How to Convert HTML to PDF in React Using html2pdf
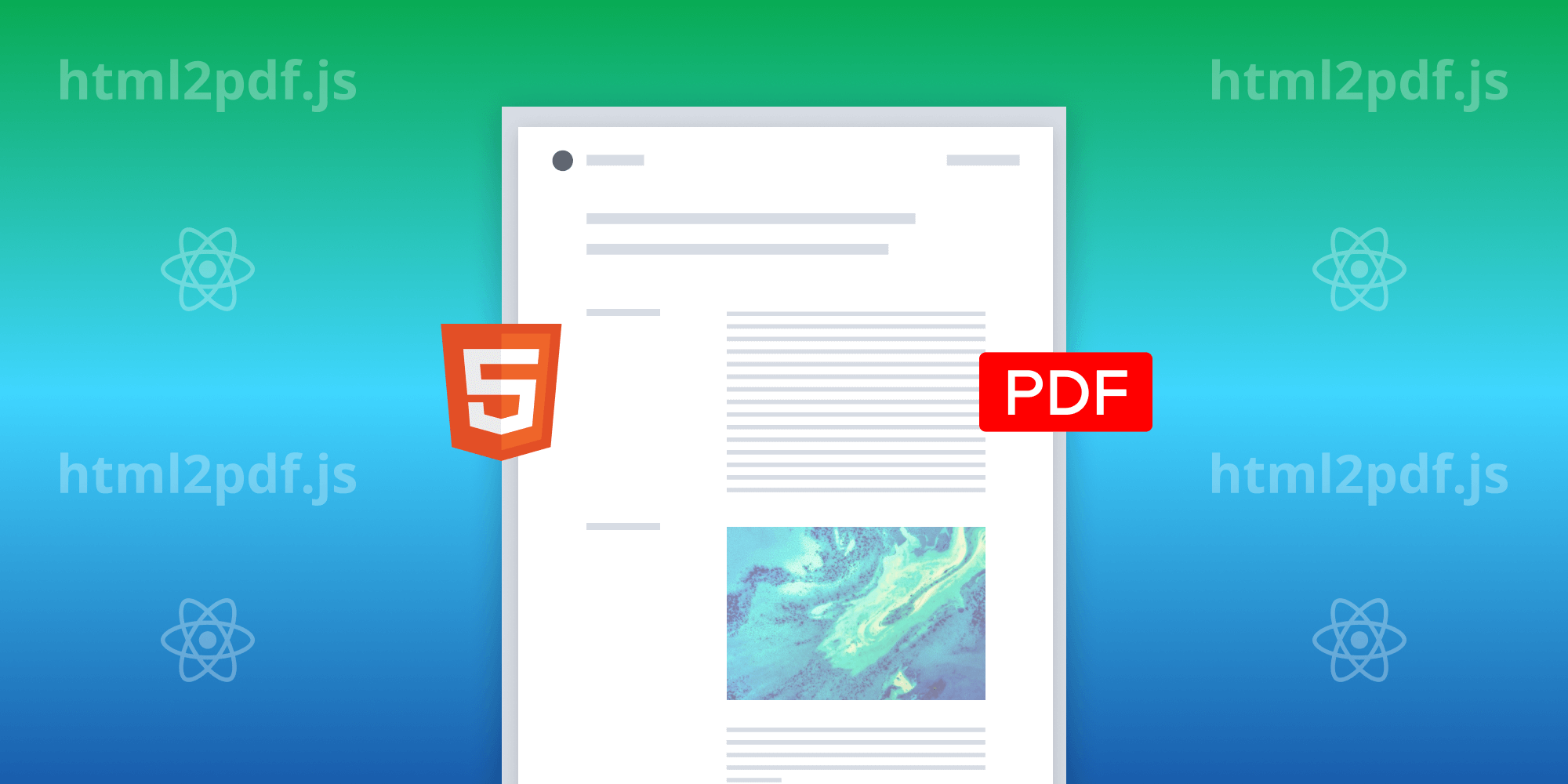
In today’s digital age, document sharing and printing often involve the use of PDF files. Whether you need to generate invoices, reports, or any other printable document from your web application, the ability to convert HTML content to a PDF format is a valuable feature. In this tutorial, you’ll explore how to achieve this using React and the html2pdf.js library.
