Generate a PDF from HTML with Vue.js
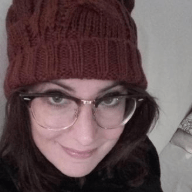
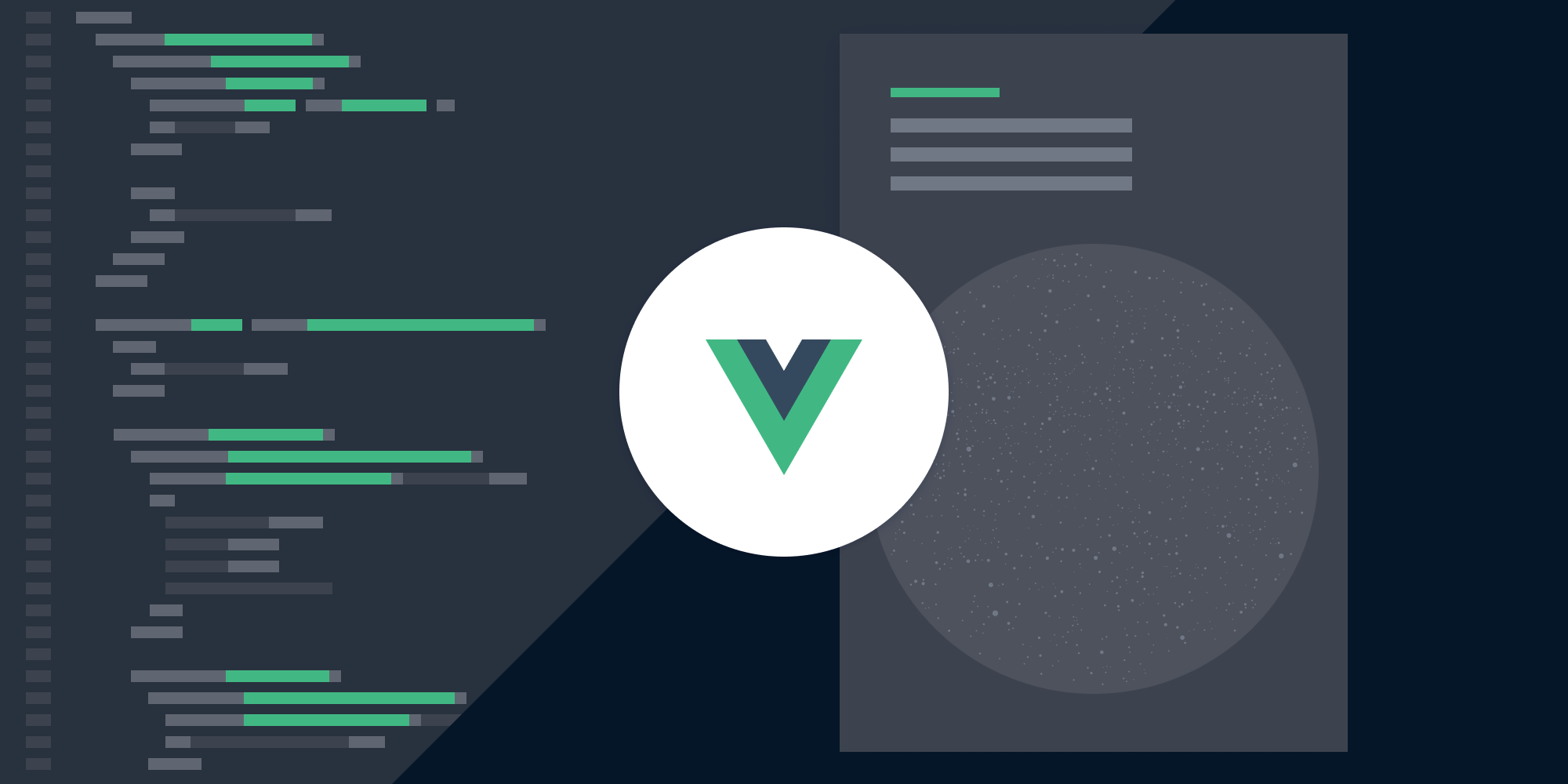
![]()
This article was first published in July 2022 and was updated in June 2024.
