How to Convert HTML to PDF Using html2pdf
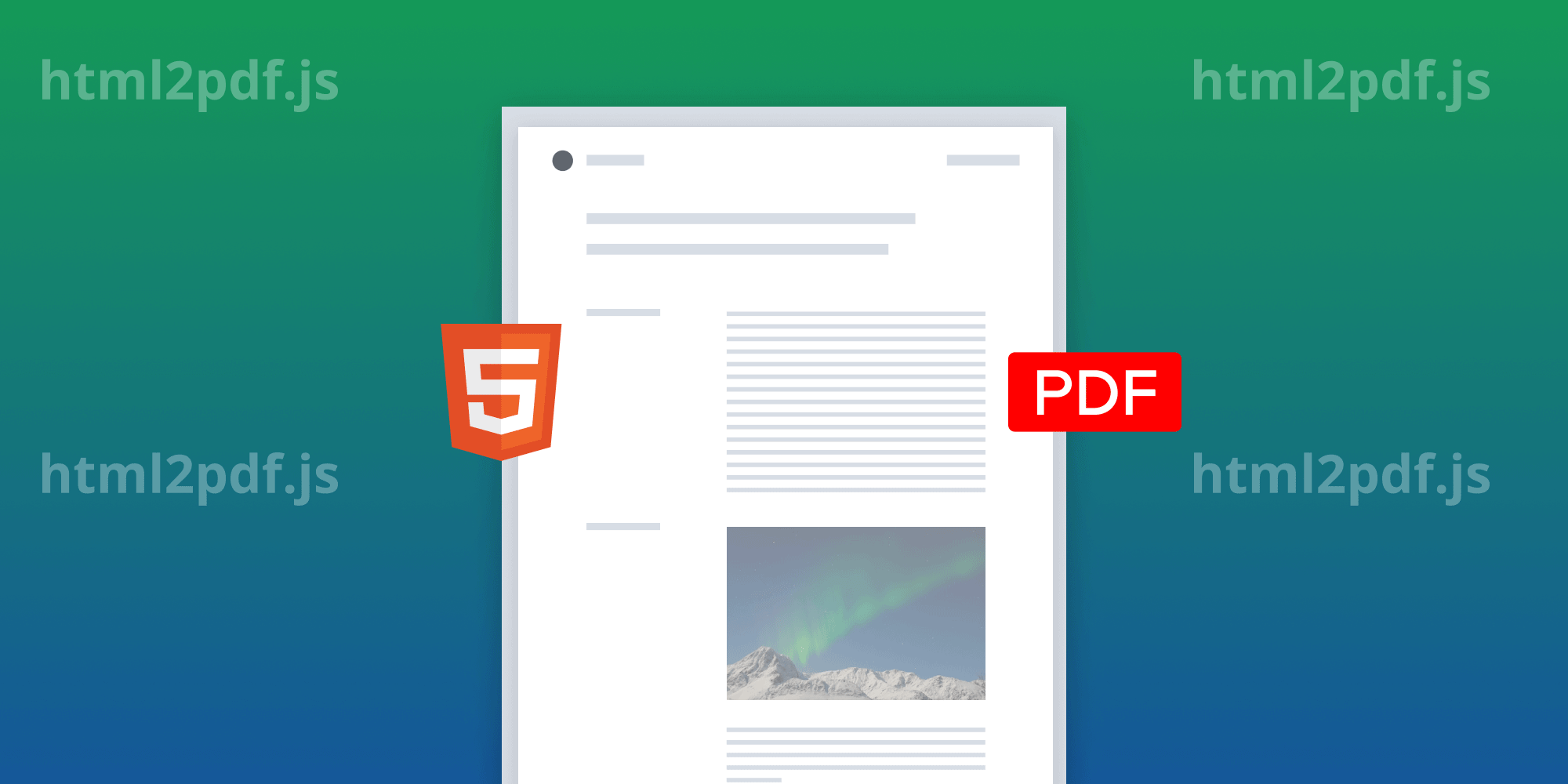
In this post, you’ll learn how to convert HTML to PDF using html2pdf.js. This JavaScript library allows you to perform conversion entirely on the client-side using only HTML, CSS, and JavaScript. This means you don’t need to have any server-side processing or dependencies to convert your HTML to PDF. Instead, the conversion process is done entirely in the browser, making it a fast and efficient way to generate PDFs.
