Adding Stamp Annotations to PDFs Using JavaScript
PSPDFKit for Web supports stamp annotation templates, which are stamp and image annotations that can be selected and used repeatedly within a document.
The SDK includes a default set of these templates with predefined text, subtitles, colors, background colors and box shapes, or images.
User Interface
It’s possible to select a stamp annotation template from the stamp annotation template picker, which shows the current list of available stamp annotation templates. In spite of what the name suggests, it can hold both stamp annotations and image annotations.

It’s also possible to create a custom stamp annotation with personalized text and settings in the custom stamp editor.
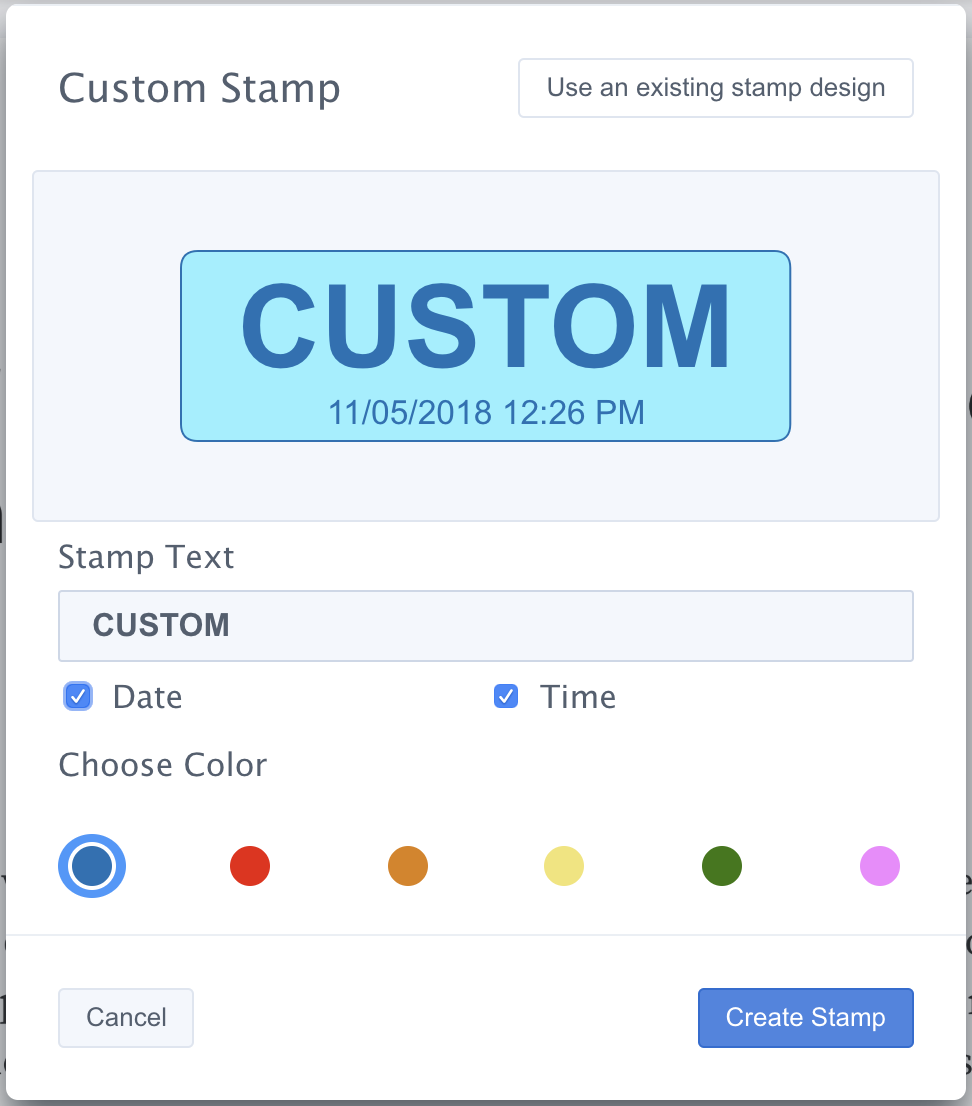
You can access the stamp annotation templates UI via the Stamp button in the toolbar.
