How to Fill a PDF Form in React
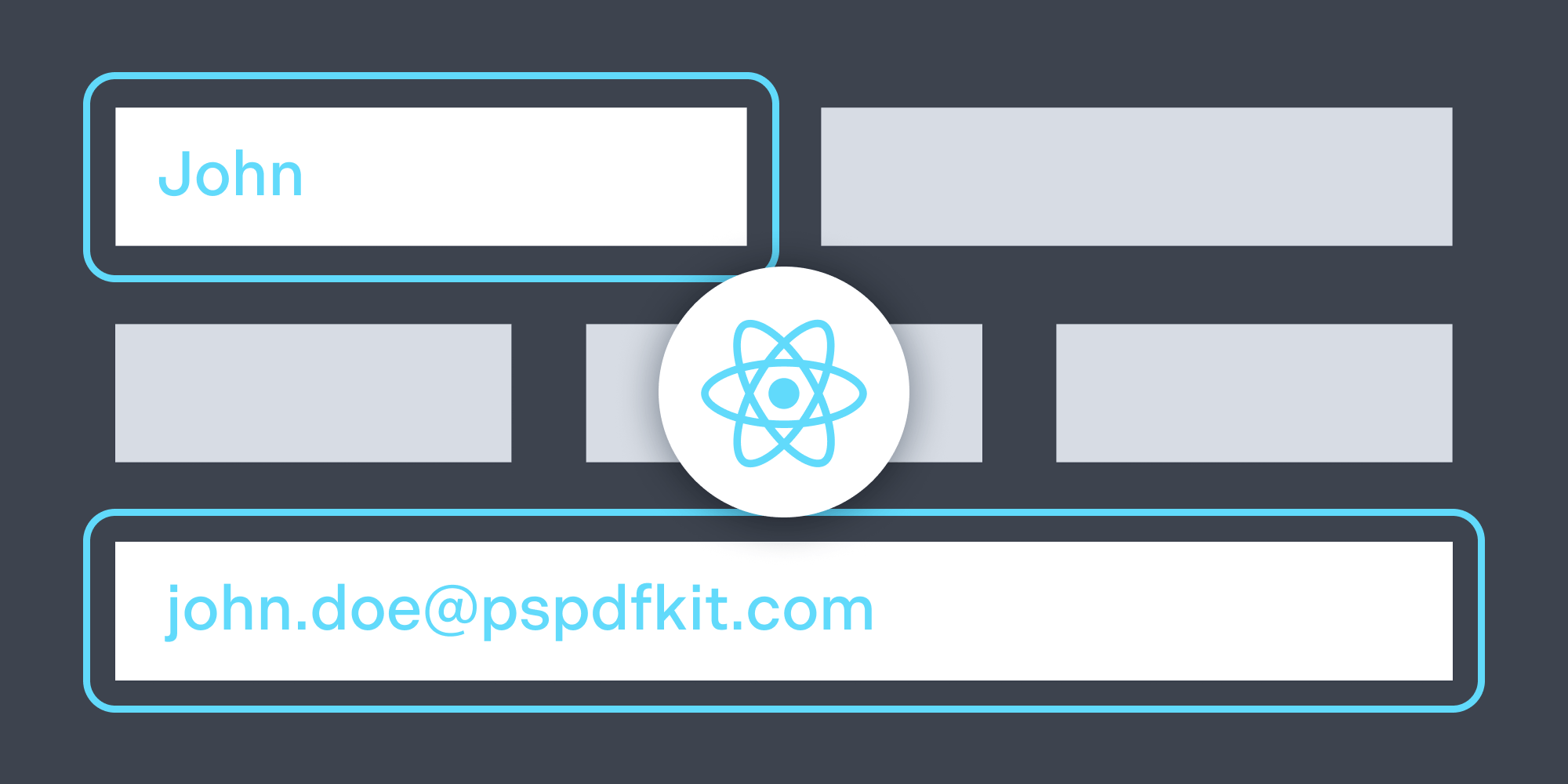
Filling out PDF forms programmatically can be a useful feature in many applications. In this tutorial, you’ll learn how to fill a PDF form using the pdf-lib
library within a React project. The pdf-lib
library provides a straightforward way to create, modify, and save PDF documents.