The library uses two main dependencies: html2canvas, which takes screenshots of webpages and converts them to canvas, and jsPDF, which allows you to generate PDFs from the canvas elements created by html2canvas.
html2pdf.js provides an easy-to-use API that lets you specify the element(s) you want to convert to PDF and any additional configuration options you may need.
Getting Started with html2pdf
-
First, you’ll need to include html2pdf.js in your HTML file. You can do this by either downloading the library from the official GitHub repository or including it via a content delivery network (CDN):
<script src="https://cdnjs.cloudflare.com/ajax/libs/html2pdf.js/0.10.1/html2pdf.bundle.min.js" integrity="sha512-GsLlZN/3F2ErC5ifS5QtgpiJtWd43JWSuIgh7mbzZ8zBps+dvLusV+eNQATqgA/HdeKFVgA5v3S/cIrLF7QnIg==" crossorigin="anonymous" referrerpolicy="no-referrer" ></script>
Now that you’ve included the library in your project, follow the next steps to convert your HTML content to PDF.
-
Create the HTML content you want to convert to a PDF file. You can either use an existing HTML element on your webpage or create a new one. For this example, you’ll create a
<div>
containing some text and basic styling:
<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <meta http-equiv="X-UA-Compatible" content="IE=edge" /> <title>HTML to PDF Using html2pdf</title> <meta name="viewport" content="width=device-width, initial-scale=1" /> <!-- html2pdf CDN link --> <script src="https://cdnjs.cloudflare.com/ajax/libs/html2pdf.js/0.10.1/html2pdf.bundle.min.js" integrity="sha512-GsLlZN/3F2ErC5ifS5QtgpiJtWd43JWSuIgh7mbzZ8zBps+dvLusV+eNQATqgA/HdeKFVgA5v3S/cIrLF7QnIg==" crossorigin="anonymous" referrerpolicy="no-referrer" ></script> </head> <body> <div id="content"> <h1>Welcome to html2pdf Tutorial</h1> <p> This is an example of converting HTML content to a PDF file using the html2pdf JavaScript library. </p> </div> <script src="index.js"></script> </body> </html>
-
Create a JavaScript file (e.g.
index.js
). Select the HTML element(s) you want to convert to PDF. This can be done using JavaScript DOM selectors. For example, if you want to convert the entire webpage, you can select thedocument.documentElement
property, which represents the root element of the HTML document:
const element = document.documentElement;
This will select the <html>
element and all of its children, including the <head>
and <body>
elements, to be converted to PDF. If you only want to convert a specific part of the page, you can select a different element using a CSS selector, such as an ID or class name, like this:
const element = document.querySelector('#my-element');
This would select the element with the ID my-element
to be converted to PDF.
-
After selecting your HTML element(s), you can specify the conversion options you want to use. Some of the available options include the file name, paper size, page orientation, margins, and image quality. Here’s an example:
const options = { filename: 'my-document.pdf', margin: 1, image: { type: 'jpeg', quality: 0.98 }, html2canvas: { scale: 2 }, jsPDF: { unit: 'in', format: 'letter', orientation: 'portrait' }, };
In this example, you’re specifying that you want the PDF to be named 'my-document.pdf'
, and you want to use JPEG images with 98 percent quality. You’re also setting some options for html2canvas and jsPDF. Note that you can customize these options to suit your needs.
Here are some of the most commonly used options:
-
pagebreak
— Specifies when to insert page breaks. -
disableLinks
— If set totrue
, disables hyperlinks in the PDF. -
disableCalendars
— If set totrue
, disables date pickers and calendars in the PDF. -
enableJavascript
— If set totrue
, enables JavaScript execution in the HTML before conversion. -
header
— Specifies the content to be added at the top of each page, as a string or a function. -
footer
— Specifies the content to be added at the bottom of each page, as a string or a function.