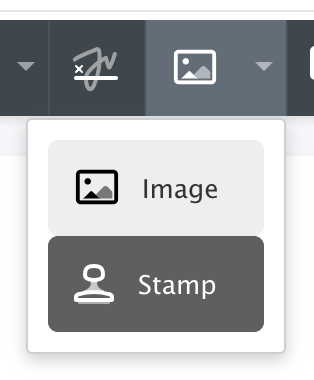
Navigating between both components is easy when using the top right button of the stamp annotation templates’ modal window.
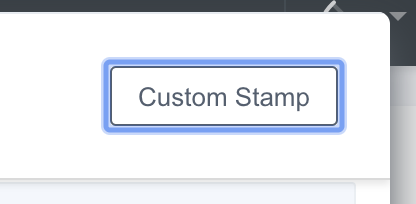
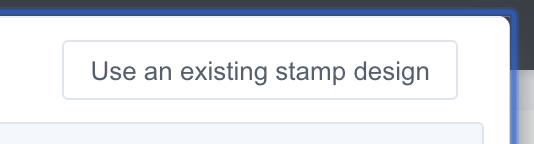
Default Stamp Annotation Templates
By default, PSPDFKit for Web includes some out-of-the-box stamp annotation templates. These are available in the stamp picker UI:
-
Approved
-
NotApproved
-
Draft
-
Final
-
Completed
-
Confidential
-
ForPublicRelease
-
NotForPublicRelease
-
ForComment
-
Void
-
PreliminaryResults
-
InformationOnly
-
Accepted (tick)
-
Rejected (cross)
-
InitialHere
-
SignHere
-
Witness
-
RejectedWithText (with localized date and time)
-
Revised (with localized date and time)
-
Custom
Note: Custom is a special stamp type that can have the text, color, and subtitle modified. The custom stamp editor UI allows your users to edit this text and add the current time and/or date as the subtitle.
Modifying the Stamp Annotation Templates
You can modify the list of stamp annotation templates and add the modified list to the configuration object passed to PSPDFKit.load
:
var stampAnnotationTemplates = PSPDFKit.defaultStampAnnotationTemplates; stampAnnotationTemplates.push( new PSPDFKit.Annotations.StampAnnotation({ stampType: "Custom", title: "My custom text", subtitle: "my custom subtitle", color: new PSPDFKit.Color({ r: 0, g: 0, b: 64 }), boundingBox: new PSPDFKit.Geometry.Rect({ left: 0, top: 0, width: 300, height: 100 }) }) ); PSPDFKit.load({ stampAnnotationTemplates: stampAnnotationTemplates });
const stampAnnotationTemplates = [ ...PSPDFKit.defaultStampAnnotationTemplates, new PSPDFKit.Annotations.StampAnnotation({ stampType: "Custom", title: "My custom text", subtitle: "my custom subtitle", color: new PSPDFKit.Color({ r: 0, g: 0, b: 64 }), boundingBox: new PSPDFKit.Geometry.Rect({ left: 0, top: 0, width: 300, height: 100 }) }) ]; PSPDFKit.load({ stampAnnotationTemplates });
You can also do this at any moment by setting the current list of stamp annotation templates with Instance#setStampAnnotationTemplates
:
instance.setStampAnnotationTemplates(function(stampAnnotationTemplates) { stampAnnotationTemplates.push( new PSPDFKit.Annotations.StampAnnotation({ stampType: "Custom", title: "My custom text", subtitle: "my custom subtitle", color: new PSPDFKit.Color({ r: 0, g: 0, b: 64 }), boundingBox: new PSPDFKit.Geometry.Rect({ left: 0, top: 0, width: 300, height: 100 }) }) ); return stampAnnotationTemplates; });
instance.setStampAnnotationTemplates(stampAnnotationTemplates => [ ...stampAnnotationTemplates, new PSPDFKit.Annotations.StampAnnotation({ stampType: "Custom", title: "My custom text", subtitle: "my custom subtitle", color: new PSPDFKit.Color({ r: 0, g: 0, b: 64 }), boundingBox: new PSPDFKit.Geometry.Rect({ left: 0, top: 0, width: 300, height: 100 }) }) ];