Converting a PDF to an Image in Java
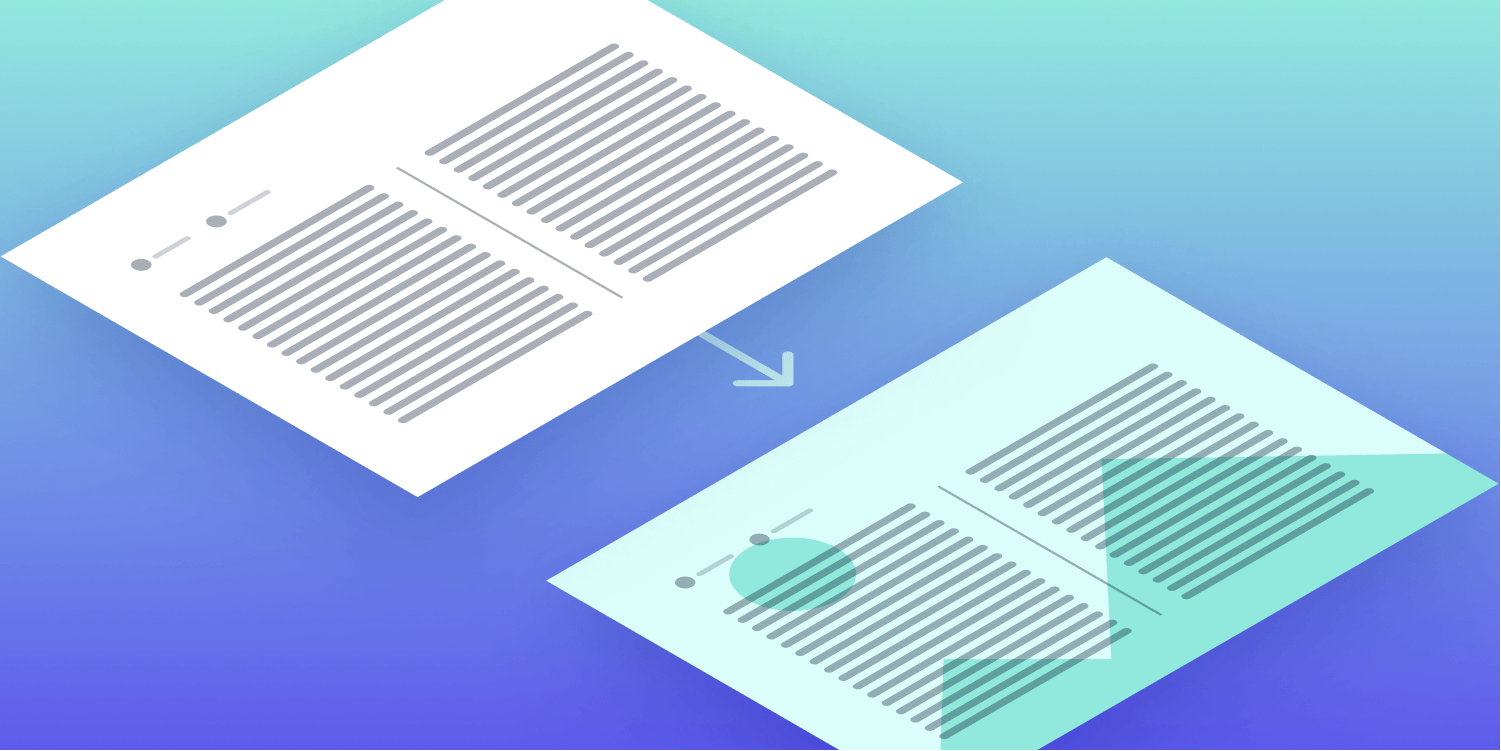
In this blog post, we’ll do a step-by-step walkthrough of how to convert PDF pages to images in both Java and Kotlin. In order to create high-fidelity page renderings, we’ll be using PSPDFKit Library for Java, because it uses an accurate and reliable PDFium-based rendering engine under the hood. Plus, its API is easy to learn and use.
Setup
You can integrate PSPDFKit Library for Java into your Maven or Gradle project in just a few minutes. Optionally, you can also integrate the JAR file directly into your project. Check out the Getting Started guide for detailed instructions — no sign up is required.
