Private Mode Using Collaboration Permissions
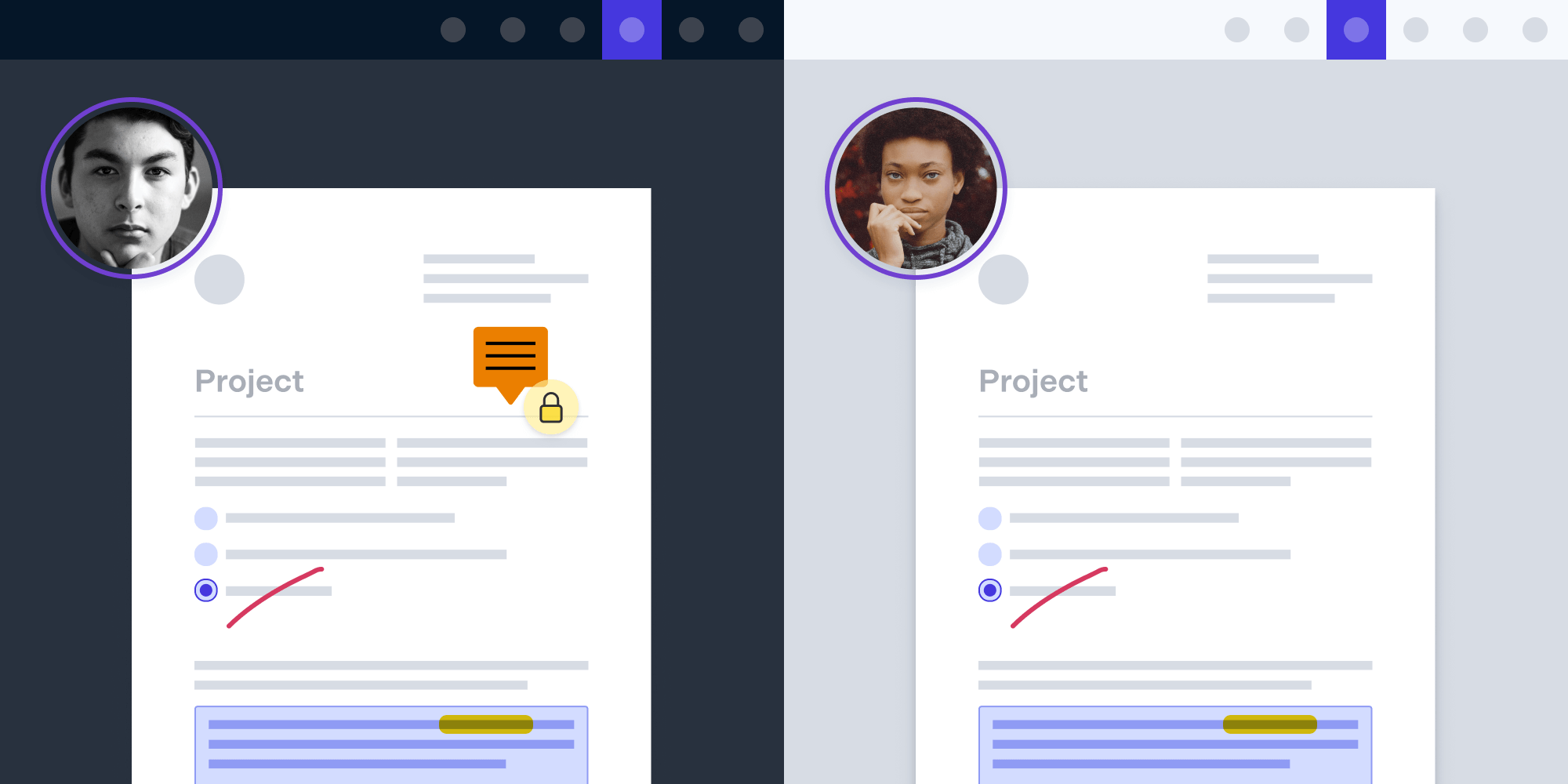
We released Collaboration Permissions with PSPDFKit for Web 2021.1. It helps you define permissions in a way that is more secure and scalable. In this blog post, we’ll see how we can implement private mode using Collaboration Permissions.
Private Mode
There might be situations where you don’t want selected annotations to be visible to others, even when they’re working on the same layer of a document. One example of such annotations is that of private note annotations.
For simplicity’s sake, we’ll only create private note annotations right now. However, you can use the same approach outlined here if you want to make your comments or form fields private.