Prerequisites
Before beginning, make sure you have the following installed:
Setting Up the Project
-
Create a new React project by running the following command in your terminal:
npx create-react-app pdf-form-filler
-
Change into the project directory:
cd pdf-form-filler
-
Install the
pdf-lib
library by running the following command:
npm install pdf-lib
Loading and Filling the PDF Form
In this step, you’ll load the PDF form and fill it with the desired values. Open the src/App.js
file in your text editor and replace its content with the following code:
import { PDFDocument } from 'pdf-lib'; const App = () => { const fillForm = async () => { // Step 1: Load the PDF form. const formUrl = 'https://pdf-lib.js.org/assets/dod_character.pdf'; const formPdfBytes = await fetch(formUrl).then((res) => res.arrayBuffer(), ); const pdfDoc = await PDFDocument.load(formPdfBytes); // Step 2: Retrieve the form fields. const form = pdfDoc.getForm(); const nameField = form.getTextField('CharacterName 2'); const ageField = form.getTextField('Age'); const heightField = form.getTextField('Height'); const weightField = form.getTextField('Weight'); const eyesField = form.getTextField('Eyes'); const skinField = form.getTextField('Skin'); const hairField = form.getTextField('Hair'); // Step 3: Set values for the form fields. nameField.setText('Mario'); ageField.setText('24 years'); heightField.setText(`5' 1"`); weightField.setText('196 lbs'); eyesField.setText('blue'); skinField.setText('white'); hairField.setText('brown'); // Step 4: Save the modified PDF. const pdfBytes = await pdfDoc.save(); // Step 5: Create a `Blob` from the PDF bytes, const blob = new Blob([pdfBytes], { type: 'application/pdf' }); // Step 6: Create a download URL for the `Blob`. const url = URL.createObjectURL(blob); // Step 7: Create a link element and simulate a click event to trigger the download. const link = document.createElement('a'); link.href = url; link.download = 'filled_form.pdf'; link.click(); }; return ( <div> <h1>PDF Form Filler</h1> <button onClick={fillForm}>Fill Form</button> </div> ); }; export default App;
Let’s break down the steps involved:
-
Load the PDF form — You start by fetching the PDF form using its URL and loading it into a PDF document using the
PDFDocument.load
method. -
Retrieve the form fields— Next, you get a reference to the form within the PDF document using
pdfDoc.getForm()
. You then retrieve individual form fields using their field names. -
Set values for the form fields — After obtaining references to the form fields, you can set the desired values using the
setText
method provided by each form field. -
Save the modified PDF — Once the form fields are filled, you save the modified PDF using
pdfDoc.save()
to generate the updated PDF document with the filled form fields. -
Create a
Blob
from the PDF bytes — You create aBlob
object from the generated PDF bytes using theBlob
constructor. TheBlob
represents the PDF data as a file-like object. -
Create a download URL for the
Blob
— You create a download URL for theBlob
usingURL.createObjectURL
. This URL allows you to download the PDF file. -
Trigger the download — Finally, you create a link element (
<a>
) dynamically, set its href attribute to the download URL, specify the desired file name in the download attribute, and simulate a click event on the link to trigger the download of the filled PDF form.
Running the Application
To run the application, execute the following command in the project directory:
npm start
![]()
Interact with the sandbox by clicking the left rectangle icon and selecting Editor > Show Default Layout. To edit, sign in with GitHub — click the rectangle icon again and choose Sign in. To preview the result, click the rectangle icon once more and choose Editor > Embed Preview. For the full example, click the Open Editor button. Enjoy experimenting with the project!
This will start the development server and open the application in your browser. Clicking the Fill Form button will initiate the form filling process, and the filled PDF form will be downloaded as filled_form.pdf
.
Downsides of Using pdf-lib
While the pdf-lib
library is a powerful tool for working with PDF documents, it does have a few downsides and limitations:
-
Limited UI interactivity — The
pdf-lib
library primarily focuses on programmatic manipulation of PDF documents. It doesn’t provide built-in user interface (UI) components or interactivity for form filling. As a result, users cannot directly type into the form fields within the application’s UI; the form fields need to be programmatically filled using code, as demonstrated in the example. -
Lack of real-time updates — Since the form fields are filled programmatically on the server or within the application code, there’s no real-time update or synchronization with the UI. If the user wants to see their input reflected in the filled form fields, they need to generate and download the updated PDF file.
Form Filling with PSPDFKit
PSPDFKit offers comprehensive form filling capabilities, providing both a user-friendly interface and programmable options.
-
UI form filling — PSPDFKit’s prebuilt UI components allow users to easily navigate and interact with PDF forms. They can fill in text fields, select options from dropdown menus, and interact with checkboxes and radio buttons. Check out the demo to see it in action.
-
Programmatic form filling — PSPDFKit for Web offers versatile programmatic form filling options:
-
PSPDFKit Server — Easily persist, restore, and synchronize form field values across devices without building this ability yourself.
-
XFDF — Exchange form field data with other PDF readers and editors seamlessly.
-
Instant JSON — Efficiently export and import changes made to form fields.
-
Manual API — Have full control over extracting, saving, and manipulating form field values.
-
In addition, PSPDFKit also provides an option for creating PDF forms:
-
PDF Form Creator — Simplify PDF form creation with a point-and-click UI. You can create PDF forms from scratch using an intuitive UI or via the API. Convert static forms into fillable forms, or modify existing forms by letting your users create, edit, and remove form fields in a PDF.
By combining UI-based form filling with programmatic controls, PSPDFKit caters to various use cases, offering a seamless user experience and empowering developers to customize form filling workflows.
Conclusion
In this tutorial, you learned how to fill a PDF form in a React project using the pdf-lib
library. It covered the steps required to load a PDF form, retrieve form fields, set values for the fields, save the modified PDF, and trigger the download of the filled form. You also read about the limitations of using pdf-lib
for form filling and learned how PSPDFKit offers a better alternative.
To learn more about PSPDFKit for Web, start your free trial. Or, launch our demo to see our viewer in action.