How to Generate PDF Reports from HTML in PHP
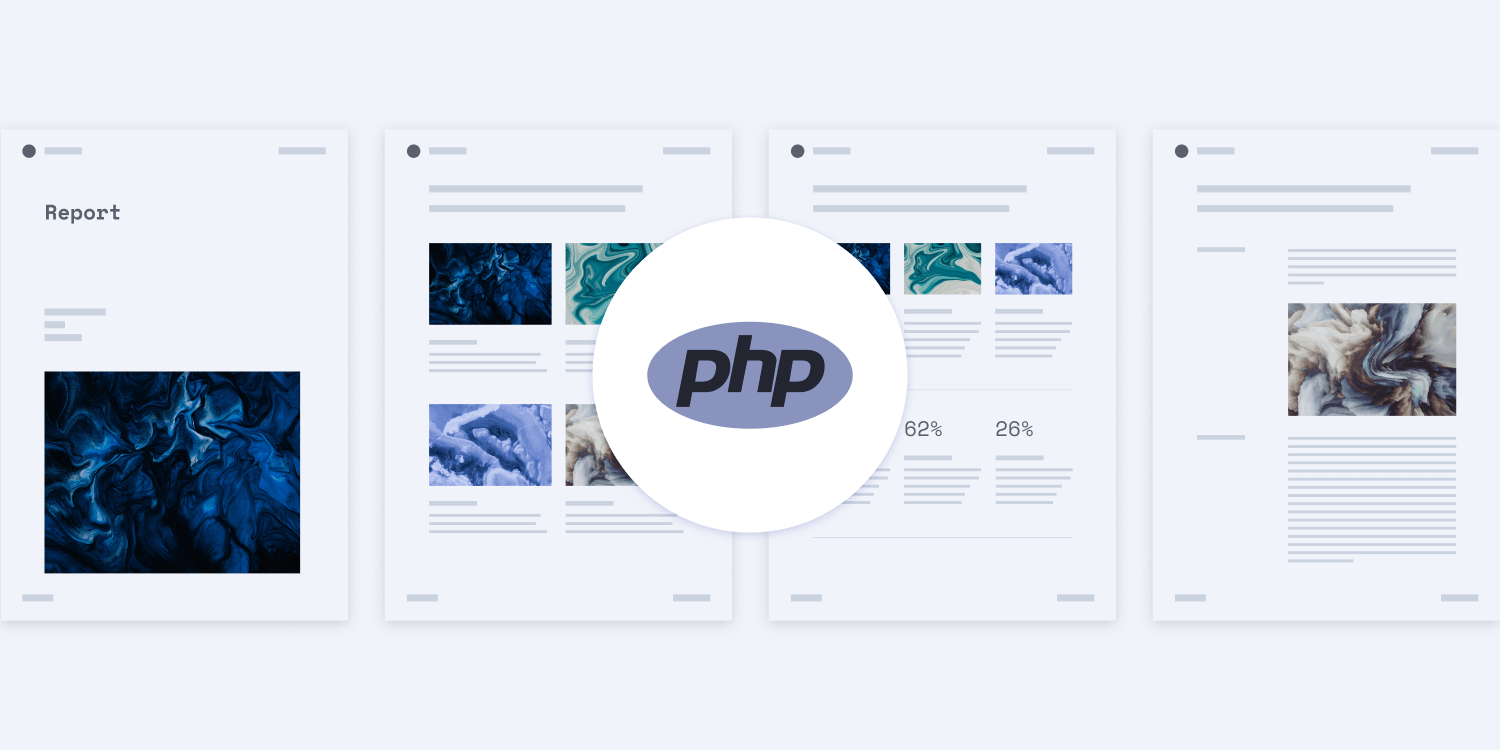
In this post, you’ll learn how to generate PDF reports from HTML using our PHP PDF Generator API. With our API, you can generate 100 PDF reports per month for free. To access your API key, sign up for a free account.
This will be especially useful if you generate and distribute a high volume of standardized reports throughout the year. With our API, you can automate your report generation by dynamically injecting data and content into a standardized HTML template.
We’ll demonstrate how you can generate a report with a free PDF report template in HTML and CSS that can be customized to meet your specific requirements. You can easily style your report by updating the CSS file with your own custom images and fonts. For reports that span multiple pages, you can add a header and footer that repeats across all your pages.
Requirements
To get started, you’ll need:
-
PHP
To access your PSPDFKit API key, sign up for a free account. Your account lets you generate 100 documents for free every month. Once you’ve signed up, you can find your API key in the Dashboard > API Keys section.
You can install PHP via Homebrew, or you can check out the other installation options.
Setup
Download the report template and extract the contents of the ZIP file into a folder. You’ll get an HTML file, Inter fonts, a Space Mono font, an SVG logo, images, and a README file.
