How to Create a React Signature Pad
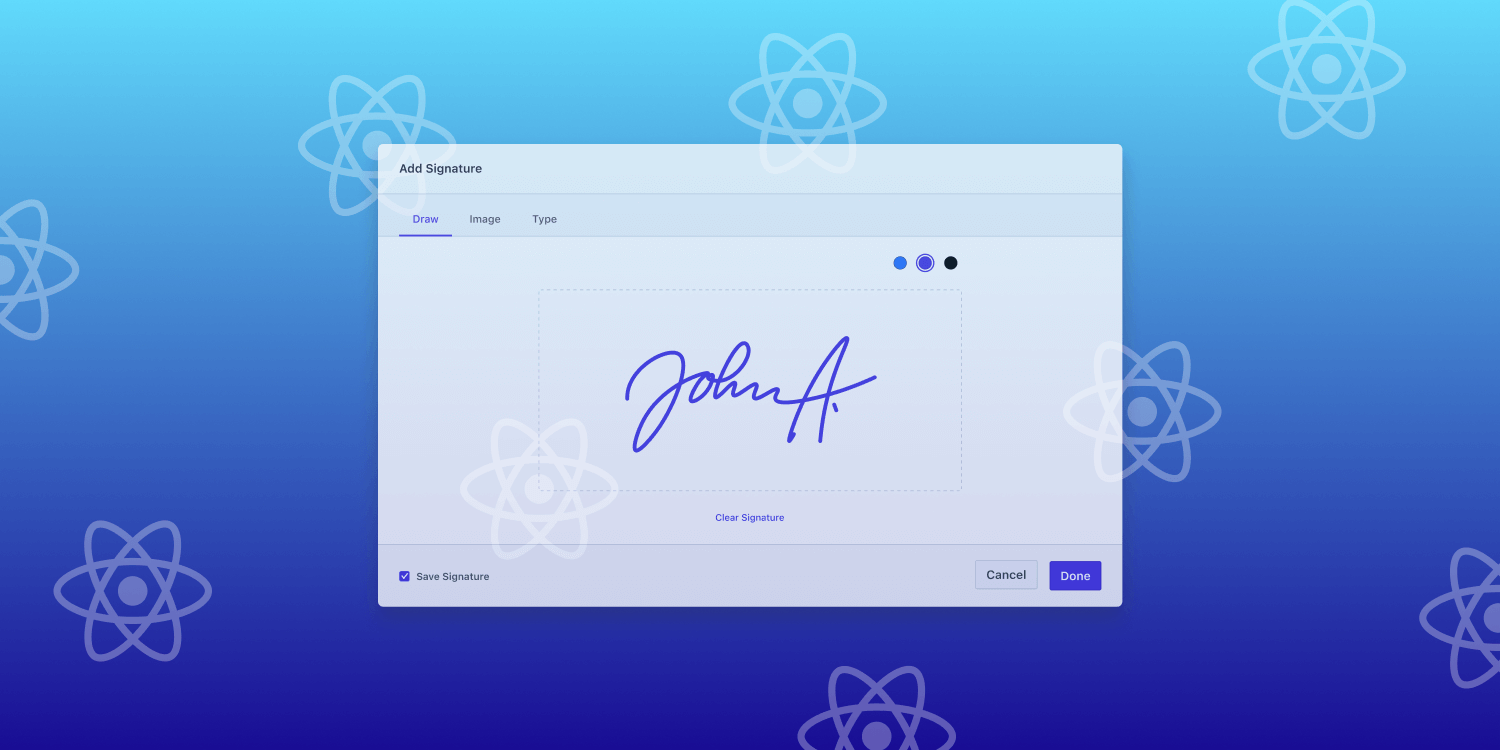
In this post, you’ll learn how to create a signature pad for your React.js web application by using the PSPDFKit library. You’ll first create a React.js project using Create React App, and then you’ll install the PSPDFKit library to add a signature pad to PDF documents.
What Is a React.js Signature Pad?
