![]()
PSPDFKit Processor has been deprecated and replaced by PSPDFKit Document Engine. All PSPDFKit Processor licenses will work as before and be supported until 15 May 2024 (we will contact you about license migration). To start using Document Engine, refer to the migration guide. With Document Engine, you’ll have access to robust new capabilities (read the blog for more information).
This guide walks you through the steps necessary to start PSPDFKit Processor. It also shows you how to use it to process documents. By the end, you’ll be able to merge two PDF documents into one using Processor’s HTTP API from PHP.
Requirements
PSPDFKit Processor runs on a variety of platforms. The following operating systems are supported:
-
macOS Ventura, Monterey, Mojave, Catalina, or Big Sur
-
Windows 10 Pro, Home, Education, or Enterprise 64-bit
-
Ubuntu, Fedora, Debian, or CentOS. Ubuntu and Debian derivatives such as Kubuntu or Xubuntu are supported as well. Currently only 64-bit Intel (x86_64) processors are supported.
Regardless of your operating system, you’ll need at least 4 GB of RAM.
Installing Docker
PSPDFKit Processor is distributed as a Docker container. To run it on your computer, you need to install a Docker runtime distribution for your operating system.
Install and start Docker Desktop for Mac. Please refer to the Docker website for instructions.
Install and start Docker Desktop for Windows. Please refer to the Docker website for instructions.
Install and start Docker Engine. Please refer to the Docker website for instructions on how to install it for your Linux distribution.
After you install Docker, use these instructions to install Docker Compose.
Starting PSPDFKit Processor
First, open your terminal emulator.
Use the terminal emulator integrated with your code editor or IDE. Alternatively, you can use Terminal.app
or iTerm2.
Use the terminal emulator integrated with your code editor or IDE. Alternatively, you can use PowerShell.
Use the terminal emulator integrated with your code editor or IDE, or one bundled with your desktop environment.
Now run the following command:
docker run --rm -t -p 5000:5000 pspdfkit/processor:2023.11.1
This command might take a while to run, depending on your internet connection speed. Wait until you see a message like this in the terminal:
[info] 2023-02-05 18:56:45.286 Running PSPDFKit Processor version 2023.11.1. pid=<0.1851.0>
The PSPDFKit Processor is now up and running!
Installing PHP
The interaction with Processor happens via its HTTP API: You send documents and commands in the request and receive the resulting file in the response. To do this, you’ll invoke the API from the PHP script. But first, you need to install PHP for your operating system:
The easiest way to install PHP on macOS is via Homebrew. Follow the instructions on the Homebrew website to install it. Then, to install PHP, run:
brew install php@7.4 && brew link php@7.4
Verify the installation by running the following command in the terminal:
php --version
The output should start with PHP 7.4
— you can ignore the rest of the message.
ℹ️ Note: If the output doesn’t match the above, try restarting your terminal app by typing
exit
and opening it again.
-
Download the PHP ZIP archive from the PHP website (pick the x86 Thread Safe build of the 7.4 release).
-
Create a folder anywhere on your C: drive.
-
Extract the ZIP archive into the folder you just created.
-
Open the terminal and switch to that folder:
cd C:\path\to\directory
Now run the .\php.exe --version
command. The output should start with PHP 7.4
— you can ignore the rest of the message.
To proceed, you’ll also need to create a PHP configuration file to enable a specific extension. So in the same directory, create a php.ini
file with the following content:
[PHP]
extension=curl
Save the file, as you’ll need it shortly.
You can install PHP using your distribution’s package manager:
apt-get update && apt-get install -y php
dnf install -y php
Now run the php --version
command. The output should start with PHP 7.4
— you can ignore the rest of the message.
Handling File Uploads
In this example project, the PDF files you’ll merge will be uploaded through a simple webpage via a standard HTML form. Create a file called index.php
with the following content:
<!DOCTYPE html> <html> <head> <title>Merge PDFs with PSPDFKit Processor</title> </head> <body> <p>Upload the files to merge:</p> <form enctype="multipart/form-data" action="merge.php" method="post"> <div>File 1: <input name="file1" type="file" accept="application/pdf"></div> <div>File 2: <input name="file2" type="file" accept="application/pdf"></div> <input type="submit" value= "Merge PDFs"> </form> </body> </html>
Now open the terminal and type the following command in the same directory where you created the index.php
file:
php -S localhost:8000
.\php.exe -c php.ini -S localhost:8000
php -S localhost:8000
Go to http://localhost:8000 in the browser. You should see a webpage similar to this:
When you choose files and click the Merge PDFs button, you’ll receive an error. This is because you haven’t yet written any code to handle the form submission.
Create a merge.php
file in the same directory and add the following content to it:
<?php $file1 = $_FILES['file1']; $file2 = $_FILES['file2']; echo $file1['name'], ", ", $file2['name']; ?>
Now when you go back to http://localhost:8000, choose the files, and submit the form, you should see the names of the files you picked printed on the screen:
Merging PDFs
You can now use Processor’s API to merge the files uploaded from the browser. Replace the contents of the merge.php
file with:
<?php $file1 = $_FILES["file1"]; $file2 = $_FILES["file2"]; $headers = ["Content-Type" => "multipart/form-data"]; $postFields = []; $postFields["document1"] = curl_file_create( $file1["tmp_name"], $file1["type"], $file1["name"] ); $postFields["document2"] = curl_file_create( $file2["tmp_name"], $file2["type"], $file2["name"] ); $postFields["instructions"] = json_encode([ "parts" => [ [ "file" => "document1" ], [ "file" => "document2" ] ], ]); $request = curl_init(); curl_setopt($request, CURLOPT_URL, "http://localhost:5000/build"); curl_setopt($request, CURLOPT_HTTPHEADER, $headers); curl_setopt($request, CURLOPT_POST, true); curl_setopt($request, CURLOPT_POSTFIELDS, $postFields); curl_setopt($request, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($request); $status = curl_getinfo($request, CURLINFO_RESPONSE_CODE); $file_size = curl_getinfo($request, CURLINFO_CONTENT_LENGTH_DOWNLOAD); curl_close($request); if ($status != 200) { echo "Request to Processor failed with status code " . $status . ': "' . $response . '".'; } else { header("Content-type: application/pdf"); header('Content-Disposition: attachment; filename="result.pdf"'); header("Content-Transfer-Encoding: binary"); header("Content-Length: " . $file_size); header("Accept-Ranges: bytes"); echo $response; }
Most of this code, up until the curl_exec
function, constructs a request that’s sent to PSPDFKit Processor. It includes two files — document1
and document2
— and a list of instructions
for Processor. By default, Processor’s output (the /build
endpoint) is the result of merging all documents or parts of the instructions
. To learn more about the /build
instructions, go to Processor’s API Reference.
The rest of the code deals with error handling, and if everything goes well, it returns the resulting file back to the browser.
You can check how it works in practice yourself! Go to http://localhost:8000, pick any two PDFs on your disk (or use these two if you don’t have any: file1.pdf, file2.pdf), and click Merge PDFs again. Depending on your browser, it will either automatically download the file for you or ask you for permission to download. In any case, look for the result.pdf
file in the downloads folder on your computer. Open that file in any PDF viewer application. If you used the two files from the links above, you should see a five-page PDF document like this:
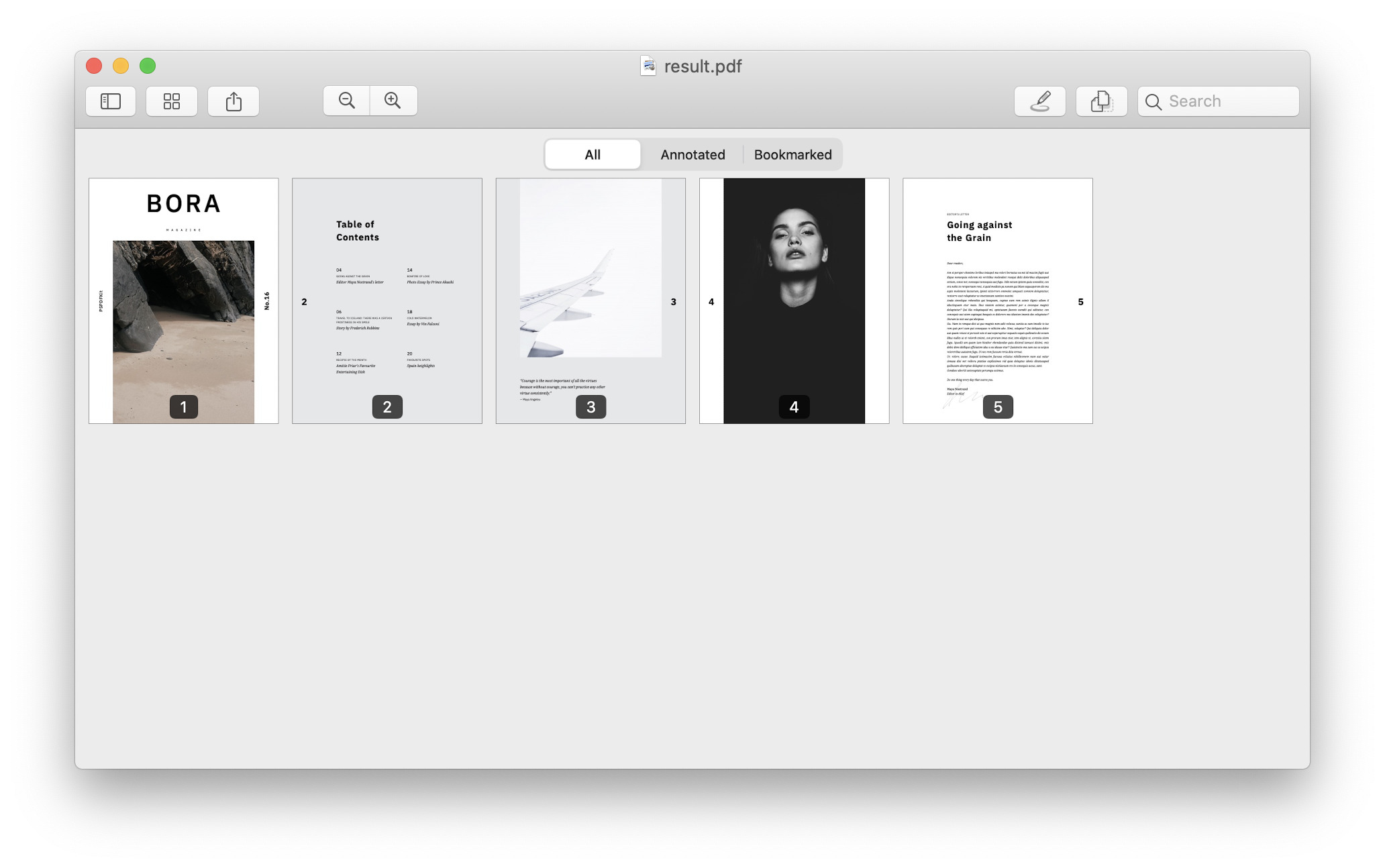
That’s it! Now you know how to use Processor from PHP to perform operations on documents.