How to Convert PDF to Image in Node.js
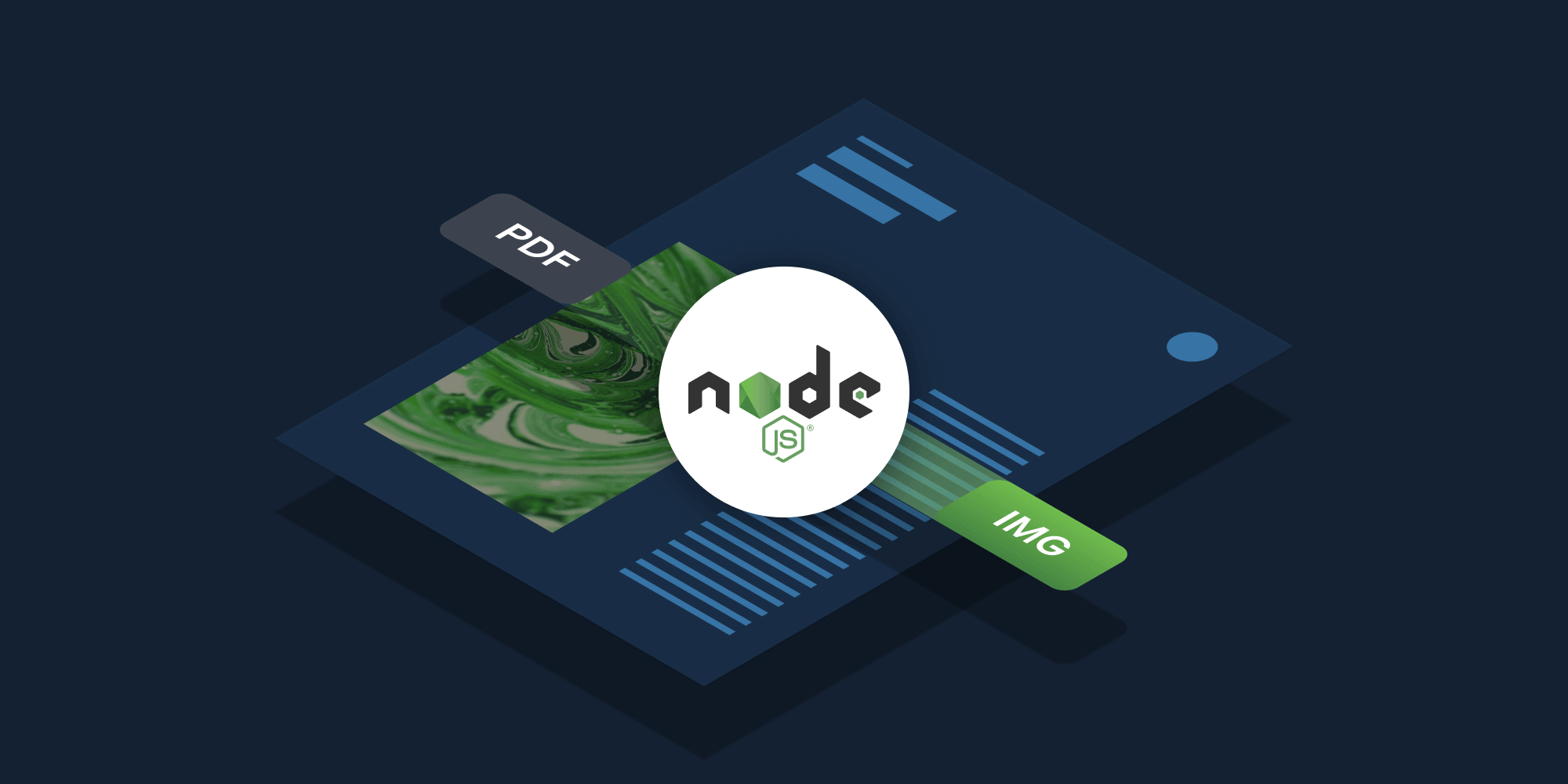
In this blog post, you’ll learn how to convert PDF documents to images using Node.js, a popular runtime for server-side JavaScript. In the first part, you’ll use the pdf2pic library, a Node.js library designed to facilitate the conversion of PDF files into different image formats, Base64 strings, and buffer formats. In the second part, you’ll delve into integrating PSPDFKit for Node.js. You’ll learn how to convert documents step by step and leverage its capabilities for efficient document handling.
Prerequisites
-
Node.js — Make sure you have Node.js installed on your system. You can download it from the official website.
-
Node Package Manager (npm) — npm comes with Node.js and is used to manage packages.
-
GraphicsMagick — GraphicsMagick is a robust image processing library and toolset that’s used as a dependency by the pdf2pic package for image conversion tasks.
-
Ghostscript — Ghostscript is a versatile document processing library and interpreter that’s also used as a dependency by the pdf2pic package.
GraphicsMagick Installation
Make sure you have GraphicsMagick installed on your system. To check if it’s installed, open a terminal or command prompt and type the following command:
gm --version
If GraphicsMagick is installed, you’ll see version information. If you get an error or if the command isn’t recognized, you need to install GraphicsMagick.
-
On macOS (using Homebrew):
brew install graphicsmagick
-
On Linux (using package manager):
sudo apt-get install graphicsmagick
-
On Windows:
Download the installer from the GraphicsMagick website.
