How to Build a Blazor Image Viewer with PSPDFKit
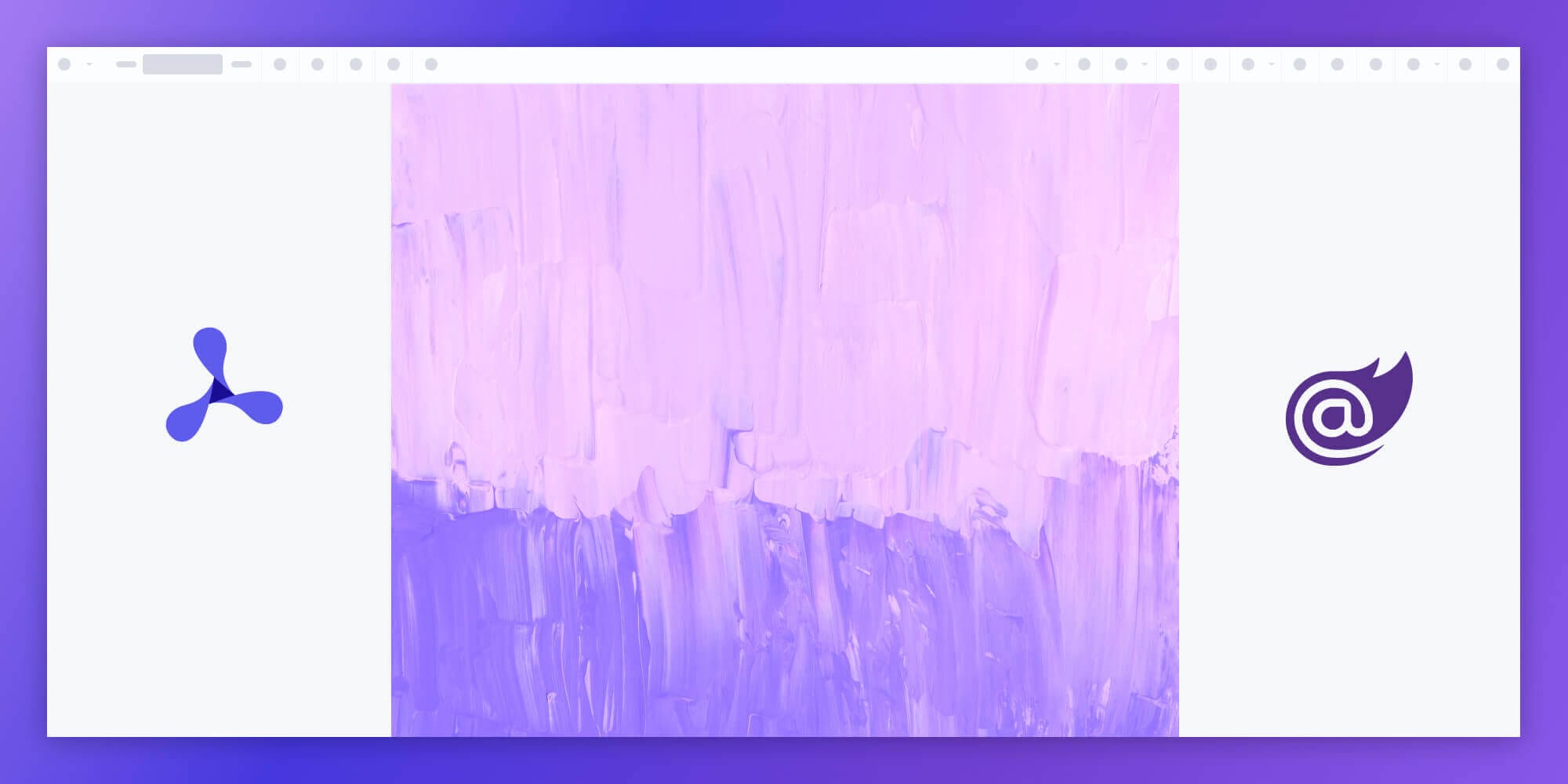
In this post, we provide you with a step-by-step guide outlining how to deploy PSPDFKit’s Blazor image viewer.
Blazor is a user interface framework in the same vein as Angular, React, and Vue. It offers all the benefits of a modern frontend framework while working entirely in C#, which is compiled and type safe.
By using Blazor, developers build frontend applications with C#, HTML, and Razor templates. Blazor apps are composed of reusable web UI components that can then be used inside your applications.
Hosting Models
-
Blazor Server
-
Blazor WebAssembly (WASM)
-
Blazor Hybrid
Blazor Hybrid isn’t recommended for use in production, but Blazor WASM and Blazor Server work almost the same way. To learn more about the models, refer to the documentation on Blazor hosting models.
In this post, you’ll use the Blazor WASM hosting model to create an image viewer.
What Is Blazor WASM?
Blazor WASM and Blazor Server reuse code and libraries from each other. Client-side code or C# code can run directly in the browser using WebAssembly.
Alternatively, Blazor can run your client logic on the server. The client UI events are sent back to the server using SignalR, which is a real-time messaging framework for ASP.NET.
Once execution completes, the required UI changes are sent to the client and merged into the DOM.
What Is WebAssembly?
WebAssembly (WASM) is a low-level instruction format that allows us to compile languages other than JavaScript (such as C++ or Rust) into a format that’s “understandable” by JavaScript virtual machines.