Interacting with the API
Create a new PHP file named index.php
in the root directory. In this post, you’ll use cURL
(client URL library) to interact with PSPDFKit API.
cURL
is a library that lets you make HTTP requests in PHP. Start by initializing a cURL session using curl_init()
:
$curl = curl_init();
Preparing the Payload
Send the payload with the instructions
object. This object should be a JSON string; otherwise, the API will reject the request. Include your HTML file in the html
key and the other files in the assets
array. In this example, your assets include the SVG logo, the Inter fonts, the images, and the Space Mono font:
$instructions = '{
"parts": [
{
"html": "index.html",
"assets": [
"logo.svg",
"Inter-Bold.ttf",
"Inter-Medium.ttf",
"Inter-Regular.ttf",
"SpaceMono-Regular.ttf",
"photo-1.png",
"photo-2.png",
"photo-3.png",
"photo-4.png",
]
}
]
}';
Making the Request
Start by opening a file named result.pdf
with read and write mode (w+
). If the file doesn’t exist, it’ll be created:
$FileHandle = fopen("result.pdf", "w+");
Make a request with the curl_setopt_array()
function. This function takes an array of options and sets them.
The first argument is the cURL session, and the second argument is an array of options.
Don’t forget to replace <YOUR_API_KEY>
with your API key:
curl_setopt_array($curl, array( CURLOPT_URL => "https://api.pspdfkit.com/build", CURLOPT_CUSTOMREQUEST => "POST", CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => "", CURLOPT_POSTFIELDS => array( "instructions" => $instructions, "index.html" => new CURLFILE("index.html"), "logo.svg" => new CURLFILE("logo.svg"), "Inter-Bold.ttf" => new CURLFILE("Inter-Bold.ttf"), "Inter-Medium.ttf" => new CURLFILE("Inter-Medium.ttf"), "Inter-Regular.ttf" => new CURLFILE("Inter-Regular.ttf"), "SpaceMono-Regular.ttf" => new CURLFILE("SpaceMono-Regular.ttf"), "photo-1.png" => new CURLFILE("photo-1.png"), "photo-2.png" => new CURLFILE("photo-2.png"), "photo-3.png" => new CURLFILE("photo-3.png"), "photo-4.png" => new CURLFILE("photo-4.png"), ), CURLOPT_HTTPHEADER => array( "Authorization: Bearer <YOUR_API_KEY>", // Replace <YOUR_API_KEY> with your API key. ), CURLOPT_FILE => $FileHandle, ));
The CURLOPT_POSTFIELDS
array contains all the parts you want to send it to the API. In this case, you’ve sent the instructions
object, the HTML file, the SVG logo, the Inter fonts, and the Space Mono font.
The CURLOPT_FILE
option is used to write the response to result.pdf
.
To execute your HTTP request, use the curl_exec()
function:
$response = curl_exec($curl);
Lastly, close the cURL session using the curl_close()
function, and close the file handle:
curl_close($curl); fclose($FileHandle);
Generating the PDF
Now, run your PHP application by executing the following command:
php index.php
This will create a result.pdf
file in the current directory.
You can see the full code below:
// index.php <?php $FileHandle = fopen("result.pdf", "w+"); $curl = curl_init(); $instructions = '{ "parts": [ { "html": "index.html", "assets": [ "logo.svg", "Inter-Bold.ttf", "Inter-Medium.ttf", "Inter-Regular.ttf", "SpaceMono-Regular.ttf", "photo-1.png", "photo-2.png", "photo-3.png", "photo-4.png", ] } ] }'; curl_setopt_array($curl, array( CURLOPT_URL => "https://api.pspdfkit.com/build", CURLOPT_CUSTOMREQUEST => "POST", CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => "", CURLOPT_POSTFIELDS => array( "instructions" => $instructions, "index.html" => new CURLFILE("index.html"), "logo.svg" => new CURLFILE("logo.svg"), "Inter-Bold.ttf" => new CURLFILE("Inter-Bold.ttf"), "Inter-Medium.ttf" => new CURLFILE("Inter-Medium.ttf"), "Inter-Regular.ttf" => new CURLFILE("Inter-Regular.ttf"), "SpaceMono-Regular.ttf" => new CURLFILE("SpaceMono-Regular.ttf"), "photo-1.png" => new CURLFILE("photo-1.png"), "photo-2.png" => new CURLFILE("photo-2.png"), "photo-3.png" => new CURLFILE("photo-3.png"), "photo-4.png" => new CURLFILE("photo-4.png"), ), CURLOPT_HTTPHEADER => array( "Authorization: Bearer <YOUR_API_KEY>", // Replace <YOUR_API_KEY> with your API key. ), CURLOPT_FILE => $FileHandle, )); $response = curl_exec($curl); curl_close($curl); fclose($FileHandle); ?>
Conclusion
In this post, you generated a PDF report from an HTML template using our PHP PDF generation API. We created similar PDF report generation guides using sample code from other programming languages:
In addition to templates for generating reports, we created free templates for other commonly used documents, like receipts, invoices, and certificates. If you’re interested in generating other types of documents in PHP, check out the following posts:
- Generating PDF receipts using PHP
- Generating PDF invoices using PHP
- Generating PDF certificates using PHP
All our templates are available for you to download on our PDF Generator API page. Feel free to customize or add any CSS to the template to fit your use case or help reflect your company’s brand.