Open Password-Protected PDFs on iOS
PSPDFKit for iOS enables you to open password-protected PDFs. This article shows how to open a password-protected PDF document both using the user interface and programmatically.
First, you need to add the password-protected PDF document you want to display to your application by dragging it into your project. On the dialog that’s displayed, select Finish to accept the default integration options. You can use this password-protected PDF file as an example.
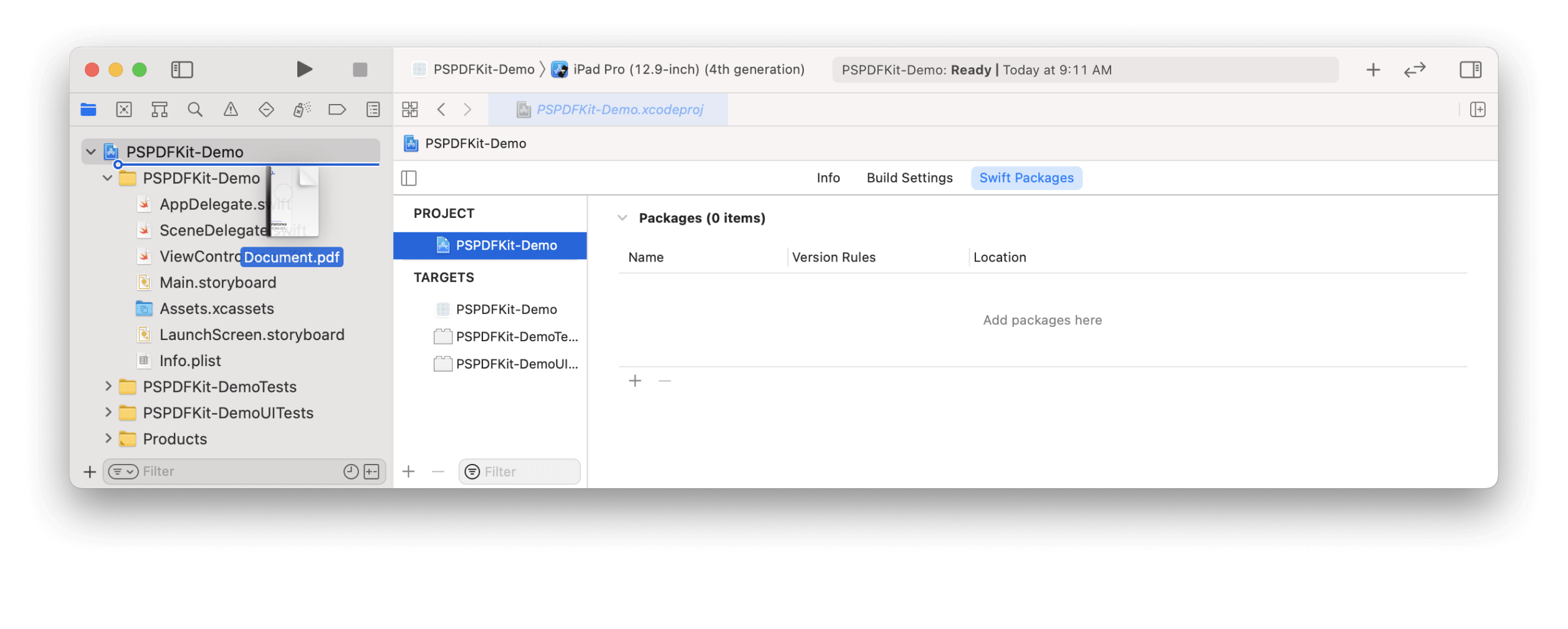
Then, load your PDF document and display the view controller. This can be done in a button action handler, table view cell selection delegate, or similar:
// Update to use your document name and location. let fileURL = Bundle.main.url(forResource: "Document", withExtension: "pdf")! let document = Document(url: fileURL) // The configuration closure is optional and allows additional customization. let pdfController = PDFViewController(document: document) { $0.isPageLabelEnabled = false } // Present the PDF view controller within a `UINavigationController` to show built-in toolbar buttons. present(UINavigationController(rootViewController: pdfController), animated: true)
When you open the password-protected PDF, PSPDFKit will prompt you to enter the password. You can use “test123” as the password to unlock the document:
![]()
For more details about opening a password-protected document programmatically, refer to
PasswordNotPresetExample.swift
in the PSPDFKit Catalog app.
Opening a Password-Protected PDF Programmatically
PSPDFKit also allows you to programmatically unlock password-protected PDFs by presetting the password before loading the document, allowing you to bypass the password prompt for your end users:
// Update to use your document name. let fileURL = Bundle.main.url(forResource: "Document", withExtension: "pdf")! let document = Document(url: fileURL) // Unlock the document before displaying it. // Note: In a real application, you should protect the password better than hardcoding it here. document.unlock(withPassword: "test123") // The configuration closure is optional and allows additional customization. let pdfController = PDFViewController(document: document) { $0.isPageLabelEnabled = false } // Present the PDF view controller within a `UINavigationController` to show built-in toolbar buttons. present(UINavigationController(rootViewController: pdfController), animated: true)
![]()
For more details about opening a password-protected document programmatically, refer to
PasswordPresetExample.swift
in the PSPDFKit Catalog app.