How to Fill PDF Forms in SharePoint Online Using PSPDFKit
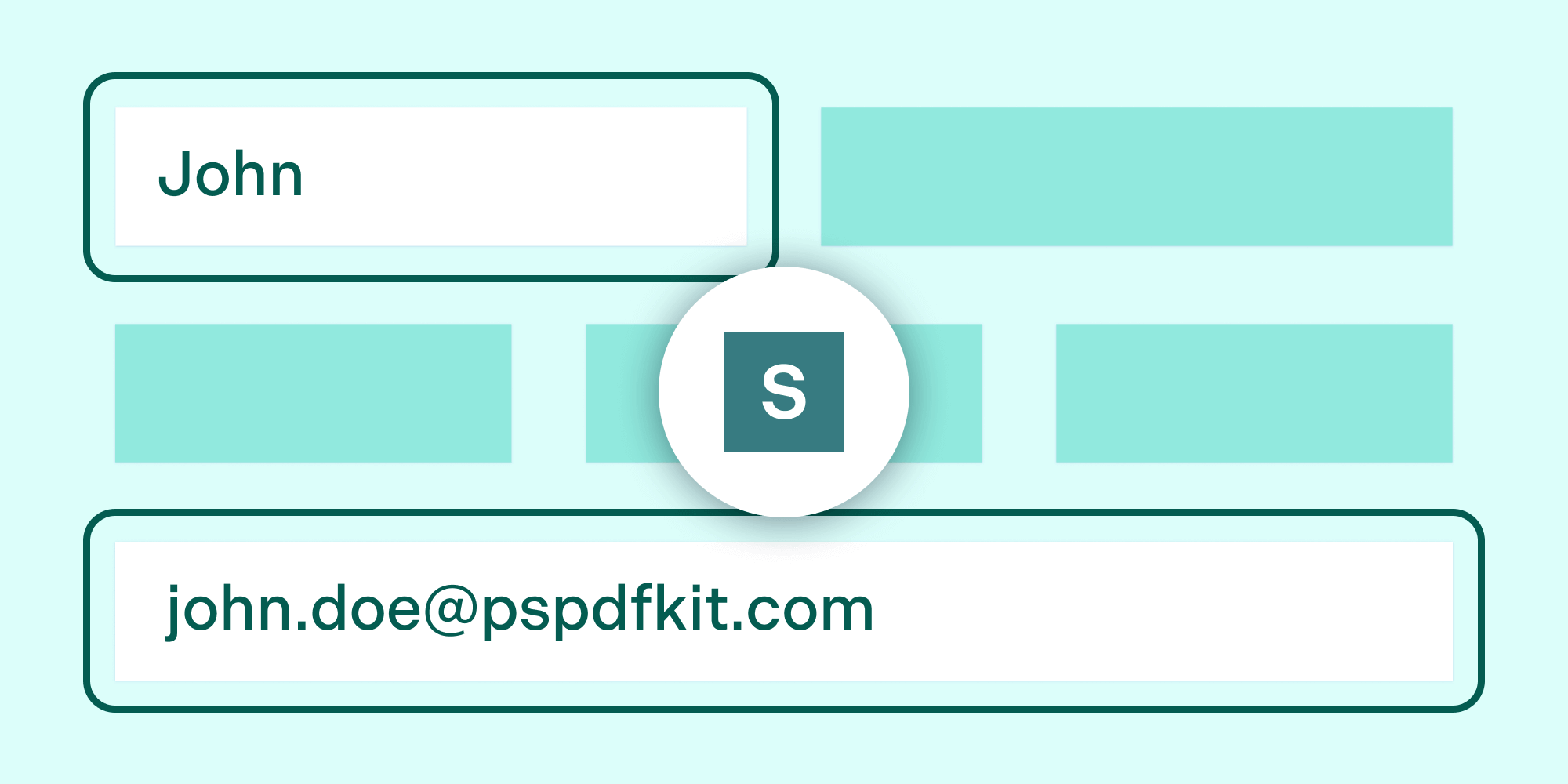
In this tutorial, you’ll learn how to add your data into a fillable PDF form in SharePoint Online. Using the PSPDFKit for Web integration for SharePoint, you can view, create, and automate PDF form filling directly in your SharePoint browser.
Integrating PSPDFKit for Web into SharePoint Online as a Web Part
PSPDFKit for SharePoint is built on top of PSPDFKit for Web Standalone, a client-side JavaScript library for viewing and editing PDF documents in a web browser. It shares the same APIs as PSPDFKit for Web Standalone, so refer to the Web documentation for customizing your SharePoint application.
Requirements
-
A Microsoft 365 tenant (sign up for the Microsoft 365 Developer Program to set up a Microsoft 365 tenant for free)
-
The SharePoint Framework (SPFx)
-
A GitHub account (for cloning the PSPDFKit for SharePoint repository)
Setup
-
Clone the
pspdfkit-sp-online-webpart
repository from GitHub by navigating to the desired directory and entering the following command in your command line/terminal:
git clone https://github.com/PSPDFKit/pspdfkit-sp-online-webpart.git
Alternatively, download the project as a .zip
file.
-
Sign in to your tenant account at admin.microsoft.com.
Click Show all in the side navigation, and find Admin centers > SharePoint to access the SharePoint admin portal.
Follow this Microsoft tutorial to configure your SharePoint tenant and create a new team site.
-
In the
pspdfkit-sp-online-webpart
project’sconfig/serve.json
file, replaceenter-your-SharePoint-site
with your SharePoint site’s URL:
"initialPage": "https://enter-your-SharePoint-site/_layouts/workbench.aspx",
-
Set up your development environment to build a client-side web part.