The task of converting HTML to PDF isn’t a trivial one, and it takes a considerable amount of code. Thankfully, nowadays, there are many libraries that can help us achieve this goal with a few simple lines. When researching said libraries, many people stumble upon html2pdf.js. In this blog post, you’ll use it in combination with Vue.js to generate a PDF from HTML.
If you prefer a video tutorial, you can watch our step-by-step guide.
Prerequisites
This post assumes you’re familiar with Vue.js. If you aren’t, first read through the Vue.js guides.
Start by creating a boilerplate Vue.js project using npm create vue@latest
. This command will install and launch create-vue
, the official tool for setting up Vue projects. You’ll be prompted to select various optional features, such as TypeScript and testing support. Refer to the list below for guidance.
-
Project name: …
<your-project-name>
-
Add TypeScript? … No / Yes — Select
No
. -
Add JSX Support? … No / Yes — Select
Yes
. -
Add Vue Router for Single Page Application development? … No / Yes — Select
No
. -
Add Pinia for state management? … No / Yes — Select
No
. -
Add Vitest for Unit testing? … No / Yes — Select
No
. -
Add an End-to-End Testing Solution? … No / Cypress / Nightwatch / Playwright — Select
No
. -
Add ESLint for code quality? … No / Yes — Select
No
. -
Add Prettier for code formatting? … No / Yes — Select
Yes
. -
Add Vue DevTools 7 extension for debugging? (experimental) … No / Yes — Select
No
.
Once the project is set up, install html2pdf.js
using npm i html2pdf.js
. The creator of html2pdf.js
suggests using v0.9.3. We used the latest version and didn’t have a problem, but if you encounter problems in your project, downgrading might be the answer.
Generating a PDF from HTML with html2pdf.js
This post shows how to convert an img
element to PDF. Add the correct URL or file path to the string placeholder used in the src
tag of the img
element.
You’ll start with an App.vue
file. Delete its content and add:
<template> <div id="app" ref="document"> <div id="element-to-convert"> <center> <img width="400" src="./path-or-url" /> </center> </div> </div> <button @click="exportToPDF">Export to PDF</button> </template> <style> #app { margin-top: 60px; } </style>
