PDF Form Filling and Reading in .NET
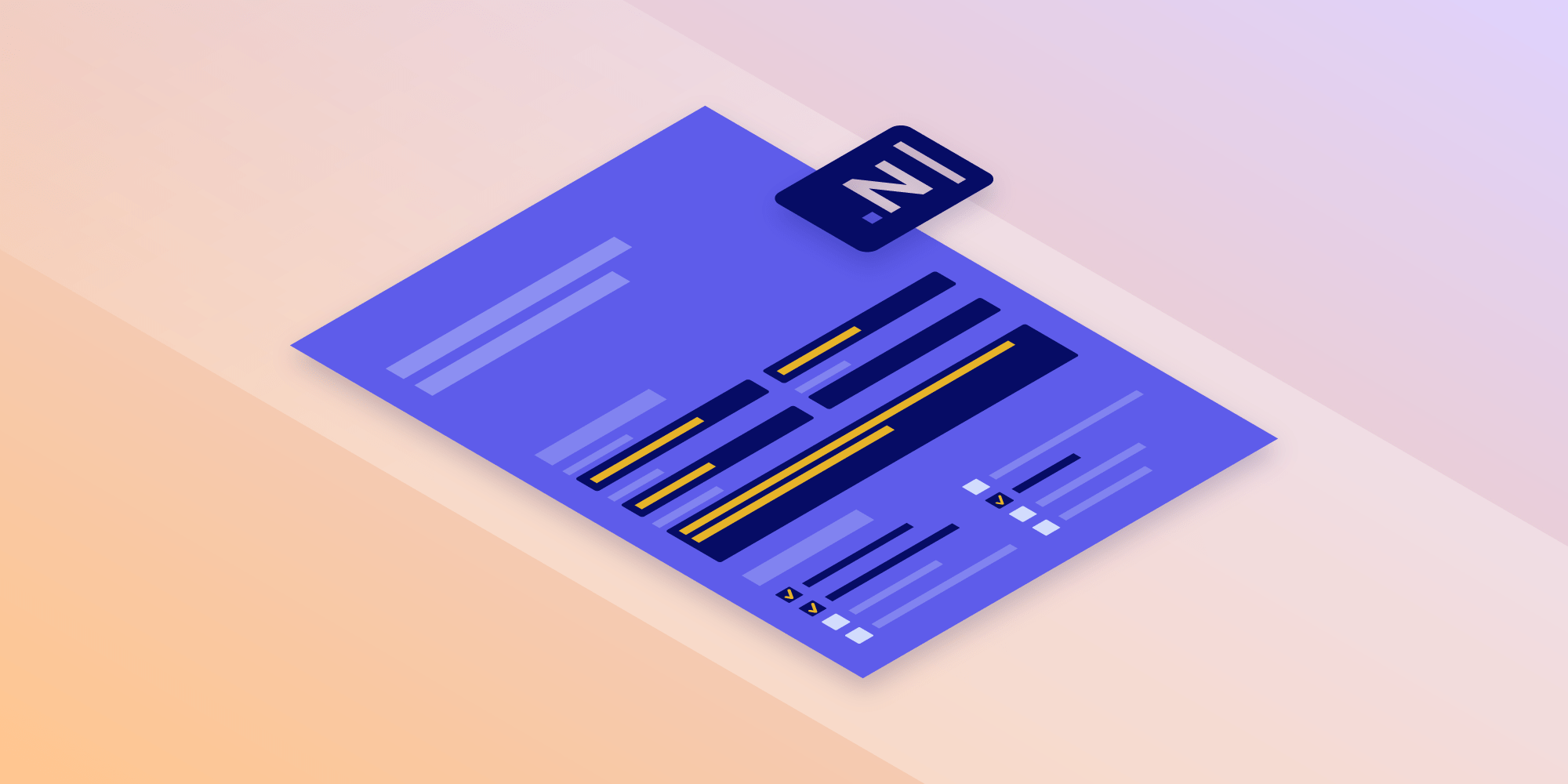
There are many reasons why businesses of all sizes prefer to use PDFs for document transfers when working across teams and even externally.
Let me give you one hypothetical example to explain why.
Meet Ted. Ted is a developer for your company. He’s an avid FreeBSD user who has never opened a Google account and uses a flip phone from the 90s (and not because it’s cool). He refuses to buy in to the new age, big data model of “your personal information pays for the product.”
