioWidget1 = new PSPDFKit.Annotations.WidgetAnnotation({ id: PSPDFKit.generateInstantId(), pageIndex: 0, formFieldName: "MyFormField", boundingBox: new PSPDFKit.Geometry.Rect({ left: 100, top: 100, width: 20, height: 20 }) }); const radioWidget2 = new PSPDFKit.Annotations.WidgetAnnotation({ id: PSPDFKit.generateInstantId(), pageIndex: 0, formFieldName: "MyFormField", boundingBox: new PSPDFKit.Geometry.Rect({ left: 130, top: 100, width: 20, height: 20 }) }); const formField = new PSPDFKit.FormFields.RadioButtonFormField({ name: "MyFormField", annotationIds: new PSPDFKit.Immutable.List([ radioWidget1.id, radioWidget2.id ]), options: new PSPDFKit.Immutable.List([ new PSPDFKit.FormOption({ label: "Option 1", value: "1" }), new PSPDFKit.FormOption({ label: "Option 2", value: "2" }) ]), defaultValue: "1" }); instance.create([radioWidget1, radioWidget2, formField]);
See our form creation guide for more details.
Adding Bookmarks
In some instances, you might want to bookmark important pages in your PDF report before it’s generated. With PSPDFKit, you can programmatically add bookmarks to your document with JavaScript:
const bookmark = new PSPDFKit.Bookmark({ name: "test bookmark", action: new PSPDFKit.Actions.GoToAction({ pageIndex: 3 }) }); instance.create(bookmark);
You can also easily add bookmarks using the sidebar UI.
Flattening Annotations
Flattening annotations can be useful, as this prevents users from modifying them. Using the sample code below, you can flatten all annotations:
await instance.applyOperations({ type: "flattenAnnotations" });
See our document editor guide for more details.