Prerequisites
Before starting, make sure you have the following prerequisites:
Setting Up a React Application
Begin by creating a new React application if you don’t already have one. You’ll use Create React App to set up your project:
npx create-react-app html2pdf-react-app cd html2pdf-react-app
Installing html2pdf.js
html2pdf.js is a powerful JavaScript library that simplifies the process of converting HTML content to a PDF document. To install it, run the following command inside your project directory:
npm install html2pdf.js
With html2pdf.js in place, you can start building your PDF converter.
Creating the PDF Converter Component
In your React application, you’ll create a component named PdfConverter
, which is responsible for converting HTML content to PDF.
-
Start by importing the
useRef
hook from the React library, which will help you reference the HTML content you want to convert. Additionally, import the html2pdf library, which enables HTML-to-PDF conversion:
import { useRef } from 'react'; import html2pdf from 'html2pdf.js';
-
To customize the PDF conversion process, define an
options
object. This object will hold various settings that affect how the PDF is generated. You have the flexibility to specify options such as the PDF file name, margins, image quality, paper size, and more.
Here’s an example of defining the options
object:
const options = { filename: 'my-document.pdf', margin: 1, image: { type: 'jpeg', quality: 0.98 }, html2canvas: { scale: 2 }, jsPDF: { unit: 'in', format: 'letter', orientation: 'portrait' }, };
In this example, you set the file name to ‘my-document.pdf’, use JPEG images with 98 percent quality to generate the PDF, and configure other settings like margins and paper size. However, feel free to adjust these options according to your requirements.
html2pdf.js provides many more configuration options and customization features you can explore in its documentation.
-
Now, define a function named
convertToPdf
. This function will be responsible for converting the HTML content to a PDF when triggered by a button click. Inside this function, you’ll retrieve the HTML content you want to convert using theuseRef
hook:
const contentRef = useRef(null); const convertToPdf = () => { const content = contentRef.current; };
contentRef
is a reference to the HTML content you want to convert, and content
contains that content.
-
Use the
html2pdf
library to initiate and configure the PDF conversion process within theconvertToPdf
function. Call thehtml2pdf()
function, chain it with the.set(options)
method to apply the conversion options, specify the HTML content to convert using.from(content)
, and then trigger the conversion and save the PDF using.save()
:
const contentRef = useRef(null); const convertToPdf = () => { const content = contentRef.current; html2pdf().set(options).from(content).save(); };
This function takes care of setting up the conversion, specifying the content, and generating the PDF.
-
Return the JSX structure for your component. Include the HTML content you wish to convert and a button labeled Convert to PDF, and set up an
onClick
event handler to call theconvertToPdf
function when the button is clicked:
return ( <div> <div ref={contentRef}> {/* Your HTML content that you want to convert to PDF */} <h1>Hello, PDF!</h1> <p> This is a simple example of HTML-to-PDF conversion using React and html2pdf. </p> </div> <button onClick={convertToPdf}>Convert to PDF</button> </div> );
Here’s the full code:
import { useRef } from 'react'; import html2pdf from 'html2pdf.js'; const PdfConverter = () => { const contentRef = useRef(null); const convertToPdf = () => { const content = contentRef.current; const options = { filename: 'my-document.pdf', margin: 1, image: { type: 'jpeg', quality: 0.98 }, html2canvas: { scale: 2 }, jsPDF: { unit: 'in', format: 'letter', orientation: 'portrait', }, }; html2pdf().set(options).from(content).save(); }; return ( <div> <div ref={contentRef}> {/* Your HTML content that you want to convert to PDF */} <h1>Hello, PDF!</h1> <p> This is a simple example of HTML-to-PDF conversion using React and html2pdf. </p> </div> <button onClick={convertToPdf}>Convert to PDF</button> </div> ); }; export default PdfConverter;
Integrating the PDF Converter Component
To use the PdfConverter
component in your React application, import and render it in your main component (src/App.js
):
import './App.css'; import PdfConverter from './PdfConverter'; function App() { return ( <div> <header> <h1>HTML-to-PDF Conversion with React</h1> </header> <main> <PdfConverter /> </main> </div> ); } export default App;
Running Your React Application
You’re now ready to run your React application:
npm start
Open your browser, and you’ll see your HTML content, along with a Convert to PDF button. Clicking this button will generate a PDF version of the content and open it in a new browser window, and it’ll be ready for download or printing.
