How to Convert Images to PDF in Node.js
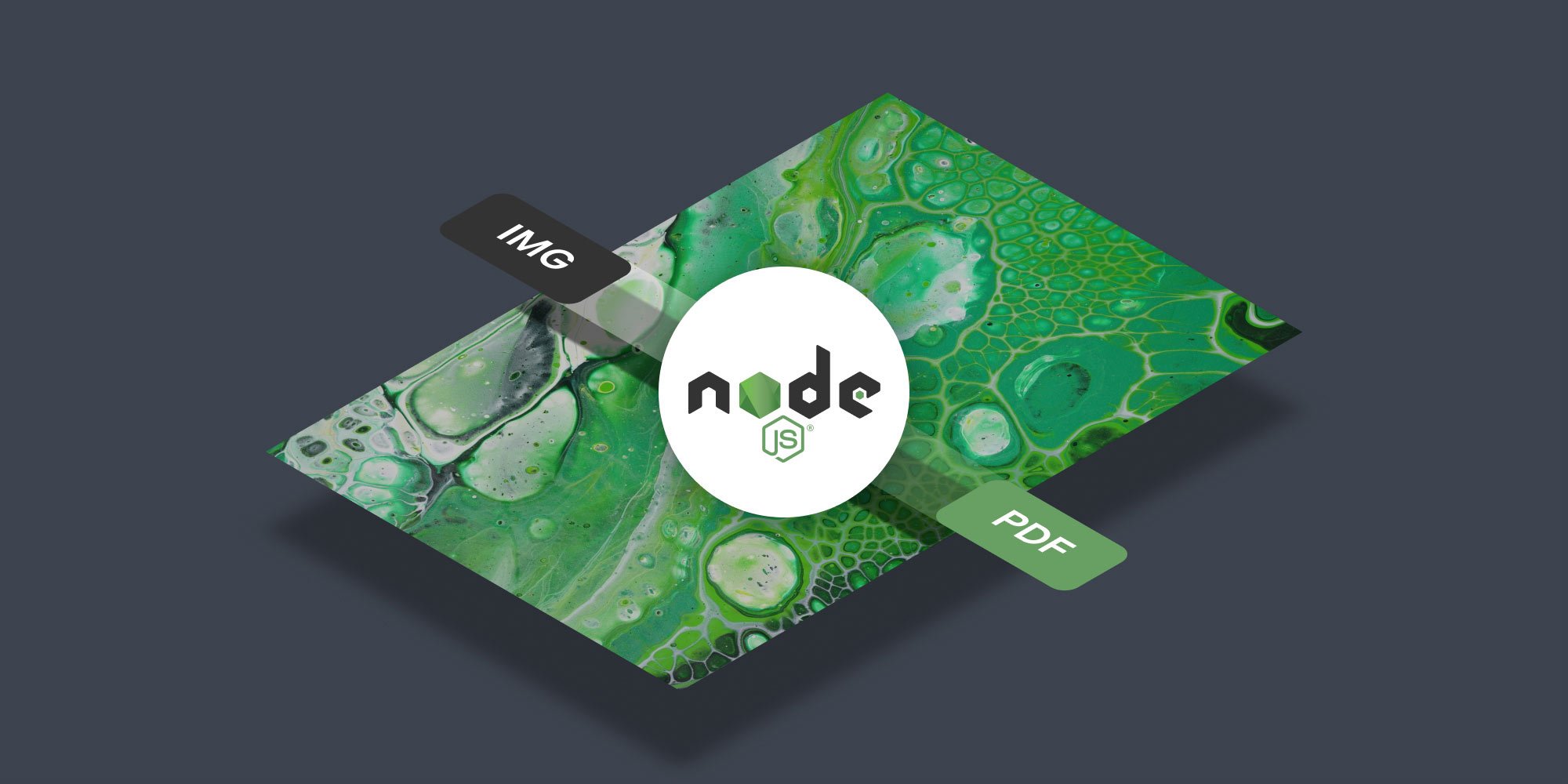
In this tutorial, you’ll learn the process of converting an image to a PDF using Node.js. In the first part, you’ll use the pdf-lib library, and in the second part, you’ll use PSPDFKit for Node.js to convert an image to a PDF.
Prerequisites
Before you begin, make sure you have the following installed:
-
Node.js — You can download it from the official Node.js website.
-
npm — Node Package Manager comes bundled with Node.js, so it’ll be installed by default.
