Configuring Scroll Directions and Page Transitions in Our Viewer
You can configure the page transition, scroll direction, and scroll mode of a PDFViewController
in PDFConfiguration
(see the PDF configuration guide for more information).
In SettingsExample
from the Catalog app, you’ll see a complete example of how these properties interact together in the context of a PDFSettingsViewController
. A PDFSettingsViewController
is used to change key UX settings that are configurable via the PDFConfiguration.settingsOptions
property.
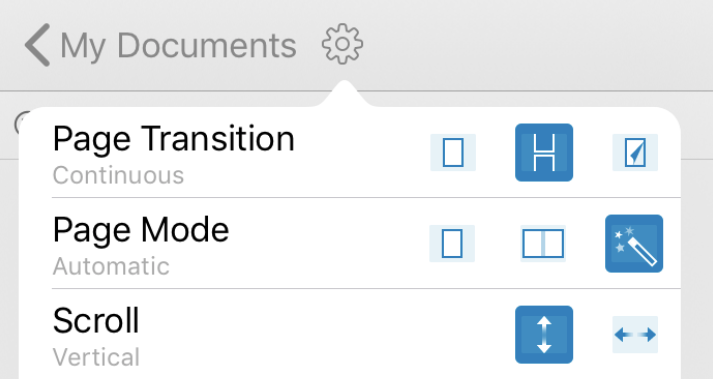
You need to be aware of the following constraints when using these PDFConfiguration
properties (pageTransition
, scrollDirection
, and pageMode
) simultaneously.
PSPDFPageTransitionScrollPerSpread
If the page transition is PageTransition.scrollPerSpread
, there are no constraints:
-
The
scrollDirection
property can be eitherScrollDirection.horizontal
orScrollDirection.vertical
-
The
pageMode
property can bePageMode.single
,PageMode.double
, orPageMode.automatic
.
let configuration = PDFConfiguration { builder in // When the page transition is `scrollPerPage`. builder.pageTransition = .scrollPerSpread builder.scrollDirection = .vertical // Can also be `.horizontal`. builder.pageMode = .single // Can also be `.double` or `.automatic`. }
PSPDFConfiguration *configuration = [PSPDFConfiguration configurationWithBuilder:^(PSPDFConfigurationBuilder *builder) { // When the page transition is `PSPDFPageTransitionScrollPerSpread`. builder.pageTransition = PSPDFPageTransitionScrollPerSpread; builder.scrollDirection = PSPDFScrollDirectionVertical; // Can also be `PSPDFScrollDirectionHorizontal`. builder.pageMode = PSPDFPageModeSingle; // Can also be `PSPDFPageModeDouble` or `PSPDFPageModeAutomatic`. }];
PSPDFPageTransitionScrollContinuous
If the page transition is PageTransition.scrollContinuous
:
-
The
scrollDirection
property can be eitherScrollDirection.horizontal
orScrollDirection.vertical
. -
If
ScrollDirection.vertical
is used, thepageMode
property can bePageMode.single
,PageMode.double
, orPageMode.automatic
. It will be forced toPageMode.single
ifScrollDirection.horizontal
is used.
let configuration = PDFConfiguration { builder in // When the page transition is `scrollContinuous`. builder.pageTransition = .scrollContinuous builder.scrollDirection = .vertical // Can also be `.horizontal`. builder.pageMode = .single // Setting a value to `pageMode` will only be honored if `scrollDirection` is `.vertical`. It will be forced to single otherwise. }
PSPDFConfiguration *configuration = [PSPDFConfiguration configurationWithBuilder:^(PSPDFConfigurationBuilder *builder) { // When the page transition is `PSPDFPageTransitionScrollContinuous`. builder.pageTransition = PSPDFPageTransitionScrollContinuous; builder.scrollDirection = PSPDFScrollDirectionVertical; // Can also be `PSPDFScrollDirectionHorizontal`. builder.pageMode = PSPDFPageModeSingle; // Setting a value to `pageMode` will only be honored if `scrollDirection` is `PSPDFScrollDirectionVertical`. It will be forced to `PSPDFPageModeSingle` otherwise. }];
PSPDFPageTransitionCurl
If the page transition is PageTransition.curl
:
-
The value of
scrollDirection
will be forced toScrollDirection.horizontal
. -
The
pageMode
property can bePageMode.single
,PageMode.double
, orPageMode.automatic
.
