How to Merge PDFs Using JavaScript
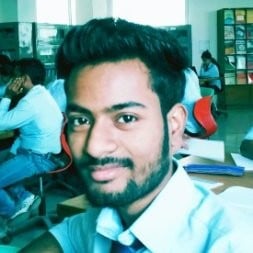
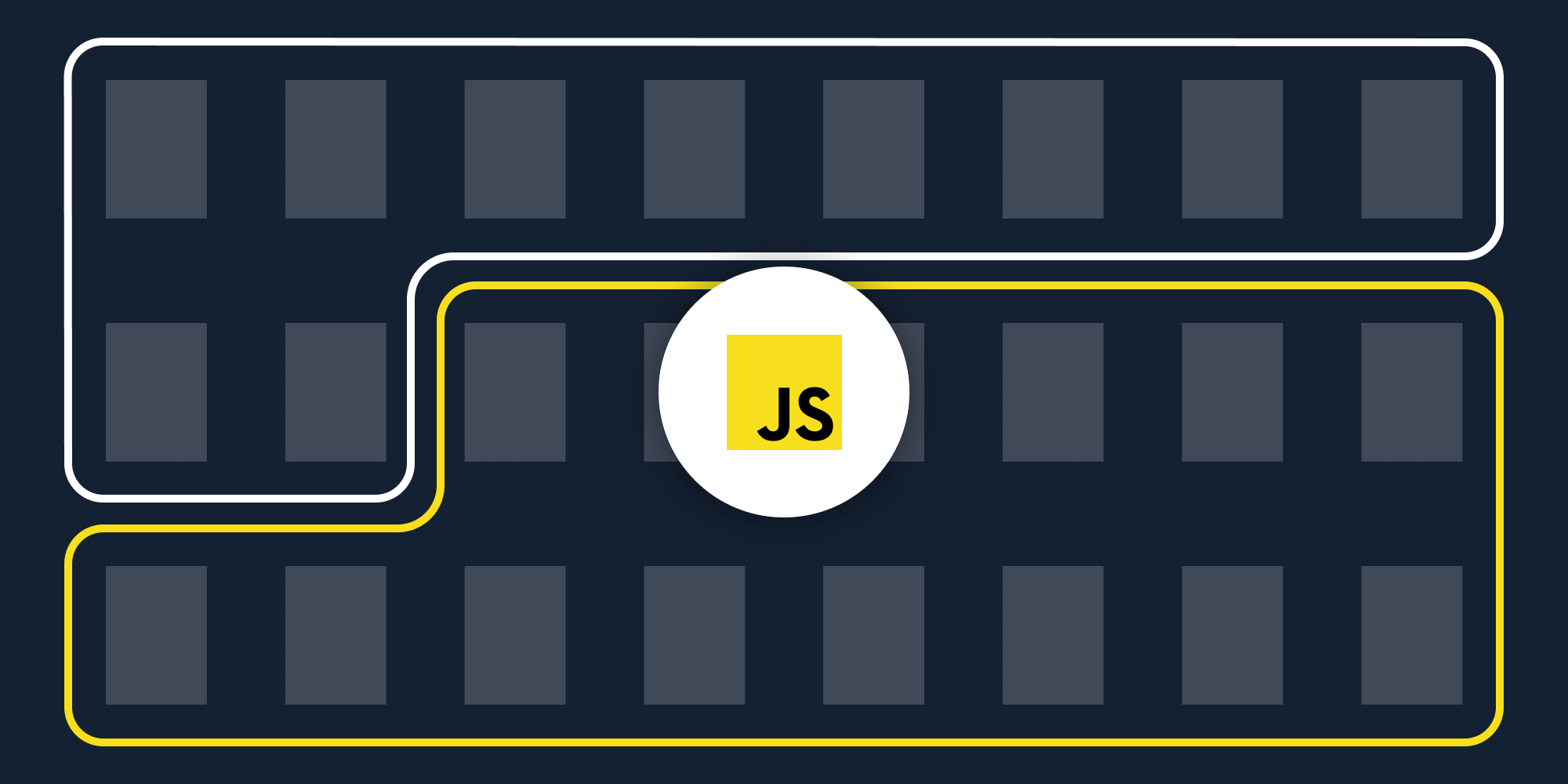
In this post, you’ll learn how to combine multiple PDF files using our Merge PDF JavaScript API. With our API, you can merge multiple PDF files up to 100 times per month for free. You’ll just need to create a free account to get access to your API key.
