How to Convert HTML to PDF in React Native
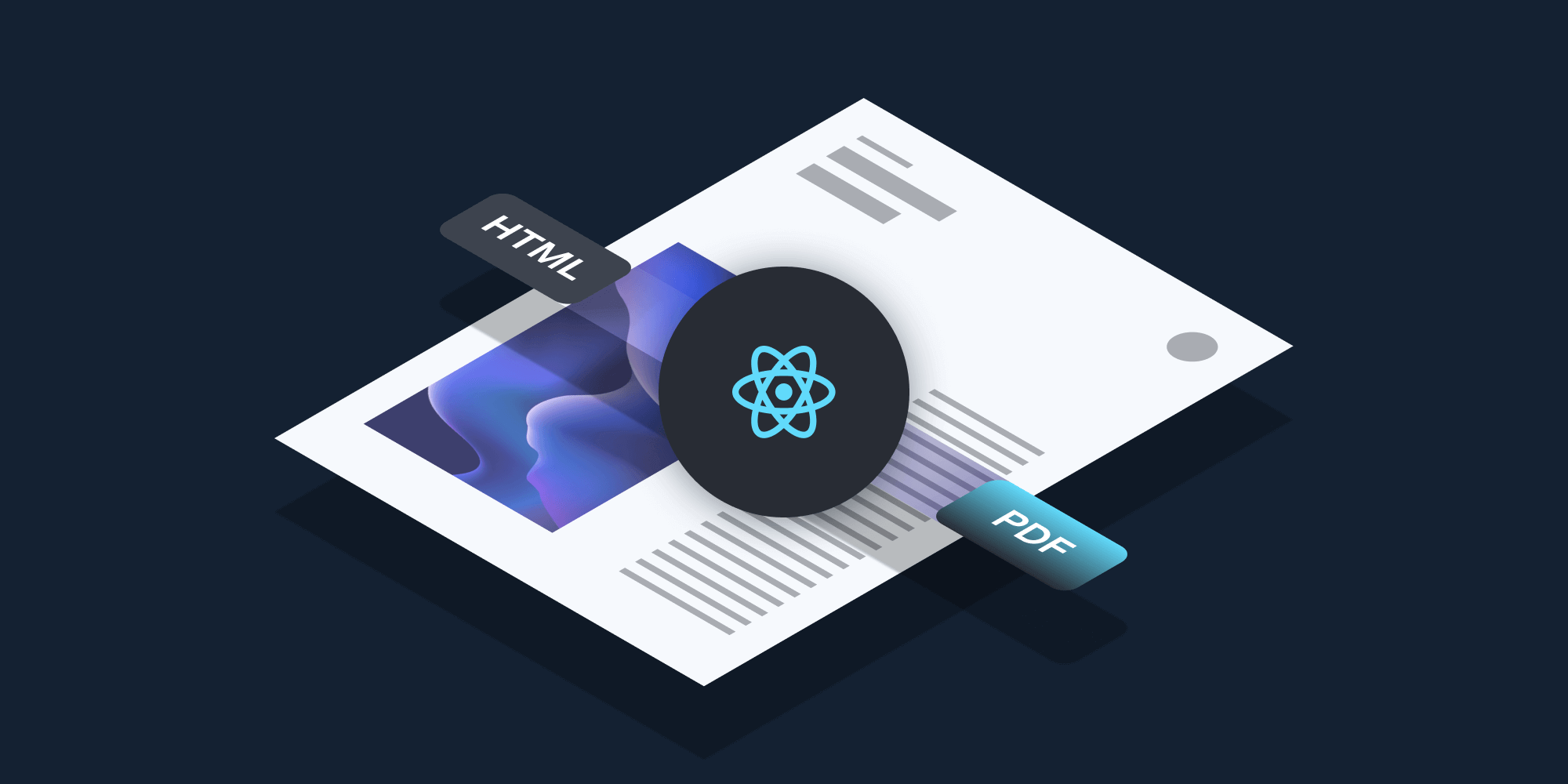
In this post, you’ll learn how to use React Native to convert HTML to PDF. React Native is a mobile framework that can be integrated into existing iOS and Android projects, or you can use it to build one from scratch. It’s based on the popular JavaScript library ReactJS.
The main selling point of React Native is that it lets you create native apps without compromising on performance.
With PSPDFKit, you can convert HTML from a web page’s URL or an HTML string to a PDF document. This tutorial explores both ways of converting HTML to a PDF document.
PSPDFKit React Native PDF Library
PSPDFKit offers a commercial React Native library for viewing, generating, annotating, and editing PDFs. You can use it to quickly add PDF functionality to your React Native applications.
It offers a variety of additional features and capabilities, including:
-
15+ out-of-the-box annotations to mark up, draw on, and add comments to documents.
-
PDF editing to merge, split, rotate, and crop documents.
-
PDF forms to create, fill, and capture PDF form data.
-
Digital signatures to validate the authenticity and integrity of a PDF.
Requirements
-
A React Native development environment for running React Native projects using the React Native command-line interface (CLI) — not the Expo CLI — and configured for the platforms you want to build (Android, iOS, or both).
Dependencies
There are two dependencies you’ll need:
![]()
The PSPDFKit React Native dependency is installed from the GitHub repository and not the npm registry. To install the PSPDFKit React Native dependency, run
yarn add github:PSPDFKit/react-native
in your project directory ornpm install github:PSPDFKit/react-native
if you’re using npm.
Installing Dependencies
To create a fresh React Native project and integrate PSPDFKit as a dependency, you can follow our getting started on React Native guide.
Now, follow the steps below to install react-native
in your project.
