Converting HTML to PDF on iOS
Processor
provides a powerful API for generating PDF files directly from HTML strings. The conversion is fast, but it only supports simple HTML tags, such as <strong>
, <a>
, or <font>
. You can use the following methods for this:
-
Processor.generatePDF(fromHTMLString:options:completionBlock:)
— generates a PDF from an HTML string and keeps it in memory -
Processor.generatePDF(fromHTMLString:outputFileURL:options:completionBlock:)
— generates a PDF from an HTML string and saves it to disk at the providedoutputFileURL
Generating PDF Files from Complex HTML
If you want to generate a PDF file from more complex HTML, you can use another technique — first, save your HTML to a temporary local file, and then generate a PDF file from that URL. PSPDFKit utilizes the full power of WebKit when generating PDF files from URLs, allowing you to use CSS, embed images, and take advantage of a variety of tags and modern HTML constructs. This is something that isn’t possible with simple HTML-to-PDF conversion.
ℹ️ Note: Using these APIs requires the HTML-to-PDF Conversion component in your license.
let htmlURL: URL = ... // URL to save temporary HTML file to. let pdfURL: URL = ... // URL to save converted PDF file to. let html = """ <html> <head> <style type="text/css"> h1 { color: red; } </style> </head> <body> <h1>Hello, world!</h1> </body> </html> """ do { try html.data(using: .utf8)!.write(to: htmlURL) } catch { // Handle the error. } Processor.generatePDF(from: htmlURL, outputFileURL: pdfURL, options: nil) { outputURL, error in if let outputURL = outputURL { let document = Document(url: outputURL) // Handle the document. } else if let error = error { // Handle the error. } }
ℹ️ Note: Generating PDF files from URLs is a more time- and memory-consuming operation than regular HTML-to-PDF conversion. Make sure to perform the conversion and opt to do simple HTML-to-PDF conversion if possible.
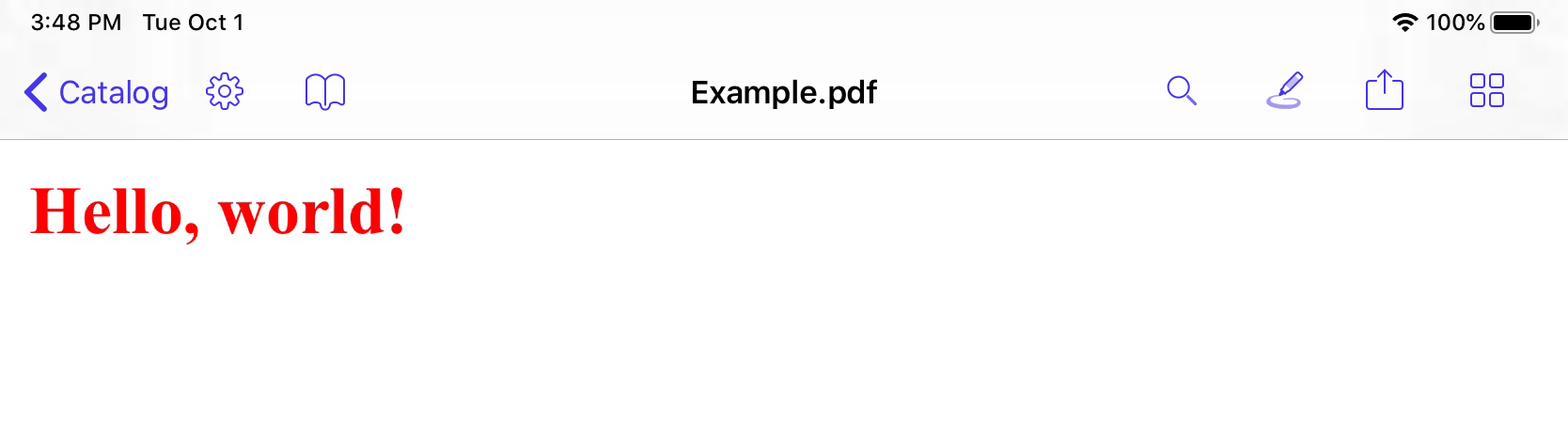
For more details about how to generate PDF files from HTML strings, URLs, and attributed strings, take a look at the Processor
documentation and ConvertHTMLToPDFExample
from our Catalog app.