How to Generate PDF Receipts from HTML in C#
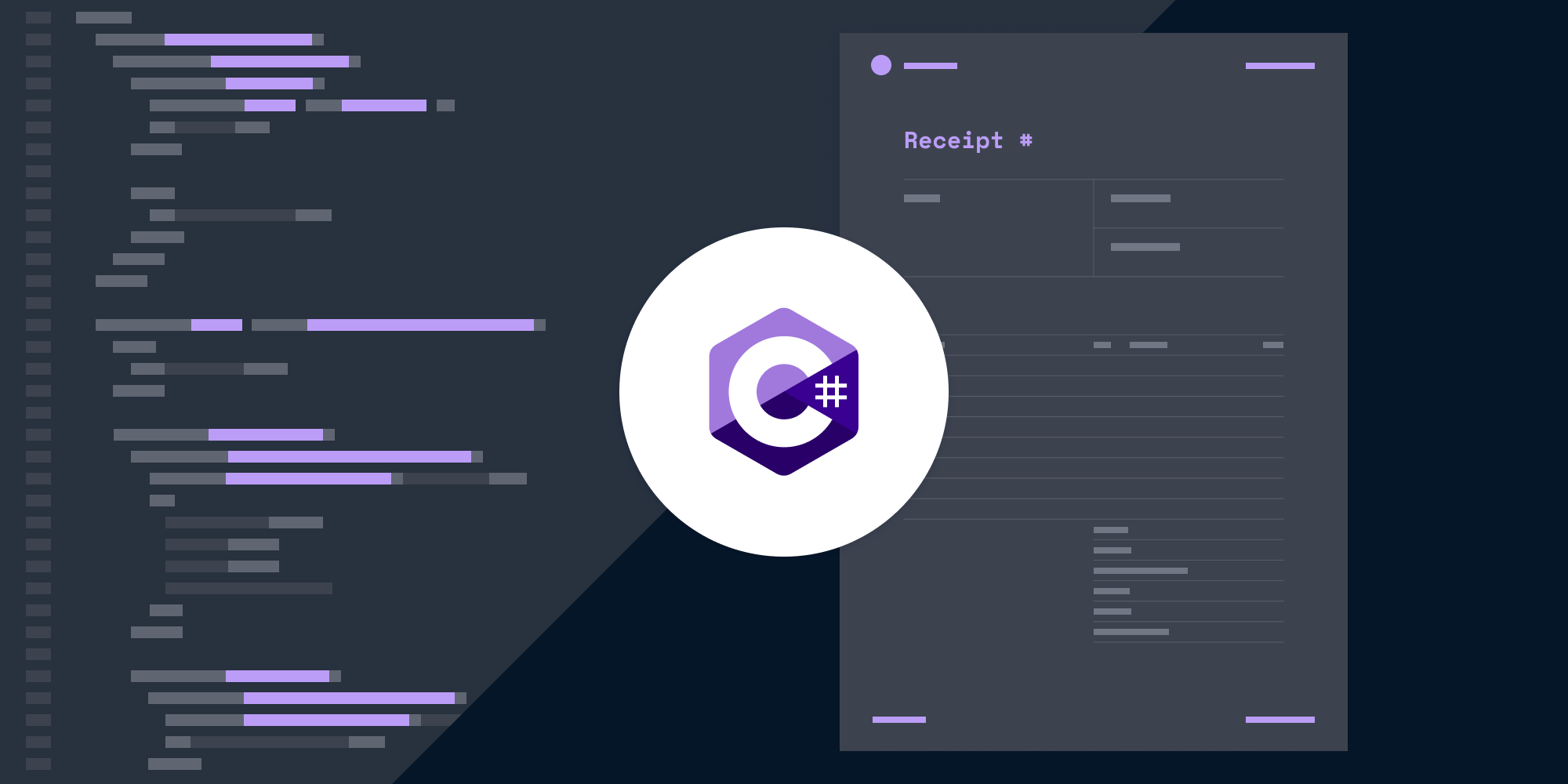
In this post, you’ll learn how to generate PDF receipts from HTML using our C# PDF Generator API. With our API, you can generate 100 PDF receipts per month for free. To access your API key, sign up for a free account.
To help you get started, we’ve provided a free receipt template in HTML and CSS that can be customized to meet your specific requirements. You can easily style your receipts by updating the CSS file with your own custom images and fonts. For receipts that are longer than one page, you can add a header and footer that repeats across all your pages.
Requirements
To get started, you’ll need:
-
The .Net SDK
To access your PSPDFKit API key, sign up for a free account. Your account lets you generate 100 documents for free every month. Once you’ve signed up, you can find your API key in the Dashboard > API Keys section.
![]()
You can find the example on GitHub.
