PSPDFKit 💖 React Native
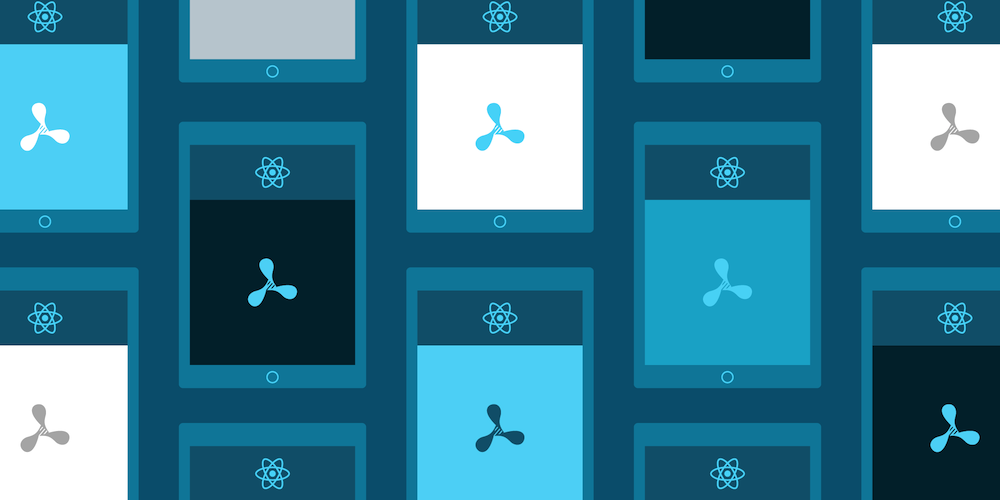
React Native enables you to build native mobile apps using a consistent developer experience based on JavaScript and React. It’s built by Facebook and already used by thousands of apps in production. We were motivated by the momentum and popularity of React Native and decided to give its users an easy way to use PSPDFKit in their projects.
See also: React Native UI Component for iOS, for Android, and Windows UWP.
That’s why we made our React Native PDF library on GitHub publicly available. Now, it’s easy to present a full-featured PDF viewer in your React Native app. After you follow the installation steps from our getting started guide, you can present a PDF document like this:
import React, { Component } from 'react'; import { AppRegistry, StyleSheet, NativeModules, Text, TouchableHighlight, View, } from 'react-native'; var PSPDFKit = NativeModules.PSPDFKit; class ReactNativeApp extends Component { _onPressButton() { PSPDFKit.present('document.pdf', {}); } render() { return ( <View style={styles.container}> <TouchableHighlight onPress={this._onPressButton}> <Text style={styles.text}>Tap to Open Document</Text> </TouchableHighlight> </View> ); } } const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center', backgroundColor: '#F5FCFF', }, text: { fontSize: 20, textAlign: 'center', margin: 10, }, }); AppRegistry.registerComponent('ReactNativeApp', () => ReactNativeApp);
All the heavy lifting is done by the PSPDFKit.present
method.
Configuration
It’s easy to configure your PDF viewer. Pass a configuration dictionary into the PSPDFKit.present
method:
PSPDFKit.present('document.pdf', { showThumbnailBar: 'scrollable', pageTransition: 'scrollContinuous', scrollDirection: 'vertical', });
Now the viewer uses continuous vertical scrolling and a scrollable thumbnail bar.
The configuration dictionary is a mirror of the PSPDFConfiguration
class.
Android
With React Native, it’s possible to write an iOS and Android app using the same code. To make this possible, our library supports both iOS and Android. Follow our getting started guide for Android to run your app on an Android device.
Summary
Our React Native PDF SDK offers a variety of additional features and capabilities, including:
-
15+ out-of-the-box annotations to mark up, draw on, and add comments to documents.
-
PDF editing to merge, split, rotate, and crop documents.
-
PDF forms to create, fill, and capture PDF form data.
-
Digital signatures to validate the authenticity and integrity of a PDF.
-
And much more!
At PSPDFKit, we offer a commercial, feature-rich, and completely customizable React Native PDF library that’s easy to integrate and comes with well-documented APIs. To get started, try it for free.