Signatures (Legacy)
ℹ️ Note: This guide is written for the older signatures functionality included as part of the Annotations component. Please see our newer documentation on Electronic Signatures and the corresponding migration guide.
PSPDFKit for Web supports signature form fields (pspdfkit/form-field/signature
) that represent handwritten signatures and can be signed using ink annotation signatures.
Ink signatures are regular ink annotations that are automatically associated to a form field when their bounding box overlaps the form field’s bounding box.
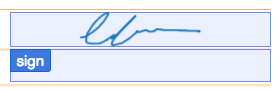
User Interface
It’s possible to create an ink signature by clicking a signable form field or by using the main toolbar button for ink signatures.
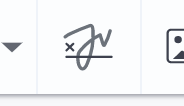
When signing with a form element, the signature will be adapted to fit in the form element. If the signature is created via the toolbar button, the signature will instead be centered on the page.
When there are no stored signatures, tapping an empty signature form element shows a signature modal dialog, which is where you can sign a document by drawing an ink annotation for your signature.
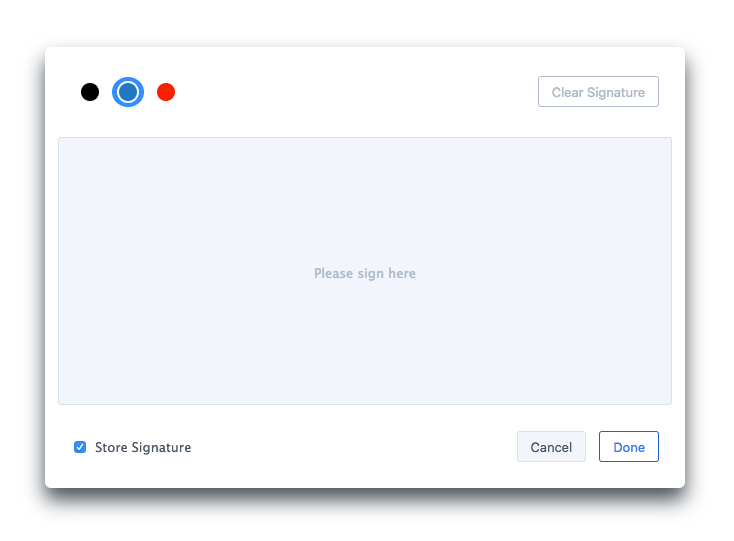
If there are already stored signatures, a signature picker is shown instead. This allows you to choose an existing signature or create a new one.
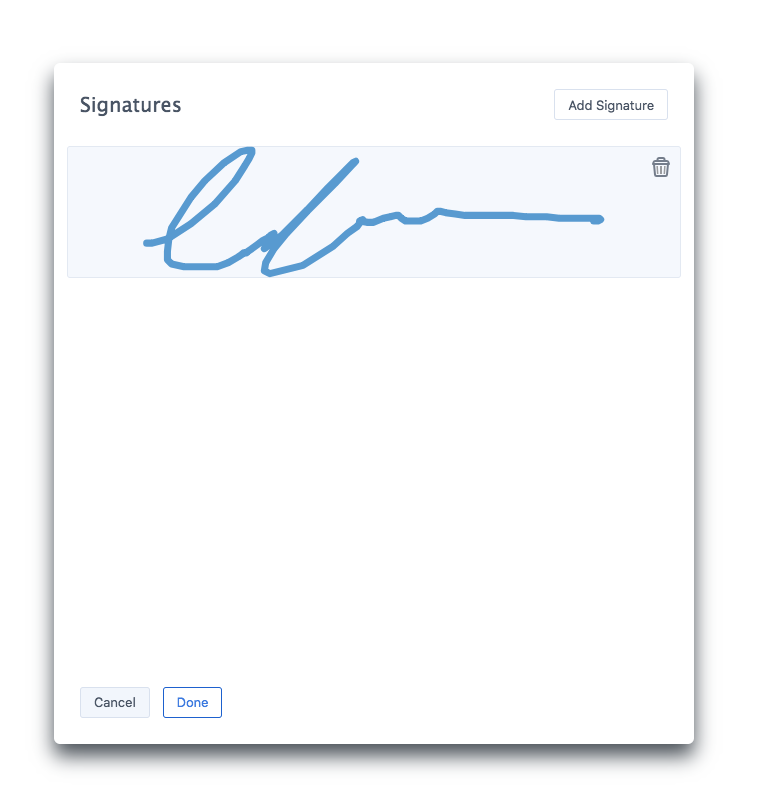
Storing Signatures
When creating a new signature, checking the Store Signature option allows you to temporarily store the annotation for exporting later.
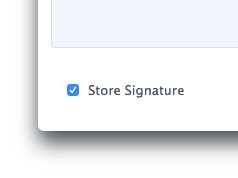
Stored ink signatures are available via the Signature Picker or the API method Instance#getInkSignatures
. This method returns a Promise
that resolves to a PSPDFKit.Immutable.List
of ink annotations:
const annotations = await instance.getInkSignatures();
This list can be converted to serializable JavaScript objects using the PSPDFKit.Annotations.toSerializableObject
helper:
const annotations = await instance.getInkSignatures(); JSON.stringify( annotations .map((annotation) => PSPDFKit.Annotations.toSerializableObject(annotation)) .toJS() );
Serialized annotations can be stored in localStorage
or a database for reuse.
Loading Signatures
It’s possible to preload a list of signature templates when PSPDFKit for Web is initialized. To do so, you must define the populateInkSignatures
option in the main configuration object. The value for this option must be a function that returns a Promise
that resolves to a PSPDFKit.Immutable.List
of ink annotations.
Serialized annotations can be converted to PSPDFKit-compliant annotations using the PSPDFKit.Annotations.fromSerializableObject
helper:
const populateInkSignatures = () => { return fetch("/your-ink-annotations-endpoint").then((annotations) => { return PSPDFKit.Immutable.List( JSON.parse(annotations).map((annotation) => PSPDFKit.Annotations.fromSerializableObject(annotation) ) ); }); }; PSPDFKit.load({ populateInkSignatures: populateInkSignatures // ... });
Updating Signatures
Ink signatures can also be updated at any time using the Instance#setInkSignatures
method:
// Delete all locally stored ink signatures.
instance.setInkSignatures(PSPDFKit.Immutable.List());
Preventing Form Fields from Saving Signatures
In certain situations, it might be useful to disable signature storage for some form fields. This is often the case when the PDF file is accessible to multiple users — in such a scenario, you might not want to save signatures.
PSPDFKit for Web allows you to define a list of form field names that are not allowed to save signatures. This can be done by passing a list of names to the initial configuration object:
PSPDFKit.load({ formFieldsNotSavingSignatures: ["signatureFieldName1", "anotherFieldName"] // ..., });
Creating Signature Form Fields
PSPDFKit for Web provides APIs for creating and modifying signature form fields if the Form Creator component is included in your license. This allows you to modify the properties of the associated widget, as well as attach JavaScript actions in response to user input. Please see the Form Creation guide for more information.