Pros and Cons of Using html2pdf for Converting HTML to PDF
As with anything, there are both pros and cons to this conversion solution.
Pros
-
Easy to use — html2pdf is a straightforward library that allows you to convert HTML to PDF using a few lines of JavaScript code.
-
No server-side processing required — Since html2pdf is entirely client-side, you don’t need to rely on any server-side processing or dependencies. This makes it a great option if you don’t have access to server-side resources or you want to reduce server load.
-
Customizable options — html2pdf provides many customization options, such as paper size, page orientation, margins, image quality, and more. This allows you to generate PDFs that meet your specific needs.
-
Open source — html2pdf is an open source library, which means you can use it for free and contribute to its development if you wish.
Cons
-
Limited support for advanced PDF features — html2pdf may not support some advanced PDF features, such as embedded fonts, annotations, or form fields. For more complex PDF generation tasks, a server-side library like wkhtmltopdf or a more powerful client-side library like pdfmake might be more suitable.
-
Performance issues — Since html2pdf relies on html2canvas to take screenshots of webpages and convert them to a canvas, the conversion process can be slow and resource-intensive, especially for large or complex documents.
-
Dependence on external libraries — html2pdf relies on other libraries (html2canvas and jsPDF) for its functionality. While this approach simplifies the code and reduces maintenance, it can also introduce potential compatibility issues or limitations from the underlying libraries.
-
Incomplete support for CSS and JavaScript — html2pdf may not render certain CSS styles or JavaScript-driven content accurately. This limitation can be problematic if your HTML content relies heavily on advanced CSS or dynamic content generated through JavaScript.
Alternatives to html2pdf.js
While html2pdf.js is a popular option for converting HTML to PDF, there are several other libraries and tools available for this purpose. Below are some of the alternatives to consider.
-
wkhtmltopdf — This is a command-line tool that uses the WebKit rendering engine to convert HTML to PDF. It supports advanced features such as headers and footers, tables of contents, and page numbering. However, it requires server-side processing and may not be as customizable as html2pdf.js.
-
Puppeteer — This is a Node.js library that provides a high-level API for controlling headless Chrome or Chromium browsers. It can be used to generate PDFs from HTML content, and it supports advanced features such as page breaks, headers and footers, and tables of contents. However, it requires server-side processing and may not be as lightweight as html2pdf.js.
-
PSPDFKit — We offer a couple of options for converting HTML to PDF:
-
HTML-to-PDF API — A REST API and hosted solution that provides 100 free conversions per month and offers additional packages for a per-document fee. This option allows you to integrate HTML-to-PDF conversion into your applications without the need for self-hosting or a server setup.
-
PDF Generation SDK — This allows you to generate a PDF from scratch, leveraging HTML and CSS technologies for highly flexible designs and context-customized PDFs for your users.
-
If you’re interested in PSPDFKit, you can contact our Sales team.
Conclusion
Overall, html2pdf.js is a useful client-side library for generating PDFs from HTML content, offering simplicity and customization. However, for advanced features and a more comprehensive solution, consider PSPDFKit as a robust alternative. PSPDFKit offers server-side and hosted options, flexible PDF generation, and API integrations.
To get started, you can:
-
Start your free trial to test the library and see how it works in your application.
-
Launch our demo to see the viewer in action.