How Do I Customize the Options in the Annotation Inspector?
To customize the options in the annotation inspector, you’ll have to change the propertiesForAnnotations
of PDFConfigurationBuilder
. Use a dictionary containing an Annotation.Tool
. This dictionary should specify the annotation type as a key, and an array of strings — which lists the allowed properties — as the value.
Example 1
The example below shows how to customize annotation inspector options for ink annotations:
let configuration = PDFConfiguration { // Do not show color presets. var typesShowingColorPresets = $0.typesShowingColorPresets typesShowingColorPresets.remove(.ink) $0.typesShowingColorPresets = typesShowingColorPresets // Configure the properties for ink annotations to only show the color and blend mode setting in the annotation inspector. var properties = $0.propertiesForAnnotations properties[.ink] = [[AnnotationStyle.Key.color, AnnotationStyle.Key.blendMode]] $0.propertiesForAnnotations = properties }
PSPDFConfiguration *configuration = [PSPDFConfiguration configurationWithBuilder:^(PSPDFConfigurationBuilder *builder) { // Do not show color presets. PSPDFAnnotationType typesShowingColorPresetes = builder.typesShowingColorPresets; typesShowingColorPresetes = typesShowingColorPresetes &~ PSPDFAnnotationTypeInk; builder.typesShowingColorPresets = typesShowingColorPresetes; // Configure the properties for ink annotations to only show the color and blend mode setting in the annotation inspector. NSMutableDictionary<PSPDFAnnotationString, id> *propertiesForAnnotations = [builder.propertiesForAnnotations mutableCopy]; propertiesForAnnotations[PSPDFAnnotationStringInk] = @[@[PSPDFAnnotationStyleKeyColor, PSPDFAnnotationStyleKeyBlendMode]]; builder.propertiesForAnnotations = propertiesForAnnotations; }];