A signature pad is a graphical interface that can be inserted into documents your users need to sign. It allows them to draw their signatures on a document using their mouse, electronic pens, or dedicated signing devices. It also enables saving signatures as images and vice versa.
Signature pads are used in web applications that need an applicant’s handwritten signature, such as when:
-
Adding signatures to ID cards or official documents
-
Signing forms, contracts, or other financial and legal documentation
-
Signing bills, receipts, and invoices
Why Build a React.js Signature Pad?
The conventional way of signing documents — using pen and paper — can be inefficient. This is especially true if you have to sign PDF documents and the recipient isn’t physically present; you then have to print, sign, and scan the document before sending it to the receiver.
Replacing signed paper documentation with an electronic signature process by adding a signature pad to your PDFs allows organizations to operate more efficiently, and it can help them save time, resources, and money.
PSPDFKit facilitates paperless signing and provides an easier way to create, edit, view, annotate, and sign PDF documents in your React.js applications. It’s also highly customizable: PSPDFKit allows you to fully modify the layout of your PDF software application based on your needs and preferences.
PSPDFKit React.js Library
We offer a commercial React.js PDF library that can easily be integrated into your web application. It comes with 30+ features that let you view, annotate, edit, and sign documents directly in your browser. Out of the box, it has a polished and flexible UI that you can extend or simplify based on your unique use case.
- A prebuilt and polished UI
- 15+ annotation tools
- Support for multiple file types
- Dedicated support from engineers
PSPDFKit has developer-friendly documentation and offers a beautiful user interface for users to edit PDF files easily. Web applications such as Autodesk, Disney, DocuSign, Dropbox, IBM, and Lufthansa use the PSPDFKit library to edit PDF documents. If you’re looking for software that enables you to create and edit PDF files effectively, PSPDFKit is an excellent choice.
Requirements
-
Node.js installed on your computer.
-
A code editor of your choice.
-
A package manager compatible with npm.
Creating a New React Project
-
Create a new React app using the Create React App tool:
yarn create react-app pspdfkit-react-example
npx create-react-app pspdfkit-react-example
-
Change to the created project directory:
cd pspdfkit-react-example
Adding PSPDFKit to Your Project
-
Add the PSPDFKit dependency:
yarn add pspdfkit
npm install pspdfkit
-
Copy the PSPDFKit for Web library assets to the
public
directory:
cp -R ./node_modules/pspdfkit/dist/pspdfkit-lib public/pspdfkit-lib
The above code will copy the pspdfkit-lib
directory from within node_modules/
to the public/
directory to make it available to the SDK at runtime.
-
Make sure your
public
directory contains apspdfkit-lib
directory with the PSPDFKit library assets.
Adding e-Signatures via the PSPDFKit UI
PSPDFKit provides a user-friendly interface that allows users to annotate, edit, and sign PDF documents. In this section, you’ll learn how to sign PDF documents using the PSPDFKit user interface.
-
In the PSPDFKit web interface, click the icon with the sign symbol.
-
Sign on the dialogue box that appears. You can change the color of the pen, and PSPDFKit also allows you to draw your signature and insert an image or text as your signature.
-
You can then resize and change the position of the signature.
Next, we’ll show you how to sign documents programmatically in React.js.
Adding eSignatures Programmatically in React.js
PSPDFKit allows you to add two different kinds of signatures to PDF documents: ink and image signatures. This next part will guide you through creating these signatures and how to add a signature field to a PDF document.
Adding Ink Signatures in a React.js Web Application
Ink signatures are similar to signatures done using a pen on paper. In this use case, PSPDFKit provides a signature pad where you can draw your signature.
