How to Fill Out PDF Forms in Java
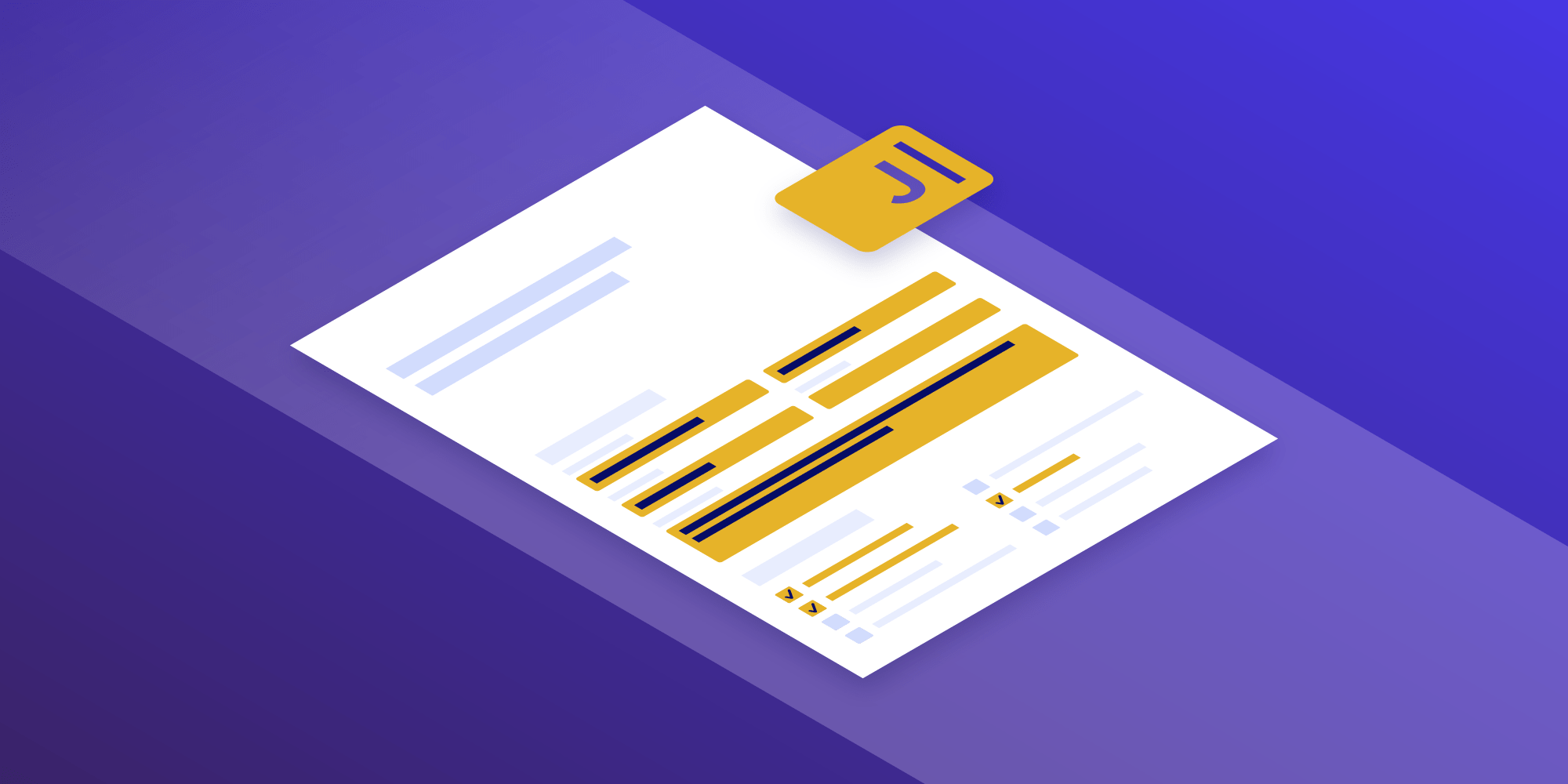
PDF forms are widely used in many onboarding processes and account creation requests. Due to the well-defined standard of the PDF specification and vast support across many different platforms, it’s easy to see why companies and individuals want to work with PDF forms.
Programmatically Filling PDF Forms
Most people would expect a person to fill out a PDF field by field, but today we’ll cover a slightly different use case, in which we’ll programmatically fill out a PDF form. This use case could be for when there’s a mobile- or web-based onboarding system where the UI can enhance the experience for the user, but we still want the “paper” trail to be created with PDFs for electronic archiving purposes.
This is where our Java PDF library can come in handy. Once we’ve collated all the data from user input, we’ll require a library to fill the PDF form programmatically. At this point, we can save the filled-in document and send it or manipulate it further.
