How to Merge Two or More PDFs on Windows
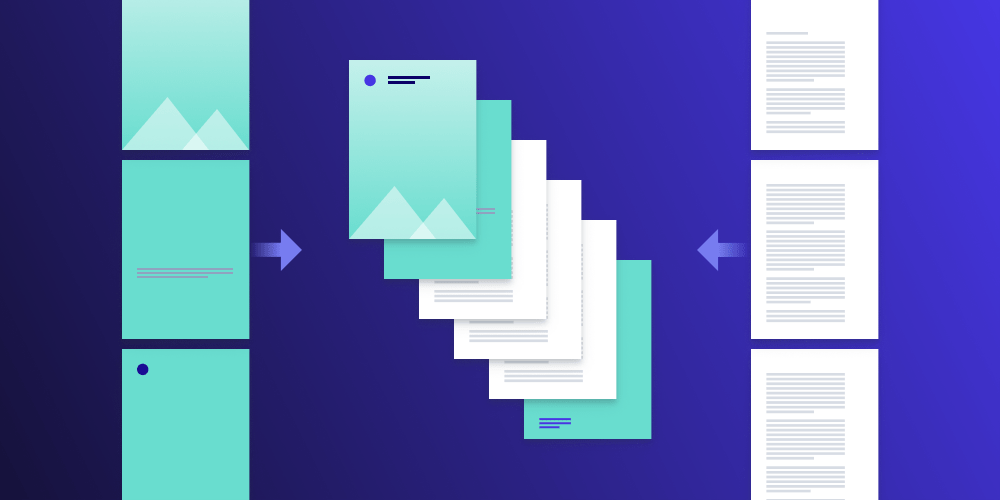
There are many situations where content spread across multiple pages or documents needs to be collated into a single document. Examples of this content include board meeting reports; brochure, factsheet, and product manual production; and interview preparation. This is the kind of task that most PDF editors — like PDF Viewer, Adobe Acrobat, and Apple’s Preview — support in some form.
One could approach this task by using the UWP Windows.Data.Pdf
API to load pages, render them to images, and add them to the visual tree as UI elements before printing them as PDF pages. However, this has significant problems, e.g. all images need to be in memory, the output PDF would contain resolution-limited bitmap renders of each page, and user interaction is always required.
In this post, we’ll cover how PSPDFKit for Windows lets you merge two or more PDFs — either visually in the UI or entirely programmatically in code — while maintaining pages in their original PDF data form. With this approach, you can merge entire PDF files directly into a new document without having to render every single page of each PDF separately. In this way, metadata, bookmarks, and other document-based information will be preserved in the new document. PSPDFKit also automatically handles merging rotated pages without the user having to manually adjust anything. And most importantly, annotations will be persisted in the merged document as well.
Merging Visually
Let’s say we’ve got two documents, each consisting of several pages. The first document has front and back covers, a front page, and a back page, and we want to merge the contents of the second document into the first one, placing it between the front and back pages of the first document. The video above demonstrates this process.
First, we open the document with covers. Then we start the visual Document Editor. Next, we select the page where the imported document’s pages will be placed. Then we import the second document. The Document Editor will show a stack of pages and where they will go. This can be moved around with controls if necessary. Finally, we can save this as a new document. It’s as simple as that!
Automated Merging
Let’s now assume you have a frequent need to merge documents and you don’t want to do your merging manually. In that case, you can write code to automate the process — perhaps to run on a server that’s batch processing your documents.
Here’s how to easily accomplish this:
// Open two documents from `StorageFiles`. var firstDoc = DocumentSource.CreateFromStorageFile(fileOne); var secondDoc = DocumentSource.CreateFromStorageFile(fileTwo); var newFile = await Editor.MergePDFsAsync(new List<StorageFile>{ firstDoc, secondDoc });
With a few lines of simple code, it’s possible to merge two or more documents together and write the result out to a new file.
If you need to control the placement of pages precisely — for example, if you have complex dynamic rules about which pages need to be copied — then you can use the Document Editor Job
.
The equivalent code for the above example could look like this:
// Create a job from a source document. var job = new PSPDFKit.Document.Editor.Job(documentOne); // For every page in a second source document, make a new page and add it to the job. var docOnePageCount = await documentOne.GetTotalPageCountAsync(); var docTwoPageCount = await documentTwo.GetTotalPageCountAsync(); for (var pageIndex = 0; pageIndex < docTwoPageCount; pageIndex++ ) { // Create a new page copied from the second document at the page index. var newPage = NewPage.FromPage(documentTwo, pageIndex); // Append it to the job's document. await job.AddPageAtIndexAsync(newPage, docOnePageCount + pageIndex); } // Create a new document and get its `StorageFile`. var newDocument = await Editor.NewStorageFileFromJobAsync(job);
Note that if you simply want to append one document to another, the first example offers better performance, not to mention it requires far less code.
With the Document Editor, it’s also possible to stack as many of these operations together as needed. One factor to be aware of is the size of the document in memory. Opening and appending documents can be a memory-intensive process, so if you’re importing large documents, keep this in mind.
Extra Operations
The code above shows the use of the powerful PSPDFKit Document Editor, which offers more processing facilities — including rotating pages, moving pages, and editing page labels.
But let’s say we want to make flip cards now. We have a page on one side, and if we flip it on the horizontal edge, we have another page, but in the correct readable orientation. With the previous example, if we did this, the second page would be upside-down.
We can fix this:
// Create a job from a source document that happens to have one page. var job = new PSPDFKit.Document.Editor.Job(dogDocument); // Create a new page copied from the first page of the second document. var newPage = NewPage.FromPage(catDocument, 0); // Append it to the job's document. await job.AddPageAtIndexAsync(newPage, 1); // Rotate the new page. await job.RotatePageAsync(1, Rotation.Degrees180); // Create a new document. var newDocument = await Editor.NewStorageFileFromJobAsync(job);
We take the second page and rotate it 180 degrees. Now, if we’re looking at the first page and flip it along the horizontal edge, the second page will appear in the correct orientation! We’ve made flip cards.
From here, it’s easy to explore additional possibilities. You can examine the various methods available on Job
in our merging guide, or refer to the API to read more about PSPDFKit.Document.Editor.Job
.
In the code examples in this blog, we worked with files, but it’s also possible to load Document
s from any source you require — such as memory, network data, or even a cryptographic solution — using an implementation of IDataProvider
.
Conclusion
In this post, you learned how to easily create documents by selective merging from a source document either visually or programmatically using PSPDFKit for Windows. You also learned how extra operations can be applied to the newly created document. Feel free to explore the guides and API documentation for more examples and other important features of the SDK.
If you’d like to try PSPDFKit for Windows for yourself, head over to the trial page and download the SDK today.