Prerequisites
We need a Server-backed setup with a PSPDFKit Instant license. Also, please make sure that instant
is enabled in your PSPDFKit instance.
Getting Started
Let’s assume there are two users — John and Olivia — who are collaborating on a single document. They each want to write a few private note annotations for themselves that will be hidden from each another. With the exception of these selected annotations, all the other annotations will be visible to everyone.
Enabling Collaboration Permissions
The first thing we have to do is enable Collaboration Permissions for both users by making the following changes in their JSON Web Tokens (JWTs):
// John's JWT claims. const jsonWebToken = { ...otherClaims, user_id: "john", default_group: "group_john", collaboration_permissions: [] }; // Olivia's JWT claims. const jsonWebToken = { ...otherClaims, user_id: "olivia", default_group: "group_olivia", collaboration_permissions: [] };
This will enable Collaboration Permissions for both of them, but they won’t see any annotations, comments, or form fields on their document. This happens because we haven’t given them permission to view any of them yet.
Allowing Viewing One’s Own Annotations
Next, we’ll allow both John and Olivia to view all the annotations they created themselves:
// John's JWT claims. const jsonWebToken = { ...otherClaims, user_id: "john", default_group: "group_john", collaboration_permissions: ["annotations:view:self"] }; // Olivia's JWT claims. const jsonWebToken = { ...otherClaims, user_id: "olivia", default_group: "group_olivia", collaboration_permissions: ["annotations:view:self"] };
Now both of them will be able to see all the annotations they personally created. But they won’t be able to see any annotation created by the other user, i.e. John can’t see any annotation created by Olivia, and vice versa.
Allowing Collaboration
To collaborate, John and Olivia should be able to see each other’s annotations. We’ll use the group scope in permissions to allow this. All the annotations created by John will have the group group_john
, and all the annotations created by Olivia will have the group group_olivia
. So if we allow John to view all the annotations with the group group_olivia
and allow Olivia to view all the annotations with the group group_john
, both of them will be able to view all the annotations created by each other (in addition to their own):
// John's JWT claims. const jsonWebToken = { ...otherClaims, user_id: "john", default_group: "group_john", collaboration_permissions: [ "annotations:view:self", + "annotations:view:group=group_olivia" ] } // Olivia's JWT claims. const jsonWebToken = { ...otherClaims, user_id: "olivia", default_group: "group_olivia", collaboration_permissions: [ "annotations:view:self", + "annotations:view:group=group_john" ] }
Private Note Annotations
So far, we’ve allowed both users to see all the annotations created on the document. Now we have to hide the private note annotations from other users. We can do this by changing the group of those note annotations. But to change the group of any annotation, the user needs to have the permission to do that:
// John's JWT claims. const jsonWebToken = { ...otherClaims, user_id: "john", default_group: "group_john", collaboration_permissions: [ "annotations:view:self", "annotations:view:group=group_olivia" + "annotations:set-group:group=private_group_john" ] } // Olivia's JWT claims. const jsonWebToken = { ...otherClaims, user_id: "olivia", default_group: "group_olivia", collaboration_permissions: [ "annotations:view:self", "annotations:view:group=group_john", + "annotations:set-group:group=private_group_olivia" ] }
The above additions allow John and Olivia to create annotations with the groups private_group_john
and private_group_olivia
, respectively. Once they have this permission, they can now create note annotations with this group’s name instead of the default group defined in the JWT. Any annotation created with these groups won’t be visible to other users, as they don’t have permission to view the other user’s private group. For example, John doesn’t have permission to view the annotations of the group private_group_olivia
, so he cannot see the annotations made with that group name.
The private annotations will still be visible to the creators because they satisfy permissions allowed by annotations:view:self
.
Creating Private Note Annotations
Now that we’ve discussed what needs to be changed in the JWT to enable private annotations, we need to create note annotations using the new group. There are various ways in which you can create new annotations with a custom group. You can read more about the different methods in our Changing Permissions guide. Since we want to change the group of only note annotations, we can use AnnotationsPreset
and add a group field to it that will be used by all note annotations:
instance.setAnnotationPresets(presets => { presets.note = { ...presets.note, group: "private_group_john" }; return presets; });
Once the above code is executed in John’s instance, all the new note annotations he creates will have the group private_group_john
, which will make those note annotations private.
Conclusion
In this blog, we looked at the implementation of private mode, which can be useful if you want to add annotations that are only visible to the creator. This same concept can be applied to form fields and comments if you want to add private form fields and private comments. For more information, you can look at our Catalog example, where we implemented private mode for all supported content types, i.e. annotations, form fields, and comments.