Now meet Sammy. She’s a high-flying sales person for your company who sports the newest MacBook Pro, iPad, and iPhone. She’s willing to adopt any new technology that may well be the latest and greatest of our time, but she is stuck in the Apple ecosystem.
Now imagine you want to send out a form for people to fill out. So you set up a Google form for which you expect a single response from each employee. But now you encounter an issue. If you want one response, and one response only, from each employee, said employee has to have a Google account. Ted is out. And Sammy is too invested in the Apple ecosystem to handle multiple accounts across different companies. We’ve hit a flexibility issue.
In situations like the one detailed above, PDF is the one standard you can rely upon. It allows people to view documents on most devices you can think of (OK, maybe not Ted’s flip phone). And in many environments, forms can be filled out and saved documents can be forwarded on to their next destination.
Today I’m going to continue with this hypothetical example and pretend I’ve received the filled-out PDFs from Ted and Sammy. I’m going to read back the information they’ve given for further analysis. I’ll then show you how to prefill some form data in the document to make it just a bit easier for Ted and Sammy.
If you’re not too sure what PDF forms are or how to use them, you can jump over to our Forms in PDF blog post.
In this blog post, I’m going to use the PSPDFKit .NET Library with C#. You may find an environment or setup in .NET Core, the .NET framework, or ASP.NET.
Opening the Document
Before reading back the form fields, we have to open the document to work on:
var document = new PSPDFKit.Document(new FileDataProvider("Form_Example.pdf"));
Reading Back the Form Fields
To read back all the form field values for a given document, there’s one simple method call. The returning data will describe the values in a JSON key-value pair, where the key is the name of the form field and the value stands for the values set.
Because JSON is widely supported on many platforms and in many environments, it’s simple to either directly forward this JSON object elsewhere or parse it in place with a library like Newtonsoft:
var fieldValuesJson = document.GetFormProvider().GetFormFieldValuesJson();
Imagine we have a document that requests the following data from Ted and Sammy:
-
First Name
-
Last Name
-
DOB
-
Address
-
Telephone Number
We can use GetFormFieldValuesJson
to pull Ted’s data out from the document he sent over, and we’d receive the following:
{ "Name_First": "Ted", "Name_Last": "Smith", "Birthdate": "03/11/1972", "Address_1": "1234 Drive Drive", "Telephone_Home": "+123 123 1234" }
From this point, we could validate the data, save it into a database, or even fill out another PDF form 😛.
Filling Out a PDF Form
Even before we sent out the forms, we already had information about Ted and Sammy. So why not make it a little easier for them and fill out the fields we already know?
We can fill out form information with SetFormFieldValuesJson
. The method uses the exact same key-value model as we saw with GetFormFieldValuesJson
, so let’s look at filling out Sammy’s information:
var fieldValuesJson = new JObject { {"Name_First", "Sammy"}, {"Birthdate", "12/12/1995"} }; document.GetFormProvider().SetFormFieldValuesJson(fieldValuesJson); document.Save(new DocumentSaveOptions());
We can now send the saved document directly to Sammy, and her first name and birthday are already filled out. How useful!
Conclusion
The fact that it took me longer to set up the hypothetical example than it did to introduce the API to you shows how simple form getting/setting is in the PSPDFKit .NET Library. Using the power and flexibility of PDFs in your data collection process allows you to become OS and environment agnostic, thereby letting your employees or customers work in their most efficient ways.
By pairing the flexibility of PDF with the many environments of .NET, you’ll be sure to find a solution that will be quick to set up and deploy.
To further this example, once you’ve written out your form field values to a PDF, you are then free to manipulate the PDF how you’d like. For example, the PSPDFKit .NET Library offers features like document editing, which lets you add, remove, and move pages around, and even merge one or more documents together. You could even dive into the powerful Redaction feature, which allows you to automate the removal of personal information like Social Security numbers, dates, and phone numbers — permanently removing data from the document and ensuring it’s irrecoverable.
To find out more about form filling with the PSPDFKit .NET Library and the many other features supported by the library, why not download the free trial?
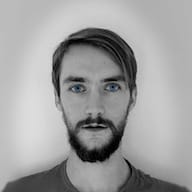
When Nick started tinkering with guitar effects pedals, he didn’t realize it’d take him all the way to a career in software. He has worked on products that communicate with space, blast Metallica to packed stadiums, and enable millions to use documents through PSPDFKit, but in his personal life, he enjoys the simplicity of running in the mountains.