Open the ViewerComponent.js
file and update the PSPDFKit.load()
function:
await PSPDFKit.load({ // Container where PSPDFKit should be mounted. container, // The document to open. document: props.document, // Use the public directory URL as a base URL. PSPDFKit will download its library assets from here. baseUrl: `${window.location.protocol}//${window.location.host}/${process.env.PUBLIC_URL}`, }).then(function (instance) { const annotation = new PSPDFKit.Annotations.InkAnnotation({ pageIndex: 0, isSignature: true, lines: PSPDFKit.Immutable.List([ PSPDFKit.Immutable.List([ new PSPDFKit.Geometry.DrawingPoint({ x: 5, y: 5 }), new PSPDFKit.Geometry.DrawingPoint({ x: 95, y: 95 }), ]), PSPDFKit.Immutable.List([ new PSPDFKit.Geometry.DrawingPoint({ x: 95, y: 5 }), new PSPDFKit.Geometry.DrawingPoint({ x: 5, y: 95 }), ]), ]), boundingBox: new PSPDFKit.Geometry.Rect({ left: 0, top: 0, width: 100, height: 100, }), }); instance.create(annotation).then(function (createdAnnotations) { console.log(createdAnnotations); }); });
In the code above:
-
The
PSPDFKit.load()
function creates an instance of PSPDFKit. -
PSPDFKit.Annotations.InkAnnotation
creates freehand drawings, and theisSignature
property must betrue
. -
The
lines
andboundingBox
should be left unchanged. -
PSPDFKit.Geometry.DrawingPoint
inserts ink signatures, and theboundingBox
determines the position and size of the ink signature.
Adding Image Signatures in a React.js Web Application
In this section, you’ll learn how to add images as signatures on PDF documents using PSPDFKit in a React.js web application.
Open the ViewerComponent.js
file and update the PSPDFKit.load()
function:
await PSPDFKit.load({ // Container where PSPDFKit should be mounted. container, // The document to open. document: props.document, // Use the public directory URL as a base URL. PSPDFKit will download its library assets from here. baseUrl: `${window.location.protocol}//${window.location.host}/${process.env.PUBLIC_URL}`, }).then(async function (instance) { // Fetches the image via its URL. const request = await fetch('<image_url>'); // Converts the image to a blob. const blob = await request.blob(); const imageAttachmentId = await instance.createAttachment(blob); const annotation = new PSPDFKit.Annotations.ImageAnnotation({ pageIndex: 0, isSignature: true, contentType: 'image/jpeg', imageAttachmentId, description: 'Image Description', boundingBox: new PSPDFKit.Geometry.Rect({ left: 30, top: 20, width: 300, height: 150, }), }); instance.create(annotation); });
In the code snippet above:
-
The PSPDFKit instance creates an image annotation that enables users to fetch images into the PDF file using a URL.
Creating Signature Fields in PDF Documents
PSPDFKit allows you to create signature fields in PDF documents that enable you to add signatures to PDF documents.
Open the ViewerComponent.js
file and update the PSPDFKit.load()
function:
await PSPDFKit.load({ // Container where PSPDFKit should be mounted. container, // The document to open. document: props.document, // Use the public directory URL as a base URL. PSPDFKit will download its library assets from here. baseUrl: `${window.location.protocol}//${window.location.host}/${process.env.PUBLIC_URL}`, }).then(async function (instance) { const widget = new PSPDFKit.Annotations.WidgetAnnotation({ pageIndex: 0, boundingBox: new PSPDFKit.Geometry.Rect({ left: 200, top: 200, width: 250, height: 150, }), formFieldName: 'My signature form field', id: PSPDFKit.generateInstantId(), }); const formField = new PSPDFKit.FormFields.SignatureFormField({ name: 'My signature form field', annotationIds: PSPDFKit.Immutable.List([widget.id]), }); instance.create([widget, formField]); });
In the code snippet above:
-
You’re creating a new signature field and enabling users to sign within that signature field.
![]()
Interact with the sandbox by clicking the left rectangle icon and selecting Editor > Show Default Layout. To edit, sign in with GitHub — click the rectangle icon again and choose Sign in. To preview the result, click the rectangle icon once more and choose Editor > Embed Preview. For the full example, click the Open Editor button. Enjoy experimenting with the project!
Conclusion
In this post, you learned how to create a React.js signature pad using PSPDFKit’s React.js library. If you hit any snags, don’t hesitate to reach out to our support team for help.
If you’d like to test the PSPDFKit for Web SDK, you can request a free trial. Alternatively, you can browse our demo page to see what our API is capable of.