Compare Documents on iOS
Document Comparison is used to visually compare pages of different documents. It’s helpful for things such as construction plans and detailed drawings, as well as other content that requires precise placement.
This can be done in PSPDFKit using ComparisonProcessor
. The process of preparing documents and comparing them involves a few steps to specify how the comparison should happen.
ℹ️ Note: The APIs described in this guide require the Document Comparison component to be enabled in your license.
Changing the Stroke Colors
One of the most important steps of generating a comparison document is the ability to change the stroke colors, which makes it easier to see the differences between two versions of a document.
Setting a different stroke color is usually the first step when trying to compare documents, as this will enable you to make any differences between pages more obvious. This will only affect stroke objects in the PDF, and it will leave the color of other elements, such as text or images, unchanged.
First, create a new ComparisonConfiguration
object that allows you to customize certain aspects of the generated comparison document, described below.
The stroke colors of both versions of a document can be changed using the oldDocumentStrokeColor
and newDocumentStrokeColor
properties:
let configuration = ComparisonConfiguration { $0.oldDocumentStrokeColor = .systemOrange $0.newDocumentStrokeColor = .systemGreen }
The table below shows how applying an orange stroke color changes the appearance of a document.
Original Document | Stroked Document |
---|---|
![]() |
![]() |
You can also change the blend mode used to overlay the new version of a document on top of the old one by changing the blendMode
property.
Trying out various stroke colors and blend modes will result in different-looking comparison documents, and you can make sure the final result fits your needs.
Changing the Working Directory
By default, the ComparisonProcessor
will generate a comparison document in the system temporary directory. To customize this behavior, change the workingDirectory
URL of ComparisonConfiguration
.
Generating the Comparison Document
You can use ComparisonProcessor
to generate a comparison document. First, initialize it with a ComparisonConfiguration
, and then call the comparisonDocument(...)
function, passing the two versions of a document to be compared as arguments:
let oldDocument: Document = /* the old version of a document */ let newDocument: Document = /* the new version of a document */ let configuration = ComparisonConfiguration.default() let processor = ComparisonProcessor(configuration: configuration) let comparisonDocument = try processor.comparisonDocument( oldDocument: oldDocument, newDocument: oldDocument )
By default, the processor will generate a comparison document using the first page of both versions of the document. If the page you want to compare has moved, or if it’s not the first page, you can specify explicit indices using extra pageIndex
arguments:
let comparisonDocument = try processor.comparisonDocument( oldDocument: oldDocument, pageIndex: 2, newDocument: oldDocument, pageIndex: 3 )
If either of the passed documents is password protected, make sure to unlock it before passing it into the processor.
ℹ️ Note: It’s only possible to compare one page at a time.
The below table shows how a comparison document generated from two different but similar-looking documents that previously had their stroke colors changed would look.
Old Document | New Document |
---|---|
![]() |
![]() |
Comparison Document | |
![]() |
Aligning the Documents
If the two versions of the document you want to compare aren’t perfectly aligned with each other, PSPDFKit provides a way for users to visually select two corresponding sets of landmark points in both versions of the document, and it automatically calculates a transformation matrix based on that.
To initiate the document alignment process, create a DocumentAlignmentViewController
with the two versions of a document and a ComparisonConfiguration
:
let oldDocument: Document = /* The old version of a document. */ let newDocument: Document = /* The new version of a document. */ let viewController = DocumentAlignmentViewController( oldDocument: oldDocument, newDocument: newDocument, configuration: .default() )
You can wrap the document alignment view controller in a UINavigationController
and customize its navigation bar button items. The document alignment process begins in the old version of the document, and then it automatically moves on to the new version once the user selects all three points in the old one.
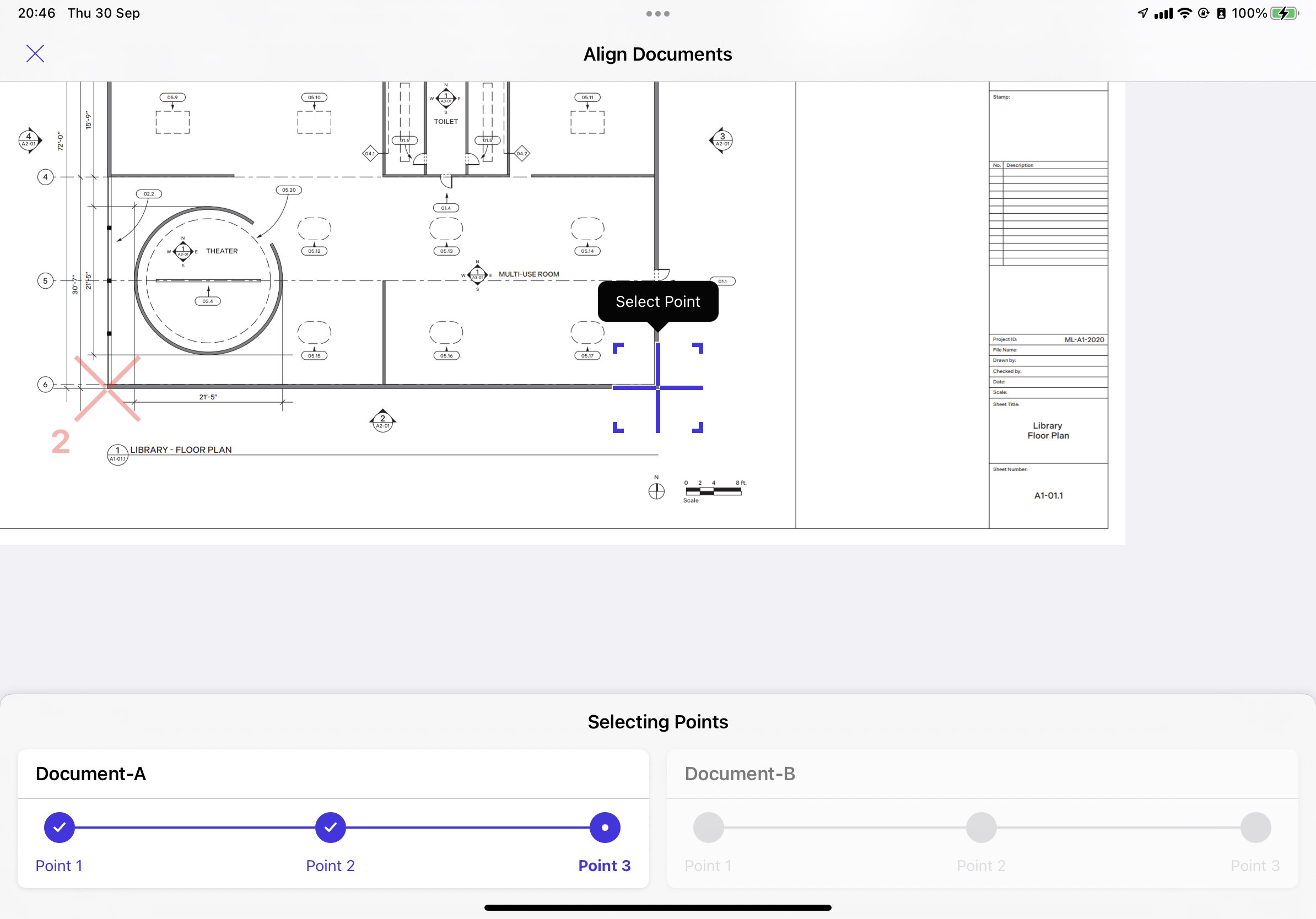
It’s crucial to select the same three landmark points, in the same order. The points should be chosen such that they don’t move, relative to each other. Selecting a point that changes position will result in corrupted comparison documents. The below table shows the recommended landmark points.
Points in the Old Document | Points in the New Document |
---|---|
![]() |
![]() |
Once all six points are selected, the document alignment view controller will automatically calculate a transformation matrix, try to generate a comparison document, and call one of its delegate methods, depending on whether or not the generation succeeded:
func documentAlignmentViewController(_ sender: DocumentAlignmentViewController, didFinishWithComparisonDocument document: Document) { /* Display the comparison document. */ } func documentAlignmentViewController(_ sender: DocumentAlignmentViewController, didFailWithError error: Error) { /* Show the error. */ }
ℹ️ Note: As an alternative to implementing the delegate methods, you can subscribe to the
comparisonDocument
future. The document alignment view controller will both call its delegate methods and send either a document or an error to the future.
The image below shows a comparison document aligned based on the two sets of three landmark points shown above.
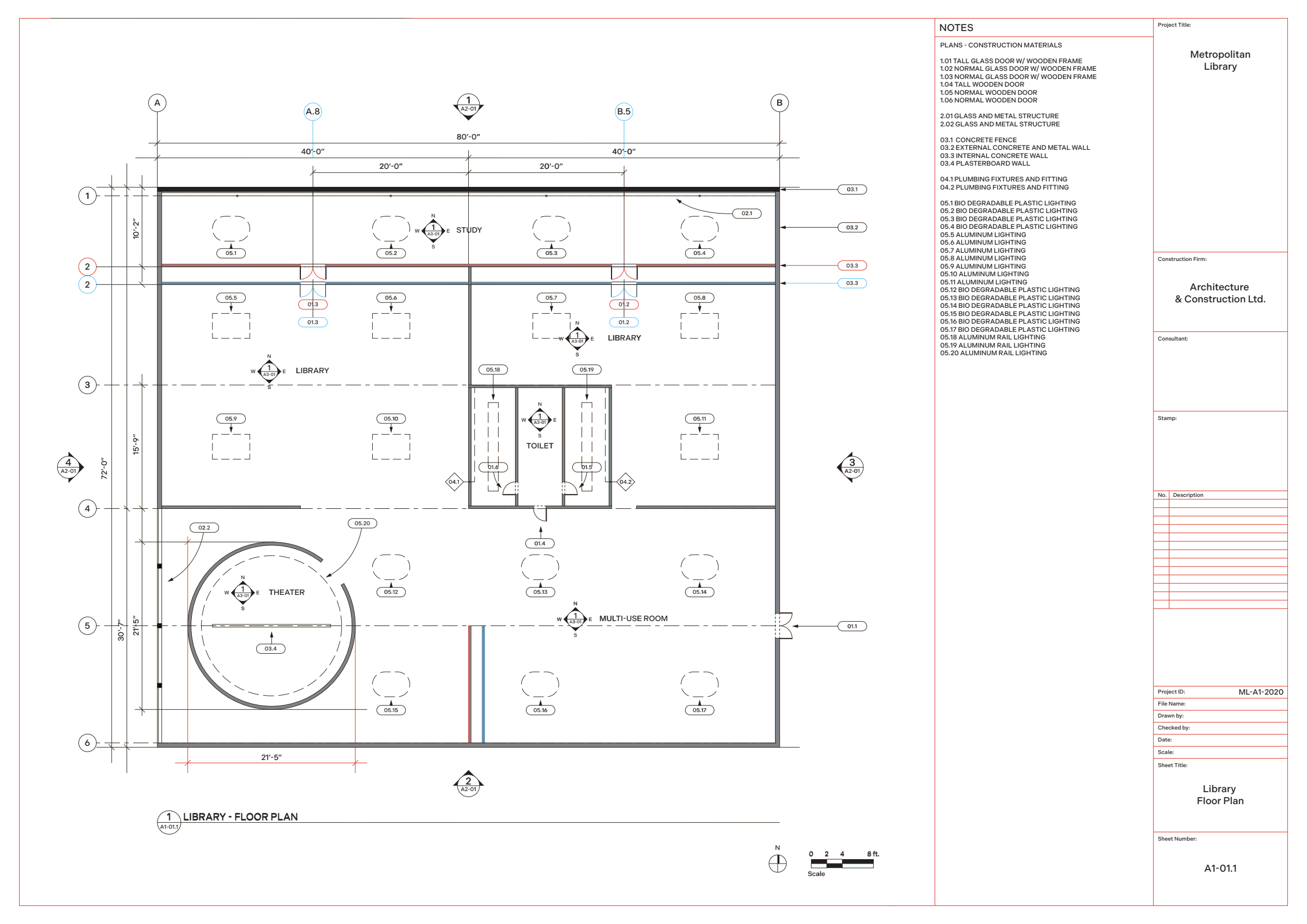
Example
Check out ComparisonExample.swift
in our PSPDFKit Catalog example project to see the document comparison and alignment APIs in action.