How to Split PDFs Using Java
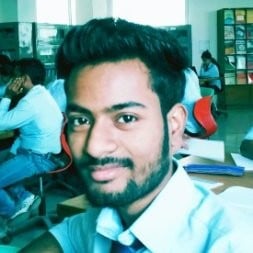
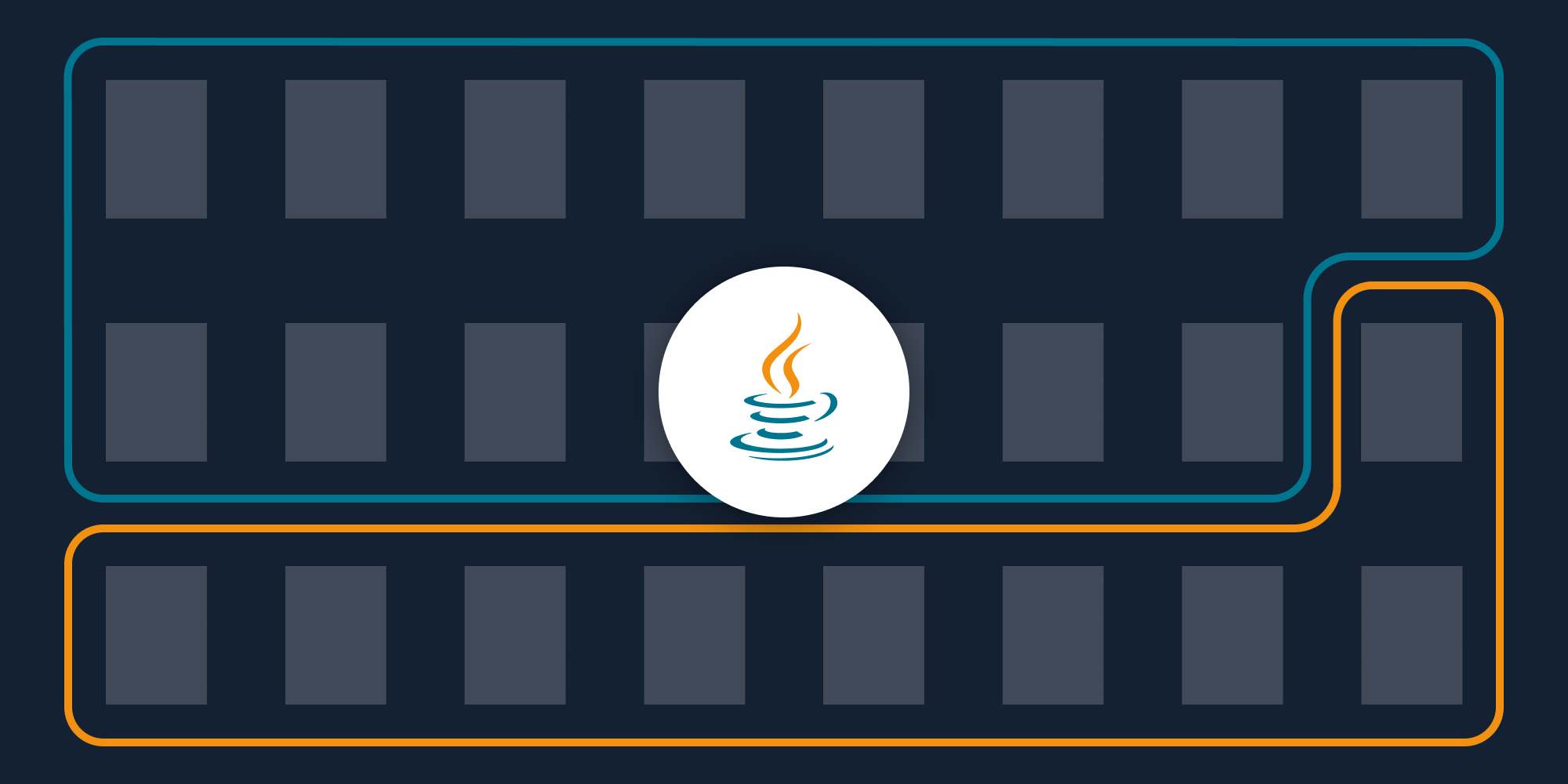
In this post, you’ll learn how to split PDF files using our Split PDF Java API. With this API, you can split up to 100 PDF files per month for free. All you need to do is create a free account to get access to your API key.
Splitting a PDF document is a common use case when working with PDFs and PDF forms because it enables logical archiving of information. Using a PDF splitting API will allow you to automate the process of splitting documents in your workflow.
A simple example would be a financial services company receiving a single PDF with clients’ personal and financial information, as well as a questionnaire they filled in. By integrating a PDF splitting API into the workflow, it’s easy to automatically split these documents into logical parts that can be stored separately.
PSPDFKit API
Document splitting is just one of our 30+ PDF API tools. You can combine our splitting tool with other tools to create complex document processing workflows, such as:
-
Converting MS Office files and images into PDFs and splitting them
-
Performing OCR on documents and splitting them
-
Watermarking and flattening PDFs and splitting them
Step 1 — Creating a Free Account on PSPDFKit
Go to our website, where you’ll see the page below, prompting you to create your free account.
Once you’ve created your account, you’ll be welcomed by the page below, which shows an overview of your plan details.