Ghostscript Installation
Make sure you have Ghostscript installed on your system. To check if it’s installed, open a terminal or command prompt and type the following command:
gs --version
If Ghostscript is installed, you’ll see version information. If not, you need to install Ghostscript.
-
On macOS (using Homebrew):
brew install ghostscript
-
On Linux (using package manager):
sudo apt-get install ghostscript
-
On Windows:
Download the installer from the Ghostscript website.
Setting Up the Project
-
Start by creating a new directory for your project.
-
Open a terminal window, navigate to your project directory, and run the following command to initialize a new Node.js project:
npm init -y
-
Install the pdf2pic library using npm:
npm install pdf2pic
Converting PDF to Images Using pdf2pic
To convert a specific page of a PDF from a file path and save it as an image file, follow the steps outlined below.
-
Import the
fromPath
function from the pdf2pic module into your code:
import { fromPath } from 'pdf2pic';
-
Define conversion options, such as image quality, format, dimensions, and density. For example:
const options = { density: 100, saveFilename: 'untitled', format: 'png', width: 600, height: 600, };
-
Initialize the conversion by specifying the PDF file’s path and options:
const convert = fromPath('example.pdf', options); const pageToConvertAsImage = 1;
-
Perform the actual conversion using the convert function, and handle the result:
async function convertPdfPageToImage() { try { const result = await convert(pageToConvertAsImage, { responseType: 'image', }); return result; } catch (error) { console.error('Conversion error:', error); } console.log('Page 1 is now converted as an image'); } convertPdfPageToImage();
The code above imports the pdf2pic module, defines conversion options, initializes the conversion, and performs it. The resulting image is saved based on your specified options.
PSPDFKit for Node.js
Unlike pdf2pic, PSPDFKit for Node.js doesn’t rely on third-party software like GraphicsMagick and Ghostscript to convert documents; it relies on its own technology built from the ground up. PSPDFKit for Node.js allows rendering and exporting independent pages of a PDF document, preserving annotations, and overlaying the annotations onto the page background. This capability is particularly valuable when working with documents that contain interactive elements or notes.
Features of PSPDFKit for Node.js
PSPDFKit for Node.js offers a range of features that make it a powerful choice for document conversion within Node.js applications:
-
Versatile Conversion — Convert PDF, Word, Excel, PowerPoint, TIFF, JPG, and PNG files seamlessly, without relying on third-party tools or MS Office licenses.
-
Custom Font Support — Preserve document integrity by handling custom fonts intelligently during conversion, ensuring consistent visual representation.
-
Diverse File Support — Handle a wide array of formats, including DOCX, XLSX, PPTX, and image types like PNG and JPEG.
-
Advanced Rendering — Render PDF pages into PNG or WebP formats for versatile visualization options.
Integrating PSPDFKit for Node.js
-
Initialize a new Node.js project:
npm init -y
This command creates a package.json
file in your project directory, which is essential for managing your project’s dependencies.
-
Install the PSPDFKit for Node.js package:
npm install @pspdfkit/nodejs
-
Place your PDF document (e.g. document.pdf) in your project directory.
-
Create a new JavaScript file named
index.js
in your project directory. This script will handle the conversion process:
const { load } = require('@pspdfkit/nodejs'); const fs = require('fs'); (async () => { const doc = fs.readFileSync('document.pdf'); const instance = await load({ document: doc }); const pageWidth = instance.getDocumentInfo().pages[0].width; const result = await instance.renderPage(0, { width: pageWidth }); fs.writeFileSync('image.png', Buffer.from(result)); instance.close(); })();
The example demonstrates how to save a single page from a PDF document as a PNG file using PSPDFKit for Node.js. Note that the annotations within the document will also be rendered over the page background.
In this script, the PDF document is loaded using the fs
module, and an instance of PSPDFKit is created using the load()
function. You then obtain the width
of the first page using getDocumentInfo().pages[0].width
. The renderPage()
function is used to render the page, and the resulting image data is written to a PNG file using fs.writeFileSync()
. Finally, the instance is closed to release any allocated resources.
![]()
By default, the resulting PDF will include a PSPDFKit watermark. To exclude the watermark, you can specify a
license
property with thekey
andappName
fields when calling theload()
function. For details on obtaining a trial license key, please get in touch with Sales.
-
Execute the script in your terminal to initiate the conversion process:
node index.js
Once executed, the script will convert the PDF document to PNG and save the resulting PNG as image.png
in your project directory.
Choosing the Image Format
By default, PSPDFKit exports the image as a PNG. However, if you prefer to export the image in WebP format, you can modify the call to instance.renderPage()
as shown below:
const result = await instance.renderPage( 0, { width: pageWidth }, 'webp', );
This flexibility enables you to tailor the output format to your specific project requirements.
Conclusion
In this guide, you explored two approaches to document conversion in Node.js applications. The first part covered using the pdf2pic library, enabling the transformation of PDF documents into images.
In the second part, you learned about PSPDFKit for Node.js, a robust SDK offering versatile document conversion features. PSPDFKit empowers you to elevate your Node.js projects, ensuring seamless and effective document management and conversion
To get started with PSPDFKit for Node.js, you can either:
-
Start your free trial to test the library and see how it works in your application.
-
Launch our demo to see the viewer in action.