Initializing PSPDFKit
We need to first initialize PSPDFKit before calling any other method of the SDK. If you’re just getting started and haven’t yet purchased a license, you can use the initializeTrial
method to get going right away. Once you have a license key — or if you have one already — you can use the initialize
method instead:
import com.pspdfkit.api.PSPDFKitInitializeException; import com.pspdfkit.api.PSPDFKit; /** * Call this method at the beginning of your app to initialize PSPDFKit. */ public void initializePSPDFKit() throws PSPDFKitInitializeException { PSPDFKit.initializeTrial(); // PSPDFKit.initialize("<License Key>") }
import com.pspdfkit.api.PSPDFKitInitializeException import com.pspdfkit.api.PSPDFKit /** * Call this method at the beginning of your app to initialize PSPDFKit. */ @Throws(PSPDFKitInitializeException::class) fun initializePSPDFKit() { PSPDFKit.initializeTrial() // PSPDFKit.initialize("<License Key>") }
Rendering PDF Pages
Before we can save PDF pages as JPEGs or PNGs, we first need to render them. To do so, we load a PDF file, get a page, and render it with the renderPage
method:
package com.my.app; import com.pspdfkit.api.PSPDFKitInitializeException; import com.pspdfkit.api.PdfDocument; import com.pspdfkit.api.PdfPage; import com.pspdfkit.api.providers.FileDataProvider; import java.io.File; import java.awt.image.BufferedImage; public class App { public static void main(String[] args) throws PSPDFKitInitializeException { initializePSPDFKit(); final File file = new File("document.pdf"); final PdfDocument document = new PdfDocument(new FileDataProvider(file)); final PdfPage page = document.getPage(0); final BufferedImage image = page.renderPage(); } }
package com.my.app import com.pspdfkit.api.PSPDFKitInitializeException import com.pspdfkit.api.PdfDocument import com.pspdfkit.api.PdfPage import com.pspdfkit.api.providers.FileDataProvider import java.io.File import java.awt.image.BufferedImage @Throws(PSPDFKitInitializeException::class) fun main(args: Array<String>) { initializePSPDFKit() val file = File("document.pdf") val document = PdfDocument.open(FileDataProvider(file)) val page = document.getPage(0) val image = page.renderPage() }
Saving Rendered Pages as Image Files
The output of the renderPage
method is a native Java BufferedImage
object, which can be saved as an image:
import javax.imageio.ImageIO; final File imageFile = new File("page0.png"); // Use "jpeg" to save as a JPEG image instead of a PNG. ImageIO.write(image, "png", image_file);
import javax.imageio.ImageIO val imageFile = File("page0.png") // Use "jpeg" to save as a JPEG image instead of a PNG. ImageIO.write(image, "png", imageFile)
Controlling the Image Size
By passing two int
arguments to the renderPage
method, we can control the size of the rendered image. The first number specifies the image width, and the second number specifies the image height — both in pixels. This is how we can — for example — render 100×100-pixel thumbnails:
final File file = new File("document.pdf"); final PdfDocument document = new PdfDocument(new FileDataProvider(file)); final PdfPage page = document.getPage(0); final BufferedImage thumbnail = page.renderPage(100, 100);
val file = File("document.pdf") val document = PdfDocument.open(FileDataProvider(file)) val page = document.getPage(0) val thumbnail = page.renderPage(100, 100)
Converting All Pages
We can create thumbnails for all pages in a document by looping over the document pages and combining the code snippets we used earlier:
final int thumbnailWidth = 100; final int thumbnailHeight = 100; final String documentPath = "document.pdf"; // Load a PDF document. final File file = new File(documentPath); final PdfDocument document = new PdfDocument(new FileDataProvider(file)); // Loop over all pages in the document. for (int pageIndex = 0; pageIndex < document.getPageCount(); pageIndex++) { // Render a thumbnail for each page. final PdfPage page = document.getPage(pageIndex); final BufferedImage thumbnail = page.renderPage(thumbnailWidth, thumbnailHeight); // Save the thumbnail as a JPEG. final File thumbnailFile = new File("page" + pageIndex + ".jpeg"); ImageIO.write(thumbnail, "jpeg", thumbnailFile); }
val thumbnailWidth = 100 val thumbnailHeight = 100 val documentPath = "document.pdf" // Load a PDF document. val file = File(documentPath) val document = PdfDocument.open(FileDataProvider(file)) // Loop over all pages in the document. for (pageIndex in 0 until document.getPageCount()) { // Render a thumbnail for each page. val page = document.getPage(pageIndex) val thumbnail = page.renderPage(thumbnailWidth, thumbnailHeight) // Save the thumbnail as a JPEG. val thumbnailFile = File("page${pageIndex}.pdf") ImageIO.write(thumbnail, "jpeg", thumbnailFile) }
Conclusion
In this post, we used PSPDFKit’s API to convert PDF pages to images in Java and Kotlin. With just a few lines of code, we’re able to render document pages in various resolutions and save them in image formats like PNG or JPEG.
But this isn’t all you can do with PSPDFKit Library for Java. You might want to programmatically add text, highlights, or other annotations to pages before rendering them. The SDK also supports redacting sensitive information in documents before converting them to images.
You can learn about everything PSPDFKit has to offer by visiting the developer guides. If you run into problems or have questions, you can talk to our developers by reaching out to our tech support.