-
Add
react-native-fs
:
yarn add react-native-fs
-
Install all the dependencies for the project:
yarn install
-
For iOS, install CocoaPods dependencies:
cd ios
pod install
Converting an HTML String to a PDF Document
-
Use the
RNProcessor
class to generate a PDF document from an HTML string. It expects a configuration object and an HTML string as parameters:
let { fileURL } = await Processor.generatePDFFromHtmlString( configuration, htmlString, );
Refer to the list with all the configuration options you can use with RNProcessor
.
-
Present the generated PDF document:
PSPDFKit.present(fileURL, { title: 'Generate PDF from HTML' });
This will readily work on Android. However, for iOS, you’ll have to write the generated PDF document to its main bundle directory. You can write a helper function like so:
const extractAsset = async (fileURL, fileName, callBack) => { try { await RNFS.readFile(fileURL, 'base64').then((document) => { let mainPath = `${RNFS.MainBundlePath}/${documentName( fileName, )}`; RNFS.writeFile(mainPath, document, 'base64') .then((success) => { callBack(mainPath); }) .catch((e) => console.log(e)); }); } catch (error) { console.log('Error copying file', error); } };
The documentName
function is also a helper function that adds the .pdf
extension to the document if it’s missing one:
const documentName = (fileName) => { if ( fileName.toLowerCase().substring(fileName.length - 4) !== '.pdf' ) { return `${fileName}.pdf`; } return fileName; };
-
Replace the code in
App.js
with the following for a working example:
import { Platform, NativeModules } from 'react-native'; import RNFS from 'react-native-fs'; const App = () => { initPdf(); }; async function initPdf() { const { RNProcessor: Processor, PSPDFKit } = NativeModules; const configuration = { name: 'Sample', override: true, }; let htmlString = ` <html lang="en"> <head> <style> body { font-family: sans-serif; } </style><title>Demo HTML Document</title> </head> <body> <br/> <h1>PDF generated from HTML</h1> <p>Hello HTML</p> <ul> <li>List item 1</li> <li>List item 2</li> <li>List item 3</li> </ul> <p><span style="color: #ff0000;"><strong> Add some style</strong></span></p> <p> </p> </body> </html>`; try { let { fileURL } = await Processor.generatePDFFromHtmlString( configuration, htmlString, ); if (Platform.OS === 'android') { PSPDFKit.present(fileURL, { title: 'Generate PDF from HTML' }); return; } await extractAsset(fileURL, 'sample.pdf', (mainpath) => { PSPDFKit.present(mainpath, { title: 'Generate PDF from HTML', }); }); } catch (e) { console.log(e.message, e.code); alert(e.message); } } const extractAsset = async (fileURL, fileName, callBack) => { try { await RNFS.readFile(fileURL, 'base64').then((document) => { let mainPath = `${RNFS.MainBundlePath}/${documentName( fileName, )}`; RNFS.writeFile(mainPath, document, 'base64') .then((success) => { callBack(mainPath); }) .catch((e) => console.log(e)); }); } catch (error) { console.log('Error copying file', error); } }; const documentName = (fileName) => { if ( fileName.toLowerCase().substring(fileName.length - 4) !== '.pdf' ) { return `${fileName}.pdf`; } return fileName; }; export default App;
-
You can now launch the application by running:
react-native run-android
react-native run-ios
Converting a URL to PDF in React Native
Generating a PDF document from a URL is similar to generating a PDF document from an HTML string. The difference is that the output URL — and not the file URL — is used to present the document.
-
Use the
RNProcessor
class to generate a PDF document from a URL. It expects a configuration object and a URL as its parameters:
let { fileURL: outputURL } = await Processor.generatePDFFromHtmlURL( configuration, originURL, );
-
Replace the code in
App.js
with the following for a working example:
import { Platform, NativeModules } from 'react-native'; import RNFS from 'react-native-fs'; const App = () => { initPdf(); }; async function initPdf() { const { RNProcessor: Processor, PSPDFKit } = NativeModules; const configuration = { name: 'Sample', override: true, }; try { let originURL = 'https://pspdfkit.com'; let { fileURL: outputURL, } = await Processor.generatePDFFromHtmlURL( configuration, originURL, ); if (Platform.OS === 'android') { PSPDFKit.present(outputURL, { title: 'Generate PDF from URL', }); return; } await extractAsset(outputURL, 'sample.pdf', (mainpath) => { PSPDFKit.present(mainpath, { title: 'Generate PDF from URL' }); }); } catch (e) { console.log(e.message, e.code); alert(e.message); } } const extractAsset = async (fileURL, fileName, callBack) => { try { await RNFS.readFile(fileURL, 'base64').then((document) => { let mainPath = `${RNFS.MainBundlePath}/${documentName( fileName, )}`; RNFS.writeFile(mainPath, document, 'base64') .then((success) => { callBack(mainPath); }) .catch((e) => console.log(e)); }); } catch (error) { console.log('Error copying file', error); } }; const documentName = (fileName) => { if ( fileName.toLowerCase().substring(fileName.length - 4) !== '.pdf' ) { return `${fileName}.pdf`; } return fileName; }; export default App;
-
Launch the application by running:
react-native run-android
react-native run-ios
Conclusion
In this post, you learned how to convert HTML files from an HTML string and from a URL to a PDF document in React Native using PSPDFKit. In case of any hiccups, don’t hesitate to reach out to our Support team for help.
PSPDFKit for React Native is an SDK for viewing, annotating, and editing PDFs. It offers developers the ability to quickly add PDF functionality to any React Native application. Try it for free, or visit our demo to see it in action.