How to Convert PDF to JPG Using Python
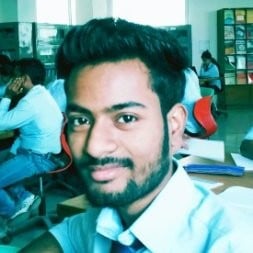
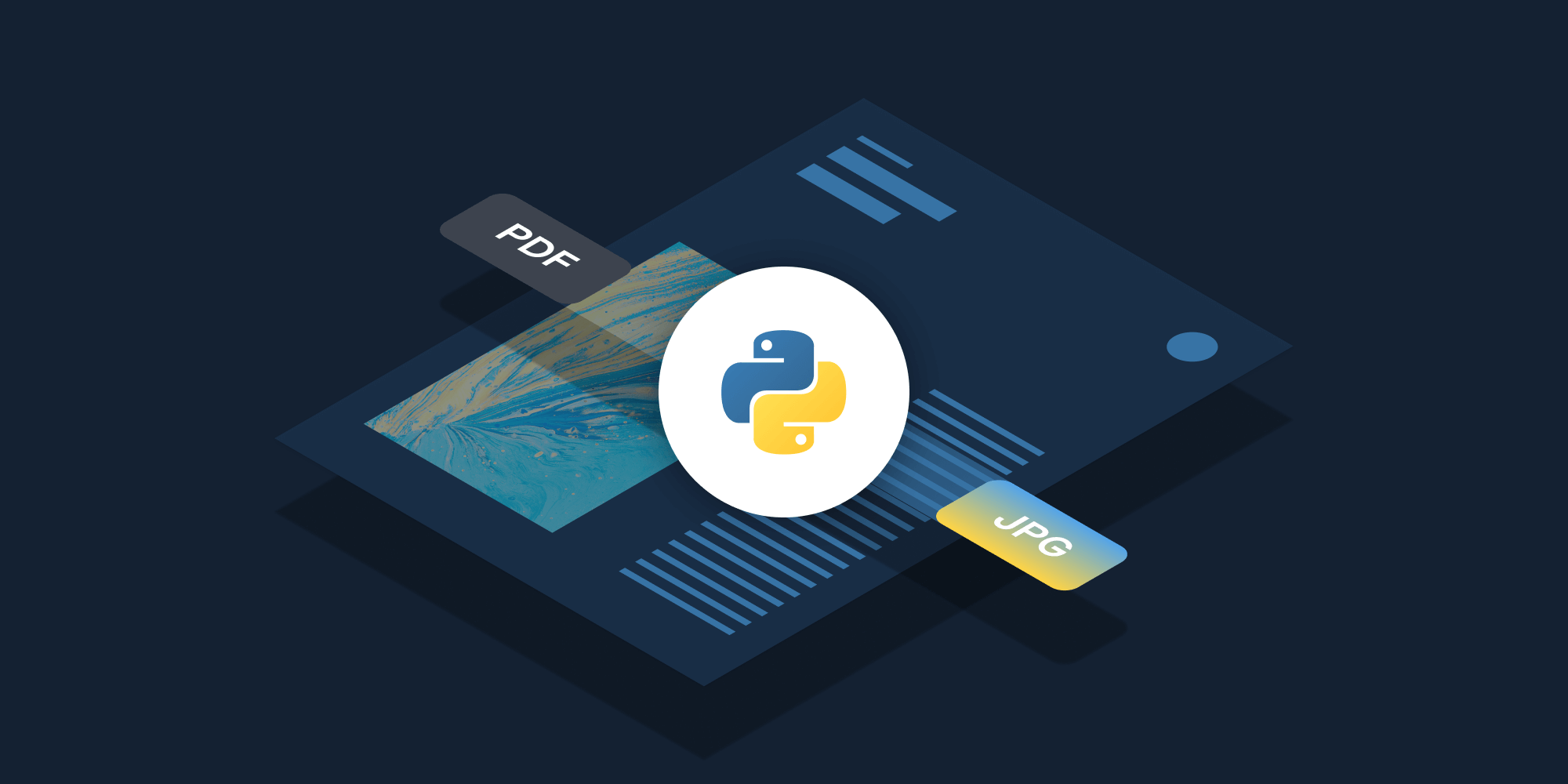
In this post, you’ll learn how to convert PDF files to JPG images using PSPDFKit’s PDF to JPG Python API. With our API, you can convert up to 100 files per month for free. All you need to do is create a free account to get access to your API key.