It will look like what’s shown below.
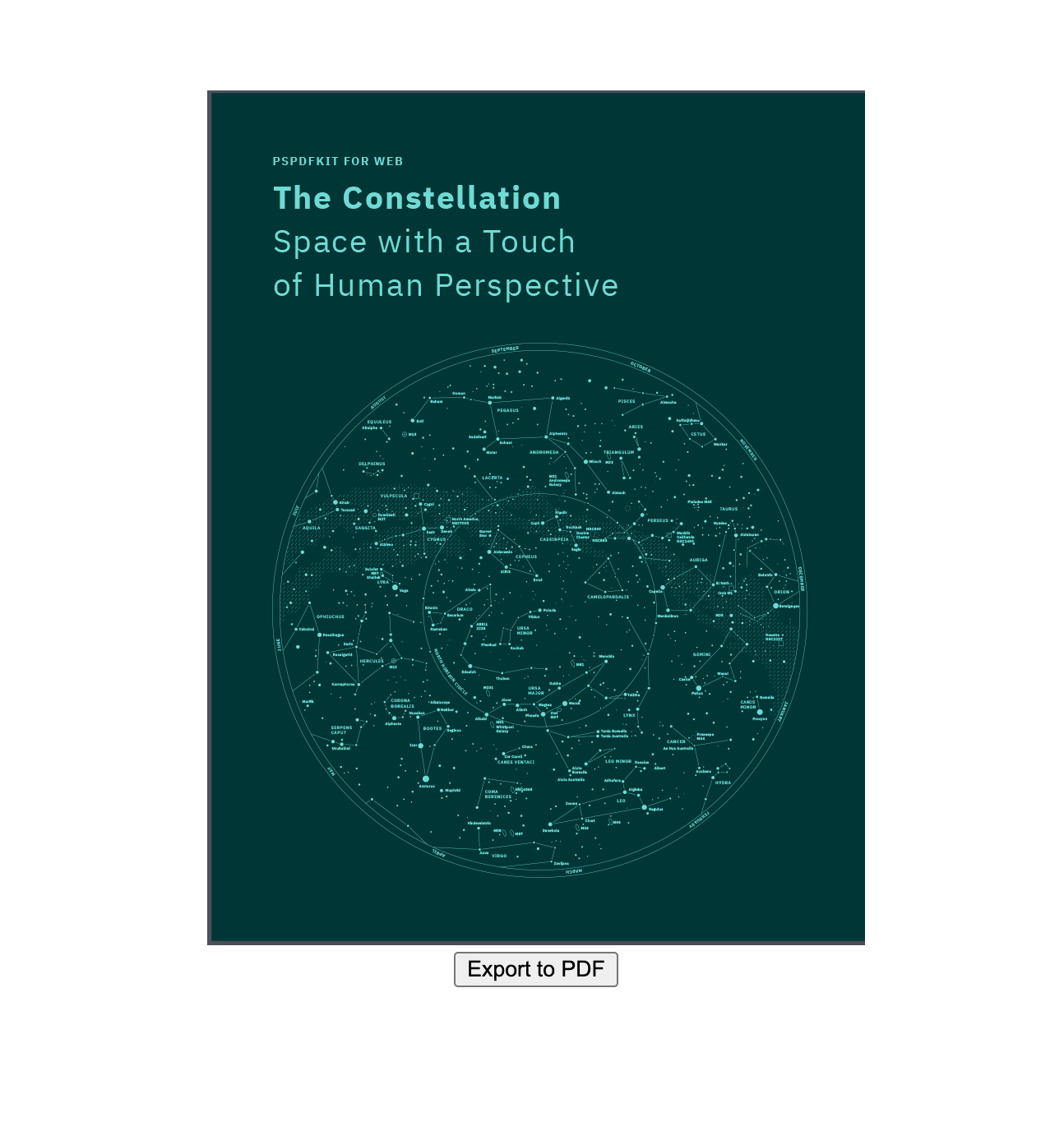
To generate a PDF, you only have to add a few lines of code to your project:
export default { name: 'app', methods: { exportToPDF() { html2pdf(document.getElementById('element-to-convert')); }, }, };
The exportToPDF
function takes a second parameter, an object, which allows you to configure some options that control how the PDF will look. The available options are margin
, filename
, pagebreak
, image
, enableLinks
, html2canvas
, and jsPDF
. You can read more about them here.
For this example, you’ll use a couple of them:
{ margin: 1, filename: "i-was-html.pdf", }
The Full Solution
Here’s how the App.vuejs
file will look after your changes:
<template> <div id="app" ref="document"> <div id="element-to-convert"> <center> <img width="400" src="./path-or-url" /> </center> </div> <button @click="exportToPDF">Export to PDF</button> </div> </template> <script> import html2pdf from "html2pdf.js"; export default { name: "app", methods: { exportToPDF() { html2pdf(document.getElementById("element-to-convert"), { margin: 1, filename: "i-was-html.pdf", }); }, }, }; </script> <style> #app { margin-top: 60px; text-align: center; } </style>
The result is a website with an image and an Export to PDF button that converts the image to PDF when pressed.
Conclusion
html2pdf.js enables us to generate PDFs from HTML quickly and seamlessly. It’s a great choice for building simple applications that generate PDF documents. In this post, you saw how it’s possible to achieve this goal with a few lines of code.
If you have — or predict having — more complex workflows and needs, PSPDFKit can help. For example, if you’re interested in automating the PDF generation process, refer to our PDF Generator API, which enables you to generate PDF files from HTML templates. You can style the CSS, add unique images and fonts, and persist your headers and footers across multiple pages.
If you’re also interested in displaying PDFs in a powerful and customizable viewer that provides a number of advanced functionalities like annotating PDFs or digitally signing them, we recommend checking out Document Engine.
For more information on converting and generating PDFs, check out our related blog posts: