How to Generate a PDF in Blazor with PDFsharp
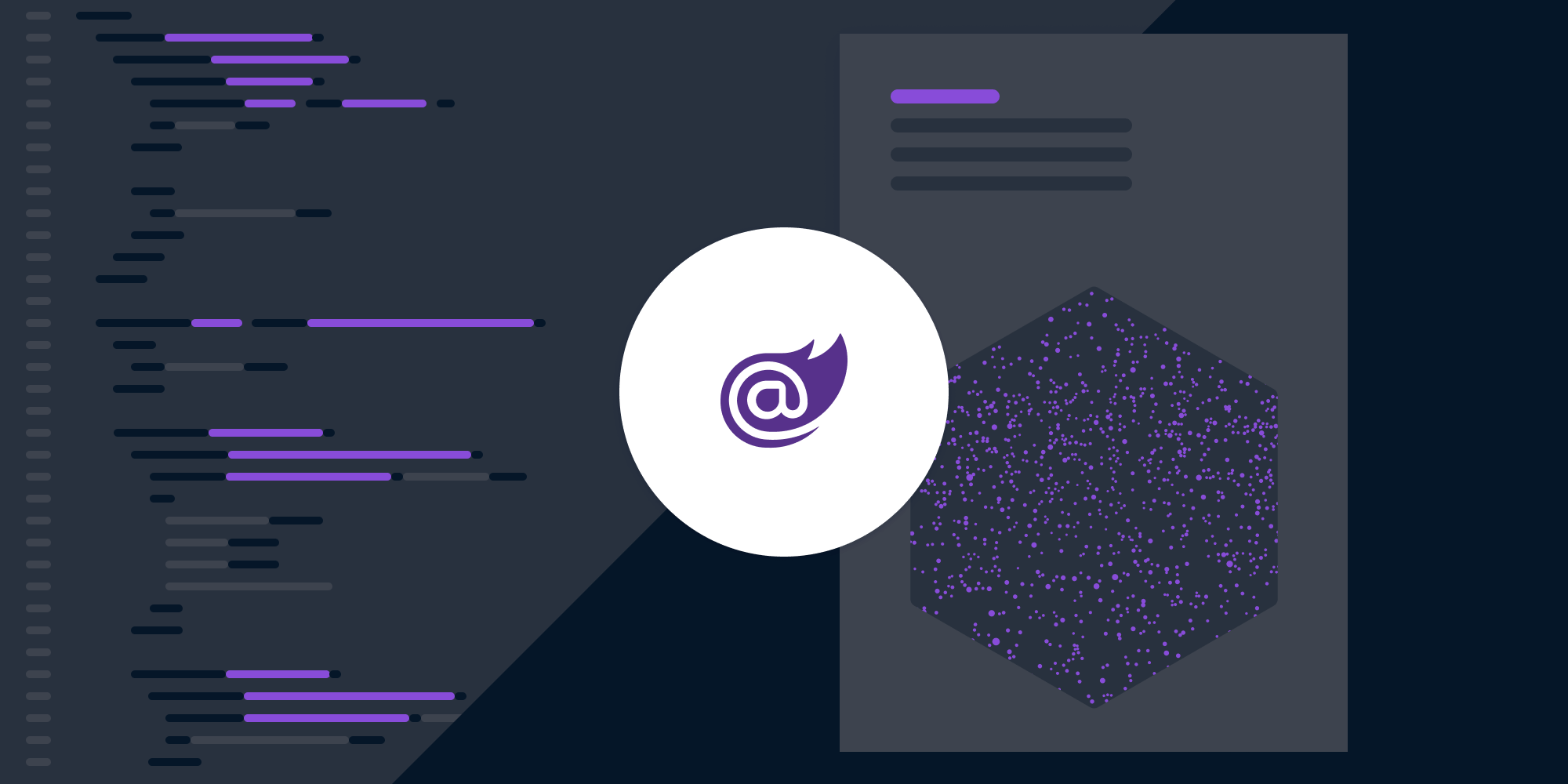
Blazor Server applications offer a dynamic way to work with web technologies using C# and .NET. In this blog post, we’ll demonstrate how to generate a simple PDF in a Blazor Server application using the PDFsharp library. Additionally, you’ll learn about PSPDFKit’s .NET PDF library, which offers more advanced features for complex .NET projects.
PDFsharp is a .NET library for processing PDF files, and it can be integrated into a Blazor project for this purpose.
Prerequisites
Step 1 — Setting Up the Blazor Server Project
-
Open Visual Studio and select File > New Project… to create a new project for your application.
-
Choose the Blazor Server App project template. Press Continue.
-
Choose .NET 7.0 for the target framework. Press Continue.
Your project must be .NET 6 or higher, as the .NET Framework is no longer supported.
-
When prompted, choose your app name (
GeneratePDFBlazor
) and use the default options. Then press Create.
Step 2 — Installing the PDFsharp Package
Now, install the PDFsharp package using the .NET CLI or NuGet Package Manager:
dotnet add package PDFsharp --version 6.0.0
For NuGet Package Manager:
-
Right-click on your project in the Solution Explorer.
-
Select Manage NuGet Packages.
-
Search for PDFsharp and add and install it.
Step 3 — Setting Up the PDF Generator
This section will walk you through setting up the PdfGenerator
class.
The PdfGenerator Class
To start, create a PdfGenerator
class. This class will handle the creation of the PDF document using PDFsharp.
In the root directory, create a new file named PdfGenerator.cs
and add the following code:
using PdfSharp; using PdfSharp.Pdf; using PdfSharp.Drawing; using PdfSharp.Fonts; using PdfSharp.Snippets.Font; public class PdfGenerator { public static byte[] CreatePdf() { using (var memoryStream = new MemoryStream()) { if (Capabilities.Build.IsCoreBuild) GlobalFontSettings.FontResolver = new FailsafeFontResolver(); // Create a new PDF document. PdfDocument document = new PdfDocument(); document.Info.Title = "Generated with PDFsharp"; // Create an empty page in this document. PdfPage page = document.AddPage(); // Get an XGraphics object for drawing on this page. XGraphics gfx = XGraphics.FromPdfPage(page); // Draw two lines with a red default pen. var width = page.Width; var height = page.Height; gfx.DrawLine(XPens.Red, 0, 0, width, height); gfx.DrawLine(XPens.Red, width, 0, 0, height); // Draw a circle with a red pen and a white brush. var radius = width / 5; gfx.DrawEllipse(new XPen(XColors.Red, 1.5), XBrushes.White, new XRect(width / 2 - radius, height / 2 - radius, 2 * radius, 2 * radius)); // Create a font. XFont font = new XFont("Times New Roman", 20, XFontStyleEx.BoldItalic); // Draw the text. gfx.DrawString("Hello, PDFsharp!", font, XBrushes.Black, new XRect(0, 0, page.Width, page.Height), XStringFormats.Center); // Save the document to memory stream. document.Save(memoryStream); return memoryStream.ToArray(); } } }
In this class, you’re using PDFsharp to create a PDF document, add a page, and draw some basic graphics and text. The final document is saved to a MemoryStream
, which is then returned as a byte array.
Step 4 — Creating the Blazor Interface
Now, you’ll create the Blazor interface.
The Index.razor File
In the Pages/Index.razor
file, define a simple UI that includes a button for generating and downloading the PDF:
@page "/" @inject IJSRuntime JSRuntime <h3>PDF Generator</h3> <button class="btn btn-primary" @onclick="DownloadPdf">Download PDF</button> @code { private async Task DownloadPdf() { byte[] pdfBytes = PdfGenerator.CreatePdf(); var fileName = "GeneratedPdf.pdf"; await JSRuntime.InvokeVoidAsync("saveAsFile", fileName, Convert.ToBase64String(pdfBytes)); } }
When the button is clicked, the DownloadPdf
method calls PdfGenerator.CreatePdf
to generate the PDF and uses JavaScript interop to initiate a file download in the browser.
JavaScript Interop in _Host.cshtml
In the Pages/_Host.cshtml
file, use JavaScript interop to create a Blob
from the byte array and trigger a download in the browser. Add the following script before the closing body
tag:
<script> window.saveAsFile = (filename, bytesBase64) => { var link = document.createElement('a'); link.href = 'data:application/octet-stream;base64,' + bytesBase64; link.download = filename; document.body.appendChild(link); link.click(); document.body.removeChild(link); }; </script>
This script creates a temporary link element, sets its href
to the base64
-encoded PDF data, and triggers a click to start the download.
Step 5 — Running the Application
In Visual Studio, press F5 or click Run. Or, you can run the following command in the terminal:
dotnet run
Step 6 — Interacting with the Application
Interact with your application in the browser. Click the button (or perform the action you set up) to trigger the PDF generation. The PDF will be generated and then downloaded by the browser.
The PDFsharp library offers a wide range of capabilities for creating complex PDFs, while Blazor and JavaScript interop make it easy to deliver these documents to your users. This basic setup can be expanded and customized to meet the specific needs of your application.
![]()
You can find the full source code for this tutorial in our GitHub repository.
PSPDFKit GdPicture.NET Library
While both PDFsharp and the PSPDFKit GdPicture.NET library offer PDF generation capabilities for .NET applications, PSPDFKit GdPicture.NET library stands out as a more comprehensive and feature-rich solution. Here are some reasons why PSPDFKit GdPicture.NET library can be considered better:
-
Extensive Capabilities — PSPDFKit GdPicture.NET library provides a wide range of functionalities, including OCR, barcode processing, PDF editing, document conversion, scanning, and image processing. It outperforms many other .NET document imaging technologies available on the market.
-
OCR (Optical Character Recognition) — PSPDFKit GdPicture.NET library supports OCR, which enables the extraction of text from scanned documents and images. This feature is essential for applications that require text recognition and data extraction from PDFs.
-
Advanced PDF Editing — PSPDFKit GdPicture.NET library offers more advanced PDF editing capabilities, such as editing text, images, and annotations, as well as adding watermarks, digital signatures, and form fields to PDF documents.
-
Comprehensive Support — PSPDFKit has a reputation for providing excellent customer support and regular updates to address any issues or introduce new features. This ensures that your application remains up to date and well-maintained.
-
Integration-Friendly — PSPDFKit GdPicture.NET library is designed to seamlessly integrate into your existing .NET applications, making it easier to adopt and implement in your projects.
-
Documentation and Resources — PSPDFKit offers comprehensive documentation, tutorials, and sample code, making it easier for developers to get started and leverage the library’s full potential.
To get started with the PSPDFKit GdPicture.NET library, follow our getting started guide.
Conclusion
This tutorial demonstrated how to use the PDFsharp library for basic PDF generation in Blazor Server applications. PDFsharp is an excellent open source tool for creating and manipulating PDFs in .NET, ideal for straightforward tasks like drawing text and graphics in documents.
For more advanced features, however, consider the PSPDFKit GdPicture.NET library. It extends capabilities to include OCR, PDF editing, barcode processing, and document conversion. This makes it a comprehensive solution for complex Blazor projects that require robust document processing functionalities. Whether you need simplicity with PDFsharp or advanced features with GdPicture.NET, both libraries offer powerful ways to handle PDFs in Blazor applications.
To see a list of all frameworks, you can contact our Sales team. Or, launch our demo to see our viewer in action.