What Is a Blazor Image Viewer?
A Blazor image viewer lets you render and view image documents in a web browser without the need to download it to your hard drive or use an external application like a PDF reader.
PSPDFKit Blazor Image Viewer
We offer a commercial Blazor image viewer library that can easily be integrated into your web application. It comes with 30+ features that let you view, annotate, edit, and sign documents directly in your browser. Out of the box, it has a polished and flexible UI that you can extend or simplify based on your unique use case.
- A prebuilt and polished UI for an improved user experience
- 15+ prebuilt annotation tools to enable document collaboration
- Support for more file types with client-side PDF, MS Office, and image viewing
- Dedicated support from engineers to speed up integration
Example of Our Blazor Image Viewer
To see our image viewer in action, upload a JPG, PNG, or TIFF file by selecting Choose Example > Standalone > Open Document. Once your image is displayed in the viewer, you can try drawing freehand, adding a note, or applying a crop or an e-signature.
Requirements to Get Started
To get started, you’ll need:
-
.NET 6.0, which is the current Long Term Support (LTS) version of .NET Core.
You can download it from Microsoft’s website.
Creating a New Blazor WASM Project
-
For this tutorial, you’ll install the Blazor WebAssembly template using the .NET CLI (command-line interface). Open your terminal, navigate to the directory you want to create your project in, and run the following command:
dotnet new blazorwasm -o PSPDFKit_BlazorWASM
This command creates your new Blazor app project and places it in a new directory called PSPDFKit_BlazorWASM
inside your current location.
-
Change your directory into the newly created project:
cd PSPDFKit_BlazorWASM
blazorwasm
is a template that creates the initial files and directory structure for a Blazor WebAssembly project.
Below, you’ll see the file structure of your project.
-
Pages
— This folder contains the routable components/pages. The route for each page is specified using the@page
directive. You’ll work on theHome
page, which is theIndex.razor
file. -
Properties/launchSettings.json
— This holds the development environment configuration. -
Shared
— This folder contains the shared components and stylesheets. TheMainLayout.razor
file defines the app’s layout component. -
wwwroot
— This folder contains the public static assets. You’ll store the PDF file and PSPDFKit artifacts here. -
_Imports.razor
— This includes common Razor directives to include in the app’s components (.razor
), such as@using
directives for namespaces. -
App.razor
— This is the root component for the app. -
Program.cs
— This is the entry point for the application that starts the server, and it’s where you configure the app services and middleware. -
PSPDFKit_BlazorWASM.csproj
— This defines the app project and its dependencies.
Adding PSPDFKit to Your Project
PSPDFKit for Web library files are distributed as an archive that can be extracted manually.
-
Download the framework here. The download will start immediately and will save a
.tar.gz
archive likePSPDFKit-Web-binary-2022.2.1.tar.gz
to your computer. -
Once the download is complete, extract the archive and copy the entire contents of its
dist
folder to your project’swwwroot
folder. -
Make sure your
wwwroot
folder contains thepspdfkit.js
file and apspdfkit-lib
directory with the library assets.
Displaying an Image Document
-
Add the image you want to display to the
wwwroot
directory. You can use our demo image as an example. -
Navigate to the
Shared/MainLayout.razor
file. The layout component inherits fromLayoutComponentBase
, and it adds a@Body
property to the component, which contains the content to be rendered inside the layout. During rendering, the@Body
property will be replaced with the content of the layout:
@inherits LayoutComponentBase @Body
-
Now you’ll start working on the
Home
route. Navigate to your project’sPages/index.razor
file, and replace the contents of the file with the following:
@page "/" @inject IJSRuntime JS <div id='container' style='background: gray; width: 100vw; height: 100vh; margin: 0 auto;'></div> @code { protected override async void OnAfterRender(bool firstRender) { if (firstRender) { await JS.InvokeVoidAsync("loadPDF", "#container", "image.png"); } } }
Here, the @page
directive is pointing to the Home
(/) route.
To be able to invoke JavaScript functions from .NET, inject the IJSRuntime
abstraction and call the InvokeVoidAsync
method, which doesn’t return a value.
The div
element is used to display the PDF document.
-
Load the PSPDFKit SDK loading code to
wwwroot/index.html
before the</body>
tag:
<script src="pspdfkit.js"></script> <script> function loadPDF(container, document) { PSPDFKit.load({ container, document, }); } </script>
Serving the Application
-
Start the app in the root directory of your project:
dotnet watch run
If you get a prompt asking for permissions, type your keychain and click Always Allow.
The server will start and will automatically restart when changes are made to the project.
![]()
You can access the source code for this tutorial on GitHub. Just navigate to the 'wasm' folder. If you're looking for the Blazor Server example, you can find the example project under the 'server' folder or follow our getting started guide here.
Adding Even More Capabilities
Once you’ve deployed your viewer, you can start customizing it to meet your specific requirements or easily add more capabilities. To help you get started, here are some of our most popular Blazor guides:
- Adding annotations
- Editing documents
- Filling PDF forms
- Adding signatures to documents
- Real-time collaboration
- Redaction
- UI customization
Conclusion
You should now have our Blazor image viewer up and running in your web application. If you hit any snags, don’t hesitate to reach out to our Support team for help.
You can also integrate our JavaScript image viewer using web frameworks like Angular and React.js. To see a list of all web frameworks, start your free trial. Or, launch our demo to see our viewer in action.