For example, here’s how you could use the header and footer options to add page numbers to the PDF:
const options = { filename: 'my-document.pdf', header: '<div style="text-align:center;">Page <span class="page"></span> of <span class="total"></span></div>', footer: '<div style="text-align:center;">Generated by my app on ' + new Date().toLocaleDateString() + '</div>', };
In this example, the header option specifies a centered <div>
element containing the text “Page X of Y,” where X is the current page number and Y is the total number of pages. The footer option specifies a centered <div>
element containing the text “Generated by my app on [current date].”
html2pdf.js provides many more configuration options and customization features that you can explore in its documentation.
-
Finally, you can use the
html2pdf()
function to initiate the conversion and save the resulting PDF:
html2pdf().set(options).from(element).save();
In this example, you’re using the set()
method to configure the conversion options, the from()
method to specify the HTML element(s) to convert, and the save()
method to save the PDF file.
-
Open the HTML file in a browser like Google Chrome, Mozilla Firefox, or Microsoft Edge. This will download the PDF file to your computer.
Pros and Cons of Using html2pdf for Converting HTML to PDF
As with anything, there are both pros and cons to this conversion solution.
Pros
-
Easy to use — html2pdf is straightforward library that allows you to convert HTML to PDF using a few lines of JavaScript code.
-
No server-side processing required — Since html2pdf is entirely client-side, you don’t need to rely on any server-side processing or dependencies. This makes it a great option if you don’t have access to server-side resources or want to reduce server load.
-
Customizable options — html2pdf provides many customization options, such as paper size, page orientation, margins, image quality, and more. This allows you to generate PDFs that meet your specific needs.
-
Open source — html2pdf is an open source library, which means you can use it for free and contribute to its development if you wish.
Cons
-
Limited support for advanced PDF features — html2pdf may not support some advanced PDF features, such as embedded fonts, annotations, or form fields. For more complex PDF generation tasks, a server-side library like wkhtmltopdf, or a more powerful client-side library like pdfmake, might be more suitable.
-
Performance issues — Since html2pdf relies on html2canvas to take screenshots of webpages and convert them to canvas, the conversion process can be slow and resource-intensive, especially for large or complex documents.
-
Dependence on external libraries — html2pdf relies on other libraries (html2canvas and jsPDF) for its functionality. While this approach simplifies the code and reduces maintenance, it can also introduce potential compatibility issues or limitations from the underlying libraries.
-
Incomplete support for CSS and JavaScript — html2pdf may not render certain CSS styles or JavaScript-driven content accurately. This limitation can be problematic if your HTML content relies heavily on advanced CSS or dynamic content generated through JavaScript.
Alternatives to html2pdf.js
While html2pdf.js is a popular option for converting HTML to PDF, there are several other libraries and tools available for this purpose. Below are some of the alternatives to consider.
-
wkhtmltopdf — This is a command-line tool that uses the WebKit rendering engine to convert HTML to PDF. It supports advanced features such as headers and footers, tables of contents, and page numbering. However, it requires server-side processing and may not be as customizable as html2pdf.js.
-
Puppeteer — This is a Node.js library that provides a high-level API for controlling headless Chrome or Chromium browsers. It can be used to generate PDFs from HTML content, and it supports advanced features such as page breaks, headers and footers, and tables of contents. However, it requires server-side processing and may not be as lightweight as html2pdf.js.
-
PSPDFKit — We offer a couple of options for converting HTML to PDF:
-
HTML-to-PDF API — A REST API and hosted solution that provides 100 free conversions per month and offers additional packages for a per-document fee. This option allows you to integrate HTML-to-PDF conversion into your applications without the need for self-hosting or a server setup.
-
PDF Generation SDK — This allows you to generate a PDF from scratch, leveraging HTML and CSS technologies for highly flexible designs and context-customized PDFs for your users.
-
PSPDFKit’s solutions are regularly maintained, with releases occurring multiple times throughout the year. We also offer one-on-one support to handle any issues or challenges you encounter, which can be a significant advantage compared to open source solutions.
If you’re interested in PSPDFKit, you can contact our Sales team.
Conclusion
Overall, html2pdf.js is a useful library for generating PDFs from HTML content. It’s entirely client-side, so you don’t need to rely on any server-side processing or dependencies. The library provides many customization options, and it’s easy to use. If you need to generate PDFs from your HTML content, give html2pdf.js a try!