Creating a New .NET Project
You’ll create a new .NET project via the .NET command-line interface (CLI) with the following command:
dotnet new console --name PspdfkitApiDemo
Change your directory into the newly created project directory:
cd PspdfkitApiDemo
To make an HTTP request, install RestSharp
as a dependency:
dotnet add package RestSharp -v 106.15.0
Downloading the Receipt Template
Download the receipt template and extract the contents of the ZIP file into a folder. You’ll get an HTML file, Inter fonts, a Space Mono font, an SVG logo, and a README file.
Creating a CSS File
If you open the index.html
file, you’ll see the styles between the opening and closing <style>
tag. Copy the styles to a new file called style.css
, and save it in the same folder:
@font-face { font-family: 'Inter'; src: url('Inter-Regular.ttf') format('truetype'); font-weight: 400; font-style: normal; } @font-face { font-family: 'Inter'; src: url('Inter-Medium.ttf') format('truetype'); font-weight: 500; font-style: normal; } @font-face { font-family: 'Inter'; src: url('Inter-Bold.ttf') format('truetype'); font-weight: 700; font-style: normal; } @font-face { font-family: 'Space Mono'; src: url('SpaceMono-Regular.ttf') format('truetype'); font-weight: 400; font-style: normal; } body { font-size: 0.75rem; font-family: 'Inter', sans-serif; font-weight: 400; color: #000000; margin: 0 auto; position: relative; } #pspdfkit-header { font-size: 0.625rem; text-transform: uppercase; letter-spacing: 2px; font-weight: 400; color: #717885; margin-top: 2.5rem; margin-bottom: 2.5rem; width: 100%; } .header-columns { display: flex; justify-content: space-between; padding-left: 2.5rem; padding-right: 2.5rem; } .logo { height: 1.5rem; width: auto; margin-right: 1rem; } .logotype { display: flex; align-items: center; font-weight: 700; } h2 { font-family: 'Space Mono', monospace; font-size: 1.25rem; font-weight: 400; } h4 { font-family: 'Space Mono', monospace; font-size: 1rem; font-weight: 400; } .page { margin-left: 5rem; margin-right: 5rem; } .intro-table { display: flex; justify-content: space-between; margin: 3rem 0 3rem 0; border-top: 1px solid #000000; border-bottom: 1px solid #000000; } .intro-form { display: flex; flex-direction: column; border-right: 1px solid #000000; width: 50%; } .intro-form:last-child { border-right: none; } .intro-table-title { font-size: 0.625rem; margin: 0; } .intro-form-item { padding: 1.25rem 1.5rem 1.25rem 1.5rem; } .intro-form-item:first-child { padding-left: 0; } .intro-form-item:last-child { padding-right: 0; } .intro-form-item-border { padding: 1.25rem 0 0.75rem 1.5rem; border-bottom: 1px solid #000000; } .intro-form-item-border:last-child { border-bottom: none; } .form { display: flex; flex-direction: column; margin-top: 6rem; } .no-border { border: none; } .border { border: 1px solid #000000; } .border-bottom { border: 1px solid #000000; border-top: none; border-left: none; border-right: none; } .signer { display: flex; justify-content: space-between; gap: 2.5rem; margin: 2rem 0 2rem 0; } .signer-item { flex-grow: 1; } input { color: #4537de; font-family: 'Space Mono', monospace; text-align: center; margin-top: 1.5rem; height: 4rem; width: 100%; box-sizing: border-box; } input#date, input#notes { text-align: left; } input#signature { height: 8rem; } .intro-text { width: 60%; } .table-box table, .summary-box table { width: 100%; font-size: 0.625rem; } .table-box table { padding-top: 2rem; } .table-box td:first-child, .summary-box td:first-child { width: 50%; } .table-box td:last-child, .summary-box td:last-child { text-align: right; } .table-box table tr.heading td { border-top: 1px solid #000000; border-bottom: 1px solid #000000; height: 1.5rem; } .table-box table tr.item td, .summary-box table tr.item td { border-bottom: 1px solid #d7dce4; height: 1.5rem; } .summary-box table tr.no-border-item td { border-bottom: none; height: 1.5rem; } .summary-box table tr.total td { border-top: 1px solid #000000; border-bottom: 1px solid #000000; height: 1.5rem; } .summary-box table tr.item td:first-child, .summary-box table tr.total td:first-child { border: none; height: 1.5rem; } #pspdfkit-footer { font-size: 0.5rem; text-transform: uppercase; letter-spacing: 1px; font-weight: 500; color: #717885; margin-top: 2.5rem; bottom: 2.5rem; position: absolute; width: 100%; } .footer-columns { display: flex; justify-content: space-between; padding-left: 2.5rem; padding-right: 2.5rem; }
To access the styles from index.html
, use the <link>
tag. While referring to the stylesheet file, just use the name of the file and don’t create nested paths:
<!DOCTYPE html> <html lang="en"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <link rel="stylesheet" href="style.css" /> <title>Receipt</title> </head> </html>
Interacting with the API
Now, open the Program.cs
file. It should have the Program
class with the Main
method:
using System; using System.IO; using System.Net; using RestSharp; namespace PspdfkitApiDemo { class Program { static void Main(string[] args) { // Implement your call to PSPDFKit API here. } } }
Preparing the Request
Next, you can start making your request. Use the RestClient
class to make requests to the PSPDFKit API /build
endpoint.