In the following example, we’ll see how PDF form filling is possible in Java with PSPDFKit Library for Java. You can jump in straight away by downloading the trial today.
Prepare the Data
First, we’ll look at setting up the data in a way that PSPDFKit Library for Java can understand and in a way that maps to the target document.
The great news is that PSPDFKit Library for Java supports PDF form filling with JSON, which is often the format of the received data. If your data isn’t in JSON format, it’s easy to map the data with plenty of open source libraries like org.json.
Let’s say we have the following, all of which are standard pieces of information we’d normally collect during an onboarding session:
-
First Name
-
Last Name
-
DOB
-
Address
-
Telephone Number
The arrangement of data that PSPDFKit Library for Java requires is a JSON object with key-value pairs, where the key is the name of the form field, and the value is the value to assign.
In our PDF form, the names of the form fields we’d like to fill, as defined by the document, are:
Name_First Name_Last Birthdate Address_1 Telephone_Home
To create the JSON data, we use a JSONObject
to set up our key-value pairs:
JSONObject valuesToSet = new JSONObject(); valuesToSet.put("Name_First", "John"); valuesToSet.put("Name_Last", "Smith"); valuesToSet.put("Birthdate", "12/12/12"); valuesToSet.put("Address_1", "123 My Street"); valuesToSet.put("Telephone_Home", "+12345678");
You can imagine all the values could be data-driven from a number of different sources: a database, a RESTful API, or any other unique data source.
Filling the PDF Form Field Values
Now that we have our data in a format that PSPDFKit Library for Java can read, we can set the form fields on the document. Note that the list of values doesn’t have to be exhaustive; we can set either all or a subset of the fields, and the values will be taken.
There’s only one call we have to make to set the form fields: setFormFieldValuesJson
. And we’ll be calling this method on the document we want to set the form fields on:
PdfDocument document = PdfDocument.open(new FileDataProvider(new File("formDocument.pdf"))); ... document.getFormProvider().setFormFieldValuesJson(valuesToSet);
If any of the field names provided cannot be found, an exception will be thrown.
Reading PDF Form Field Values
In some cases, we may want to read what form fields are already filled or get a list of form fields within the document. getFormFieldValuesJson
allows us to query the entire document for form fields. The method will return the values for all the form fields found in the document:
PdfDocument document = PdfDocument.open(new FileDataProvider(new File("formDocument.pdf"))); ... document.getFormProvider().getFormFieldValuesJson();
formFieldValues
will hold a JSON object, again with key-value pairs:
{ "Name_First": "John", "Name_Last": "Smith", "Name_Middle": null, .... }
We can see from the JSON above that the first name and last name were set, but we can also see that there was an additional middle name. It wasn’t filled because we didn’t give a value for Name_Middle
. This is perfectly acceptable, and in this case, the field will remain blank. We know that nothing was set because Name_Middle
gives a value of null
.
Saving the Document
Now we want to save the changes to ensure the values are reflected in the document permanently. To save, we need to call save
on the document:
document.save(new DocumentSaveOptions.Builder().build());
The above code will save the changes back to the same data provider that was opened. If we’d like to save to a different location, we can use saveAs
.
It’s also worth noting that the form fields in the saved document will still be editable. Therefore, the document can be opened again and the form field values can be changed.
If we want to ensure the form is non-editable, we have the option to flatten the PDF form fields. Flattening the PDF form means all the form boxes and annotations on the pages will be embedded into the contents of the document and will no longer be editable with a standard PDF viewer. However, be aware that just because a standard viewer cannot edit a document, it doesn’t mean others can’t. If you want to ensure a document isn’t tampered with, please look into our Digital Signatures SDK.
document.save(new DocumentSaveOptions.Builder().flattenAnnotations(true).build());
From the above, we can see we just need to use the flattenAnnotations
flag and the document will be fully rewritten with all the annotations and form fields embedded as part of the contents of the document.
Conclusion
From this blog post, you learned that with just a few lines of code, you can take a data source, fill out a PDF form, and save and flatten that data back into the document for use elsewhere.
The steps outlined above can be adapted to support many different scenarios — for example, reading the data from a database, automating the forwarding of the filled PDF to the next department, or even performing post-form filling document editing as seen in our How to Merge Two or More PDFs in Java (or Kotlin) post.
Learn more about our Java PDF Form Library, or get started by downloading a free trial of our Java library.
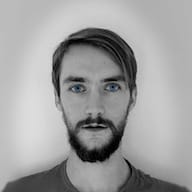
When Nick started tinkering with guitar effects pedals, he didn’t realize it’d take him all the way to a career in software. He has worked on products that communicate with space, blast Metallica to packed stadiums, and enable millions to use documents through PSPDFKit, but in his personal life, he enjoys the simplicity of running in the mountains.