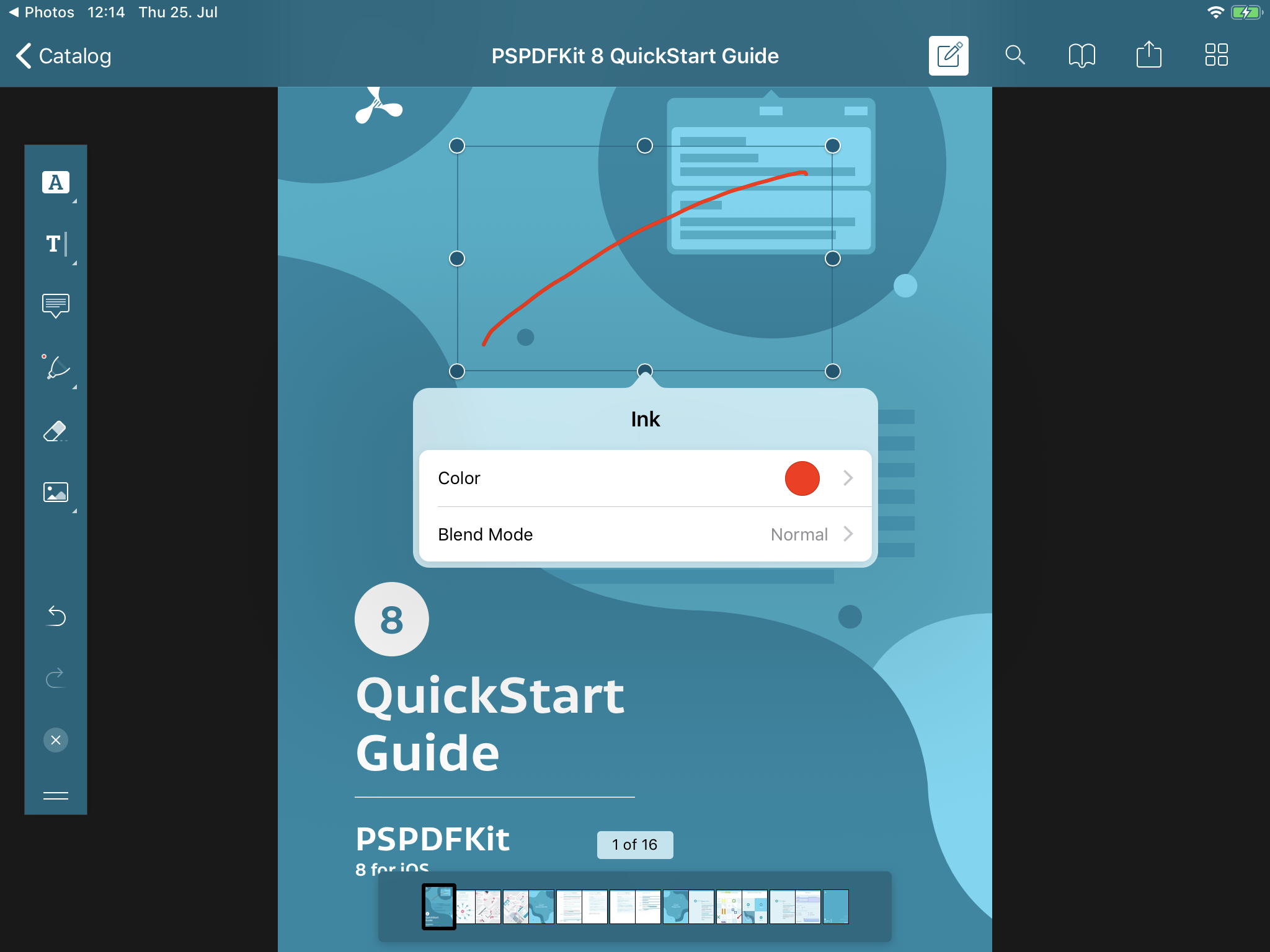
Example 2
This example illustrates how to remove the font name and size options from the annotation inspector for free text annotations:
let configuration = PDFConfiguration { // Configure the properties for free text annotations to hide the font name and size settings in the annotation inspector. var properties = $0.propertiesForAnnotations properties[.freeText] = [[AnnotationStyle.Key.textAlignment, AnnotationStyle.Key.color, AnnotationStyle.Key.fillColor, AnnotationStyle.Key.alpha]] $0.propertiesForAnnotations = properties }
PSPDFConfiguration *configuration = [PSPDFConfiguration configurationWithBuilder:^(PSPDFConfigurationBuilder *builder) {
// Configure the properties for free text annotations to hide the font name and size settings in the annotation inspector.
NSMutableDictionary<PSPDFAnnotationString, id> *propertiesForAnnotations = [builder.propertiesForAnnotations mutableCopy];
propertiesForAnnotations[PSPDFAnnotationStringFreeText] = @[@[PSPDFAnnotationStyleKeyTextAlignment, PSPDFAnnotationStyleKeyColor, PSPDFAnnotationStyleKeyFillColor, PSPDFAnnotationStyleKeyAlpha]];
builder.propertiesForAnnotations = propertiesForAnnotations;
}];
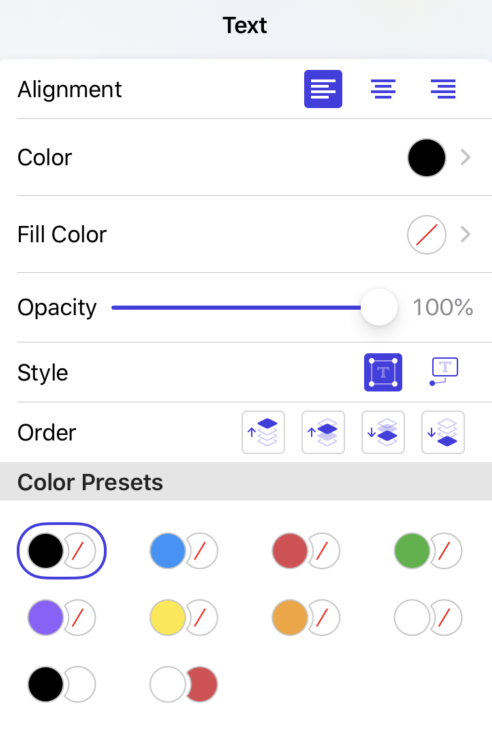
For more information, check out the AnnotationInspectorBlendModeStampExample.swift
and BlendModeMenuForMarkupAnnotationsExample.swift
examples from our PSPDFKit Catalog example app.