Then, create a RestRequest
with the required authorization header, the files you want to send, and the instructions
as a parameter:
using System; using System.IO; using System.Net; using RestSharp; namespace PspdfkitApiDemo { class Program { static void Main(string[] args) { var client = new RestClient("https://api.pspdfkit.com/build"); var request = new RestRequest(Method.POST) .AddHeader("Authorization", "Bearer {YOUR_API_KEY}") // Replace {YOUR_API_KEY} with your API key. .AddFile("index.html", "index.html") .AddFile("style.css", "style.css") .AddFile("Inter-Regular.ttf", "Inter-Regular.ttf") .AddFile("Inter-Medium.ttf", "Inter-Medium.ttf") .AddFile("Inter-Bold.ttf", "Inter-Bold.ttf") .AddFile("SpaceMono-Regular.ttf", "SpaceMono-Regular.ttf") .AddFile("logo.svg", "logo.svg") .AddParameter("instructions", new JsonObject { ["parts"] = new JsonArray { new JsonObject { ["html"] = "index.html", ["assets"] = new JsonArray { "style.css", "Inter-Regular.ttf", "Inter-Medium.ttf", "Inter-Bold.ttf", "SpaceMono-Regular.ttf", "logo.svg" } } } } .ToString()); } } }
After making a successful request, save the response to a file. Then, stream the response to a file using AdvancedResponseWriter
on your request:
request.AdvancedResponseWriter = (responseStream, response) => { if (response.StatusCode == HttpStatusCode.OK) { using (responseStream) { using var outputFileWriter = File.OpenWrite("result.pdf"); responseStream.CopyTo(outputFileWriter); } } else { var responseStreamReader = new StreamReader(responseStream); Console.Write(responseStreamReader.ReadToEnd()); } };
Executing the Request
All that’s left is to execute your request:
var response = client.Execute(request);
This will save the resulting PDF in the root directory of the project as result.pdf
.
To generate the PDF, go to your terminal and run:
dotnet run
You can see the full code below:
// Program.cs using System; using System.IO; using System.Net; using RestSharp; namespace PspdfkitApiDemo { class Program { static void Main(string[] args) { var client = new RestClient("https://api.pspdfkit.com/build"); var request = new RestRequest(Method.POST) .AddHeader("Authorization", "Bearer {YOUR_API_KEY}") // Replace {YOUR_API_KEY} with your API key. .AddFile("index.html", "index.html") .AddFile("style.css", "style.css") .AddFile("Inter-Regular.ttf", "Inter-Regular.ttf") .AddFile("Inter-Medium.ttf", "Inter-Medium.ttf") .AddFile("Inter-Bold.ttf", "Inter-Bold.ttf") .AddFile("SpaceMono-Regular.ttf", "SpaceMono-Regular.ttf") .AddFile("logo.svg", "logo.svg") .AddParameter("instructions", new JsonObject { ["parts"] = new JsonArray { new JsonObject { ["html"] = "index.html", ["assets"] = new JsonArray { "style.css", "Inter-Regular.ttf", "Inter-Medium.ttf", "Inter-Bold.ttf", "SpaceMono-Regular.ttf", "logo.svg" } } } } .ToString()); request.AdvancedResponseWriter = (responseStream, response) => { if (response.StatusCode == HttpStatusCode.OK) { using (responseStream) { using var outputFileWriter = File.OpenWrite("result.pdf"); responseStream.CopyTo(outputFileWriter); } } else { var responseStreamReader = new StreamReader(responseStream); Console.Write(responseStreamReader.ReadToEnd()); } }; client.Execute(request); } } }
Conclusion
In this post, you generated a PDF receipt from an HTML template using our C# PDF generation API. We created similar PDF receipt generation blog posts using sample code from other programming languages:
In addition to templates for generating receipts, we created free templates for other commonly used documents, like invoices, certificates, and reports. If you’re interested in generating other types of documents in C#, check out the following posts:
- Generating PDF invoices using C#
- Generating PDF certificates using C#
- Generating PDF reports using C#
All our templates are available for you to download on our PDF Generator API page. Feel free to customize or add any CSS to the template to fit your use case or help reflect your company’s brand.