PSPDFKit API
Document conversion is just one of our 30+ PDF API tools. You can combine our conversion tool with other tools to create complex document processing workflows. With PSPDFKit API, you’ll be able to convert PDFs to image formats and vice versa. You can also integrate the ability to convert Office files, HTML files, and various other file formats to PDFs and images into your application.
Additionally, you can use the API to perform operations on PDFs before converting them into images, such as:
-
Merging several PDFs into one
-
Editing, watermarking, and flattening PDFs
-
Removing or duplicating specific PDF pages
Once you create your account, you’ll be able to access all our PDF API tools.
Step 1 — Creating a Free Account on PSPDFKit
Go to our website, where you’ll see the page below, prompting you to create your free account.
Once you’ve created your account, you’ll be welcomed by the page below, which shows an overview of your plan details.
As you can see in the bottom-left corner, you’ll start with 100 documents to process, and you’ll be able to access all our PDF API tools.
Step 2 — Obtaining the API Key
After you’ve verified your email, you can get your API key from the dashboard. In the menu on the left, click API Keys. You’ll see the following page, which is an overview of your keys:
Copy the Live API Key, because you’ll need this for the PDF to JPG API.
Step 3 — Setting Up Folders and Files
Now, create a folder called pdf_to_jpg
and open it in a code editor. For this tutorial, you’ll use VS Code as your primary code editor. Next, create two folders inside pdf_to_jpg
and name them input_documents
and processed_documents
.
Now, copy your PDF file to the input_documents
folder and rename it to document.pdf
. You can use our demo document as an example.
Then, in the root folder, pdf_to_jpg
, create a file called processor.py
. This is the file where you’ll keep your code.
Your folder structure will look like this:
pdf_to_jpg ├── input_documents | └── document.pdf ├── processed_documents └── processor.py
Step 4 — Writing the Code
Open the processor.py
file and paste the code below into it:
import requests import json instructions = { 'parts': [ { 'file': 'document' } ], 'output': { 'type': 'image', 'format': 'jpg', 'dpi': 500 } } response = requests.request( 'POST', 'https://api.pspdfkit.com/build', headers = { 'Authorization': 'Bearer YOUR API KEY HERE' }, files = { 'document': open('input_documents/document.pdf', 'rb') }, data = { 'instructions': json.dumps(instructions) }, stream = True ) if response.ok: with open('processed_documents/image.jpg', 'wb') as fd: for chunk in response.iter_content(chunk_size=8096): fd.write(chunk) else: print(response.text) exit()
ℹ️ Note: Make sure to replace
YOUR_API_KEY_HERE
with your API key.
Code Explanation
In the code above, you first import the requests
and json
dependencies. After that, you create the instructions for the API call.
You then use the requests
module to make the API call, and once it succeeds, you store the result in the processed_documents
folder.
Output
To execute the code, run the command below:
python3 processor.py
On successful execution, you’ll see a new processed file, image.jpg
, located in the processed_documents
folder.
The folder structure will look like this:
pdf_to_jpg ├── input_documents | └── document.pdf ├── processed_documents | └── image.jpg └── processor.py
Final Words
In this post, you learned how to easily and seamlessly convert PDF files to JPG images for your Python application using our PDF to JPG Python API.
You can integrate all of these functions into your existing applications. With the same API token, you can also perform other operations, such as merging several documents into a single PDF, adding watermarks, and more. To get started with a free trial, sign up here.