Toggle Layer (OCG) Visibility in a PDF Using JavaScript
This guide explains how to programmatically toggle or lock the visibility of OCG layers using a variety of different APIs. Additionally, end users have the option to adjust layer visibility through the user interface (UI), which is explained in the built-in UI guide.
![]()
Working with PDF layers (OCG layers) is available when using the Web SDK in standalone operational mode.
PSPDFKit for Web exposes several APIs to manage the OCG layers present in a document:
-
instance.getLayers()
returns the list of OCG layers present in the document. -
instance.getLayersVisibilityState()
returns the current visibility state of the OCG layers in the document in the form of a list of OCG layer IDs currently visible in the document. -
instance.setLayersVisibilityState({ visibleLayerIds: [LayerId] })
makes the OCG layer IDs passed as arguments visible, hiding any other layers not included in theArray
. -
ViewState#sidebarOptions
allows you to lock the visibility of OCG layers and to specify where to render visibility and lock icons in the sidebar.
Toggling the OCG Layer Visibility Programmatically
To toggle the visibility of OCG layers programmatically, use the instance.setLayersVisibilityState()
method. The example below first gathers the information about OCG layers present in a document, and then it checks which ones are visible. Finally, it sets a subset of them as visible:
// Get OCG layers in document. const OCGLayers = await instance.getLayers(); // List of PSPDFKit.OCG // Get visible OCG layers. const visibleLayers = await instance.getLayersVisibilityState(); // visibleLayers = { visibleLayerIds: [86, 72, 121, 118] } // Set OCG layer IDs `86` and `72` as visible. await instance.setLayersVisibilityState({ visibleLayerIds: [86, 72] }); // Running this would make these two layers visible while hiding layers `121` and `118`.
Locking the OCG Layer Visibility Programmatically
With PSPDFKit, it’s possible to lock the visibility of an OCG layer, effectively disallowing the end user from toggling the layer visibility.
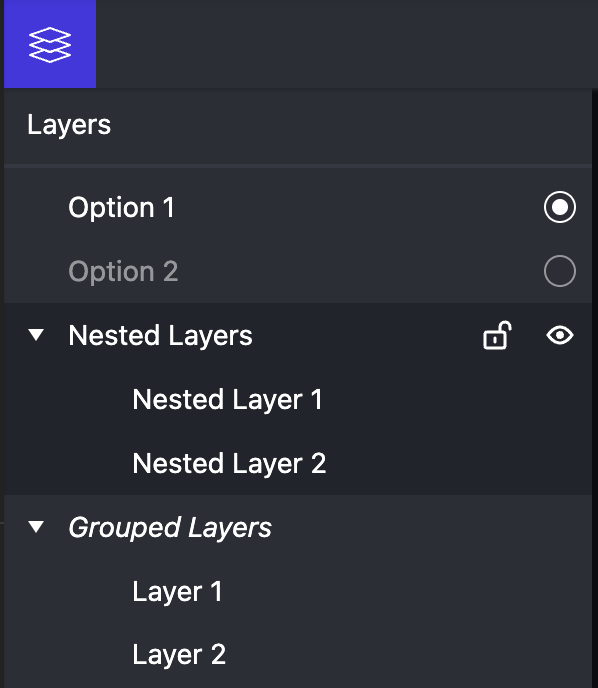
When a locked OCG layer is rendered in the sidebar, PSPDFKit will show a locked icon next to it. Locking an item will lock all its children as well, which will show a gray dot instead of the lock icon.
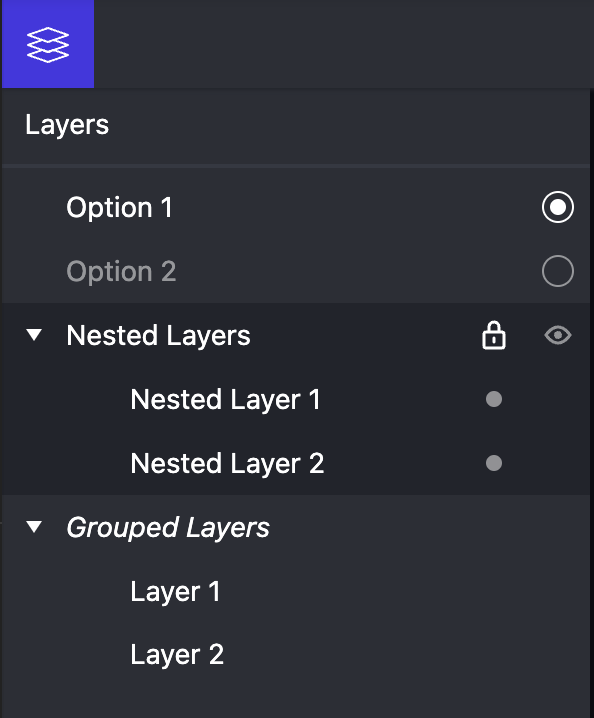
You can control locking layers via the lockedLayers
property of the PSPDFKit.ViewState#sidebarOptions
for PSPDFKit.SidebarMode.LAYERS
.
By default, OCG layers aren’t locked.
The following example shows how to lock the visibility of two OCG layers present in a document that correspond to the ocgId
of 86
and 72
:
PSPDFKit.load({ initialViewState: new PSPDFKit.ViewState({ sidebarOptions: { [PSPDFKit.SidebarMode.LAYERS]: { lockedLayers: [86, 72] // Array of `ocg.ocgId` present in the document that we want to lock. // Other configurations. } } }) });