Step 1 — Setting Up Your Project
-
Create a new directory for your project:
mkdir image-to-pdf-converter cd image-to-pdf-converter
-
Initialize a new Node.js project:
npm init -y
-
Install the pdf-lib library:
npm install pdf-lib
Step 2 — Writing the Conversion Code
-
Create a file named
convert.js
in your project directory and add the following code:
const fs = require('fs'); const { PDFDocument, rgb } = require('pdf-lib'); async function convertImageToPdf(imagePath, pdfPath) { // Read the image file asynchronously. const image = await fs.promises.readFile(imagePath); // Create a new PDF document. const pdfDoc = await PDFDocument.create(); // Add a new page to the PDF document with a dimension of 400×400 points. const page = pdfDoc.addPage([400, 400]); // Embed the image into the PDF document. const imageEmbed = await pdfDoc.embedJpg(image); // Scale the image to fit within the page dimensions while preserving aspect ratio. const { width, height } = imageEmbed.scaleToFit( page.getWidth(), page.getHeight(), ); // Draw the image on the PDF page. page.drawImage(imageEmbed, { x: page.getWidth() / 2 - width / 2, // Center the image horizontally. y: page.getHeight() / 2 - height / 2, // Center the image vertically. width, height, color: rgb(0, 0, 0), // Set the image color to black. }); // Save the PDF document as bytes. const pdfBytes = await pdfDoc.save(); // Write the PDF bytes to a file asynchronously. await fs.promises.writeFile(pdfPath, pdfBytes); } // Call the conversion function with input and output file paths. convertImageToPdf('input.jpg', 'output.pdf') .then(() => { console.log('Image converted to PDF successfully!'); }) .catch((error) => { console.error('Error converting image to PDF:', error); });
-
Replace
'input.jpg'
with the path to the image you want to convert and'output.pdf'
with the desired path for the output PDF. -
Run the conversion script using the following command:
node convert.js
Check your project directory for the generated output.pdf
file.
You’ve successfully converted an image to a PDF using Node.js and the pdf-lib library.
PSPDFKit for Node.js
PSPDFKit for Node.js introduces a suite of capabilities for document conversion within Node.js applications. It empowers developers to seamlessly convert Word, Excel, PowerPoint, and image files into PDFs, all without the need for MS Office or third-party open source dependencies. Furthermore, PSPDFKit for Node.js offers a convenient way to integrate advanced PDF functionality into any Node.js application.
Key Features of PSPDFKit for Node.js
PSPDFKit for Node.js comes with an array of features that make it an excellent choice for document conversion within Node.js applications:
-
Versatile Conversion — PSPDFKit for Node.js supports converting a wide range of file formats, including PDF, Word, Excel, PowerPoint, TIFF, JPG, and PNG. This diverse format support enables seamless document conversion without third-party tools.
-
Custom Font Support — The library intelligently handles custom fonts during conversion, ensuring that documents maintain their visual integrity and consistency, even when converted across formats.
-
Diverse File Support — PSPDFKit for Node.js supports handling various formats beyond images, such as DOCX, XLSX, PPTX, and other popular document formats, making it a comprehensive solution for document conversion.
-
Advanced Rendering — The library offers advanced rendering capabilities, allowing you to render PDF pages into formats like PNG or WebP. This provides flexibility in choosing how to visualize the converted documents.
Comparison with pdf-lib
When comparing PSPDFKit for Node.js with the pdf-lib library, consider the following:
-
Conversion Scope — PSPDFKit for Node.js boasts a broader scope of supported formats, encompassing PDF and various Office formats like Word, Excel, and PowerPoint. In contrast, pdf-lib primarily focuses on PDF creation and manipulation, with image embedding as a specific use case.
-
Font Handling — PSPDFKit for Node.js excels in its intelligent custom font handling during conversion, ensuring consistent visual representation across different formats.
-
Advanced Rendering — PSPDFKit for Node.js provides advanced rendering options, allowing you to render PDF pages into different image formats, a capability that caters to diverse visualization needs.
Integrating PSPDFKit for Node.js
-
Initialize a new Node.js project:
npm init -y
This command creates a package.json
file in your project directory, which is essential for managing your project’s dependencies.
-
Install the PSPDFKit for Node.js package:
npm install @pspdfkit/nodejs
-
Place your image document (e.g. image.png) in your project directory.
-
Create a new JavaScript file named
index.js
in your project directory. This script will take care of the conversion process for you.
const { load } = require('@pspdfkit/nodejs'); const fs = require('fs'); (async () => { const pngImage = fs.readFileSync('image.png'); const instance = await load({ document: pngImage }); const buffer = await instance.exportPDF(); fs.writeFileSync('converted.pdf', Buffer.from(buffer)); instance.close(); })();
This example loads an image and exports it to a PDF. It initiates by reading the image data and proceeds to convert it into a PDF format. The resulting PDF, named converted.pdf
, is then saved within your project directory.
Supported Image Formats | |
---|---|
PNG | ✓ |
JPEG, JPG | ✓ |
TIFF, TIF | ✓ |
![]()
By default, the resulting PDF will include a PSPDFKit watermark. To exclude the watermark, you can specify the
license
property with akey
andappName
when calling theload()
function. For details on obtaining a trial license key, please get in touch with Sales.
-
Execute the script in your terminal to initiate the conversion process:
node index.js
Conclusion
In this post, you explored two approaches to document conversion in Node.js applications. The first part covered using the pdf-lib library to convert an image to PDF. In the second part, you learned about PSPDFKit for Node.js, a robust SDK offering versatile document conversion features.
To get started with PSPDFKit for Node.js, you can either:
-
Start your free trial to test the library and see how it works in your application.
-
Launch our demo to see the viewer in action.