let configuration = PDFConfiguration { builder in // When the page transition is `curl`. builder.pageTransition = .curl builder.scrollDirection = .horizontal // Setting a value to the `scrollDirection` property will be ignored. It will be forced to `.horizontal`. builder.pageMode = .single // Can also be `.double` or `.automatic`. }
PSPDFConfiguration *configuration = [PSPDFConfiguration configurationWithBuilder:^(PSPDFConfigurationBuilder *builder) { // When the page transition is `PSPDFPageTransitionCurl`. builder.pageTransition = PSPDFPageTransitionCurl; builder.scrollDirection = PSPDFScrollDirectionHorizontal; // Setting a value to the `scrollDirection` property will be ignored. It will be forced to `PSPDFScrollDirectionHorizontal`. builder.pageMode = PSPDFPageModeSingle; // Can also be `PSPDFPageModeDouble` or `PSPDFPageModeAutomatic`. }];
Persisting the Settings Options Using the User Defaults
You can persist the settings options for your PDFSettingsViewController
in your app’s UserDefaults
.
Saving the Settings Options
You can save the settings in your app’s user defaults in PDFViewControllerDelegate.pdfViewControllerDidDismiss(_:)
, like so:
func pdfViewControllerDidDismiss(_ pdfController: PDFViewController) { // Persist the settings options in the user defaults. let defaults = UserDefaults.standard defaults.set(pdfController.configuration.pageTransition.rawValue, forKey: "pageTransition") defaults.set(pdfController.configuration.pageMode.rawValue, forKey: "pageMode") defaults.set(pdfController.configuration.scrollDirection.rawValue, forKey: "scrollDirection") defaults.set(pdfController.configuration.spreadFitting.rawValue, forKey: "spreadFitting") }
- (void)pdfViewControllerDidDismiss:(PSPDFViewController *)pdfController { // Persist the settings options in the user defaults. NSUserDefaults *defaults = NSUserDefaults.standardUserDefaults; [defaults setInteger:pdfController.configuration.pageTransition forKey: @"pageTransition"]; [defaults setInteger:pdfController.configuration.pageMode forKey: @"pageMode"]; [defaults setInteger:pdfController.configuration.scrollDirection forKey: @"scrollDirection"]; [defaults setInteger:pdfController.configuration.spreadFitting forKey: @"spreadFitting"]; }
Restoring the Settings Options
You can restore the saved settings options from your app’s user defaults in your PDFConfiguration
, as seen below:
let configuration = PDFConfiguration { builder in // Restore the settings from the user defaults. let defaults = UserDefaults.standard builder.pageTransition = PageTransition(rawValue: UInt(defaults.integer(forKey: "pageTransition")))! builder.pageMode = PageMode(rawValue: UInt(defaults.integer(forKey: "pageMode")))! builder.scrollDirection = ScrollDirection(rawValue: UInt(defaults.integer(forKey: "scrollDirection")))! builder.spreadFitting = PDFConfiguration.SpreadFitting(rawValue: defaults.integer(forKey: "spreadFitting"))! }
PSPDFConfiguration *configuration = [PSPDFConfiguration configurationWithBuilder:^(PSPDFConfigurationBuilder *builder) { // Restore the settings from the user defaults. NSUserDefaults *defaults = NSUserDefaults.standardUserDefaults; builder.pageTransition = [defaults integerForKey:@"pageTransition"]; builder.pageMode = [defaults integerForKey:@"pageMode"]; builder.scrollDirection = [defaults integerForKey:@"scrollDirection"]; builder.spreadFitting = [defaults integerForKey:@"spreadFitting"]; }];
For more details and sample code, please take a look at PersistViewSettingsExample.swift
from the Catalog app.