This post will be especially helpful for developers working with JavaScript in document-heavy workflows where users upload a large number of documents. This API will enable you to automate merging documents in your workflows.
A simple example could be an HR application where users upload resumes, cover letters, and references. By integrating a PDF merging API into the workflow, you’ll be able to automatically merge these documents and provide a consolidated document to your end users.
PSPDFKit API
Document merging is just one of our 30+ PDF API tools. You can combine our merging tool with other tools to create complex document processing workflows, such as:
-
Converting MS Office files and images into PDFs before merging
-
Performing OCR on several documents before merging
-
Merging, watermarking, and flattening PDFs
Once you create your account, you’ll be able to access all our PDF API tools.
Step 1 — Creating a Free Account on PSPDFKit
Go to our website, where you’ll see the page below, prompting you to create your free account.
Once you’ve created your account, you’ll be welcomed by the page below, which shows an overview of your plan details.
As you can see in the bottom-left corner, you’ll start with 100 documents to process, and you’ll be able to access all our PDF API tools.
Step 2 — Obtaining the API Key
After you’ve verified your email, you can get your API key from the dashboard. In the menu on the left, click API Keys. You’ll see the following page, which is an overview of your keys:
Copy the Live API Key, because you’ll need this for the Merge PDF API.
Step 3 — Setting Up Files and Folders
Now, create a folder called merge_pdf
and open it in a code editor. For this tutorial, you’ll use VS Code as your primary code editor. Next, create two folders inside merge_pdf
and name them input_documents
and processed_documents
.
Then, in the root folder, merge_pdf
, create a file called processor.js
. This is where you’ll keep your code.
Step 4 — Installing Dependencies
To get started merging PDF pages, you first need to install the following dependencies:
-
axios — This package is used for making REST API calls.
-
Form-Data — This package is used for creating form data.
Use the commands below to install both of them:
npm install form-data
npm install axios
Step 5 — Writing the Code:
Now, open the processor.js
file and paste the code below into it:
const axios = require('axios') const FormData = require('form-data') const fs = require('fs') const formData = new FormData() formData.append('instructions', JSON.stringify({ parts: [ { file: "first_half" }, { file: "second_half" } ] })) formData.append('first_half', fs.createReadStream('input_documents/first_half.pdf')) formData.append('second_half', fs.createReadStream('input_documents/second_half.pdf')) ;(async () => { try { const response = await axios.post('https://api.pspdfkit.com/build', formData, { headers: formData.getHeaders({ 'Authorization': 'Bearer YOUR_API_KEY' // Replace `YOUR_API_KEY` with your API key. }), responseType: "stream" }) response.data.pipe(fs.createWriteStream("processed_documents/node_result.pdf")) } catch (e) { const errorString = await streamToString(e.response.data) console.log(errorString) } })() function streamToString(stream) { const chunks = [] return new Promise((resolve, reject) => { stream.on("data", (chunk) => chunks.push(Buffer.from(chunk))) stream.on("error", (err) => reject(err)) stream.on("end", () => resolve(Buffer.concat(chunks).toString("utf8"))) }) }
ℹ️ Note: Make sure to replace
YOUR_API_KEY
with your API key.
Code Explanation
You first imported all the packages needed to make the API call, and then you prepared the FormData
. After that, you appended the first_half
and second_half
by reading the first_half.pdf
and second_half.pdf
files.
You then made an asynchronous API call to the Merge PDF API and stored the result in processed_documents
.
Step 6 — Output
To run the code, use the command below:
node processor.js
On the successful execution of the code, you’ll see a new processed file named node_result.pdf
in the processed_documents
folder.
The folder structure will look like this:
merge_pdf ├── input_documents | └── first_half.pdf | └── second_half.pdf ├── node_modules ├── processed_documents | └── node_result.pdf
You can now easily integrate this function into your other JavaScript modules and merge different documents.
Final Words
In this post, you learned how to easily and seamlessly merge files for your JavaScript application into a single PDF using our Merge PDF API.
If you have a more complex use case, you can use our other tools to add watermarks, perform OCR, and edit (split, flatten, delete, duplicate) documents — and you can even combine these tools. To get started with a free trial, sign up here.