As you can see in the bottom-left corner, you’ll start with 100 documents to process, and you’ll be able to access all our PDF API tools.
Step 2 — Obtaining the API Key
After you’ve verified your email, you can get your API key from the dashboard. In the menu on the left, click API Keys. You’ll see the following page, which is an overview of your keys:
Copy the Live API Key, because you’ll need this for the Split PDF API.
Step 3 — Setting Up Folders and Files
For this tutorial, you’ll use IntellIJ IDEA as your primary code editor. Now, create a new project called split_pdf
. You can choose any location, but make sure to select Java as the language, Gradle as the build system, and Groovy as the Gradle DSL.
Create a new directory in your project. Right-click on your project’s name and select New > Directory. From there, choose the src/main/java
option. Once done, create a class file inside the src/main/java
folder called processor.java
, and create two folders called input_documents
and processed_documents
in the root folder.
After that, paste your PDF file inside the input_documents
folder.
Your folder structure will look like this:
split_pdf ├── input_documents | └── document.pdf ├── processed_documents ├── src | └── main | └── java | └── processor.java
Step 4 — Installing Dependencies
Next, you’ll install two libraries:
-
OkHttp — This library makes API requests.
-
JSON — This library will parse the JSON payload.
Open the build.gradle
file and add the following dependencies to your project:
dependencies { implementation 'com.squareup.okhttp3:okhttp:4.9.2' implementation 'org.json:json:20210307' }
Once done, click the Add Configuration button. This will open a dropdown menu. Next, select Application:
Now, fill the form with the required details. Most of the fields will be prefilled, but you need to select java 18
in the module field and add -cp split_pdf_4.main
in the main class.
To apply settings, click the Apply button.
Step 5 — Writing the Code
Now, open the processor.java
file and paste the code below into it:
import java.nio.file.FileSystems; import java.io.File; import java.io.IOException; import java.nio.file.Files; import java.nio.file.StandardCopyOption; import org.json.JSONArray; import org.json.JSONObject; import okhttp3.MediaType; import okhttp3.MultipartBody; import okhttp3.OkHttpClient; import okhttp3.Request; import okhttp3.RequestBody; import okhttp3.Response; public final class Processor { public static void main(final String[] args) throws IOException { final RequestBody firstHalfBody = new MultipartBody.Builder() .setType(MultipartBody.FORM) .addFormDataPart( "document", "document.pdf", RequestBody.create( new File("input_documents/document.pdf"), MediaType.parse("application/pdf") ) ) .addFormDataPart( "instructions", new JSONObject() .put("parts", new JSONArray() .put(new JSONObject() .put("file", "document") .put("pages", new JSONObject() .put("end", -6) ) ) ).toString() ) .build(); final Request firstHalfRequest = new Request.Builder() .url("https://api.pspdfkit.com/build") .method("POST", firstHalfBody) .addHeader("Authorization", "Bearer YOUR API KEY HERE") .build(); final RequestBody secondHalfBody = new MultipartBody.Builder() .setType(MultipartBody.FORM) .addFormDataPart( "document", "document.pdf", RequestBody.create( new File("input_documents/document.pdf"), MediaType.parse("application/pdf") ) ) .addFormDataPart( "instructions", new JSONObject() .put("parts", new JSONArray() .put(new JSONObject() .put("file", "document") .put("pages", new JSONObject() .put("start", -5) ) ) ).toString() ) .build(); final Request secondHalfRequest = new Request.Builder() .url("https://api.pspdfkit.com/build") .method("POST", secondHalfBody) .addHeader("Authorization", "Bearer YOUR API KEY HERE") .build(); executeRequest(firstHalfRequest, "processed_documents/first_half.pdf"); executeRequest(secondHalfRequest, "processed_documents/second_half.pdf"); } private static void executeRequest(final Request request, final String outputFileName) throws IOException { final OkHttpClient client = new OkHttpClient() .newBuilder() .build(); final Response response = client.newCall(request).execute(); if (response.isSuccessful()) { Files.copy( response.body().byteStream(), FileSystems.getDefault().getPath(outputFileName), StandardCopyOption.REPLACE_EXISTING ); } else { // Handle the error. throw new IOException(response.body().string()); } } }
Code Explanation
In the code above, you’re importing all the packages required to run the code and creating a class called processor
. In the main function, you’re first creating the request body for the API call that contains all the instructions for splitting the PDF. Then, you’re calling the API to process the instructions.
Finally, you’re calling the executeRequest
function and passing both form data variables: firstHalfRequest
and secondHalfRequest
. The response of the API is then stored in the processed_documents
folder.
Output
To execute the code, click the Run button (which is a little green arrow). This is next to the field that says Processor, which is where you set the configuration.
On successful execution, it’ll create two files in the processed_documents
folder: first_half.pdf
and second_half.pdf
. The folder structure will look like this:
split_pdf ├── input_documents | └── document.pdf ├── processed_documents | └── first_half.pdf | └── second_half.pdf ├── src | └── main | └── java | └── processor.java
Final Words
In this post, you learned how to easily and seamlessly split PDF files for your Java application using our Split PDF API.
You can integrate these functions into your existing applications and split PDFs. With the same API token, you can also perform other operations, such as merging documents into a single PDF, adding watermarks, and more. To get started with a free trial, sign up here.