Remember to run gulp trust-dev-cert
from the project’s root to trust the self-signed developer certificate.
-
Change your directory to the
pspdfkit-sp-online-webpart
directory:
cd pspdfkit-sp-online-webpart
-
Check your Node.js version:
node --version
Ensure you have Node.js version 16.19.0 installed. If not, switch to this version using nvm (Node Version Manager) or asdf.
-
Install the dependencies:
npm install
-
Serve the project:
npm run serve
Your SharePoint account will open in a new browser tab.
Integrating PSPDFKit into SharePoint
You can add PSPDFKit to your project as a widget using the user interface (UI) elements. Follow the video tutorial or the instructions below to integrate the PSPDFKit widget into your project.
-
On your Home page, click Edit in the upper right-hand corner to open the editing interface.
-
Hover over the desired location for the PSPDFKit widget and click the plus icon that appears. This opens a dialog box with the available web parts.
-
Search for the PSPDFKit widget and select it.
-
Click the pencil icon’s Edit web part button and click Select Document from the open panel.
-
Choose your document and click Open. The PSPDFKit widget will now appear in your project.
Form Filling with PSPDFKit
PSPDFKit offers comprehensive capabilities for filling forms, providing both a user-friendly interface and programmable alternatives.
-
UI form filling — PSPDFKit’s prebuilt UI components enable users to effortlessly navigate and interact with PDF forms. They can fill in text fields, select options from dropdown menus, and interact with checkboxes and radio buttons. See it in action by exploring the demo.
-
Programmatic form filling— PSPDFKit for Web offers versatile options for programmatic form filling:
-
PSPDFKit Server — Easily persist, restore, and synchronize form field values across devices without building something yourself.
-
XFDF — Seamlessly exchange form field data with other PDF readers and editors.
-
Instant JSON — Efficiently export and import changes made to form fields.
-
Manual API — Have complete control over extracting, saving, and manipulating form field values.
-
In addition, PSPDFKit also provides an option for creating PDF forms:
-
PDF Form Creator — Simplify PDF form creation with a point-and-click UI. You can create PDF forms from scratch using an intuitive UI or via the API. Convert static forms into fillable forms, or modify existing forms by letting your users create, edit, and remove form fields in a PDF.
These options provided by PSPDFKit offer flexibility for custom workflows, data interoperability, and efficient form filling and form creation processes.
Filling Forms with XFDF
The XML Forms Data Format (XFDF) is a standard format for representing form data in PDF documents. It allows you to import and export form field values, making it an ideal choice for integrating form filling capabilities with PSPDFKit.
To import data into PDF forms using XFDF, open the src/webparts/PSPDFKit/components/PSPDFKitViewer.tsx
file and modify the PSPDFKit.load()
function as follows:
const XFDF = `<?xml version="1.0" encoding="UTF-8"?> <xfdf xml:space="preserve" xmlns="http://ns.adobe.com/xfdf/"> <annots></annots> <fields> <field name="Form field 1"> <value>Text for form field 1</value> </field> </fields> <ids modified="8AFBEA0B224C4F2BAE69171EF4FCE3C0" original="CC968B2893024520A316261F10B8978C"/> </xfdf> `; PSPDFKit.load({ XFDF, });
The code above demonstrates how to prefill the form field named “Form Field 1” with the text “Text for form field 1” using the PSPDFKit.load()
method.
You can also import form field values as XFDF using document operations, available with the Document Editor license component:
const xfdf = `<?xml version="1.0" encoding="UTF-8"?> <xfdf xml:space="preserve" xmlns="http://ns.adobe.com/xfdf/"> <annots></annots> <fields> <field name="Form field 1"> <value>Text for form field 1</value> </field> </fields> <ids modified="8AFBEA0B224C4F2BAE69171EF4FCE3C0" original="CC968B2893024520A316261F10B8978C"/> </xfdf> `; instance.applyOperations([ { type: 'applyXfdf', xfdf, }, ]);
In this code snippet, the applyOperations()
method is used to apply XFDF data to the PDF form fields.
Filling Forms with Instant JSON
To import data into PDF forms using Instant JSON in PSPDFKit, you can use the following code snippets.
Open the src/webparts/PSPDFKit/components/PSPDFKitViewer.tsx
file and modify the PSPDFKit.load()
function as follows:
instance = await PSPDFKit.load({ // Container where PSPDFKit should be mounted. container, // The document to open. document: documentURL, toolbarItems: [...PSPDFKit.defaultToolbarItems, saveItem], instantJSON: { format: 'https://pspdfkit.com/instant-json/v1', formFieldValues: [ { name: 'Form Field 1', value: 'Text for form field 1', type: 'pspdfkit/form-field-value', v: 1, }, // Add more form field values as needed. ], }, });
Document Editor license component — Use the applyOperations
method to import Instant JSON data. Modify the code inside the PSPDFKit.load()
method as follows:
instance = await PSPDFKit.load({ container, document: documentURL, toolbarItems: [...PSPDFKit.defaultToolbarItems, saveItem], }); // Import Instant JSON data. instance.applyOperations([ { type: 'applyInstantJson', instantJson: { format: 'https://pspdfkit.com/instant-json/v1', formFieldValues: [ { name: 'Form Field 1', value: 'Text for form field 1', type: 'pspdfkit/form-field-value', v: 1, }, // Add more form field values as needed. ], }, }, ]);
Make sure to adjust the formFieldValues
array with the desired form field names and values you want to prefill.
With the updated code and the instructions above, you’ll be able to use Instant JSON to prefill form field values in your PSPDFKitViewer
component.
Conclusion
In this tutorial, you learned how to integrate PSPDFKit into SharePoint Online as a web part and how to fill PDF forms using PSPDFKit’s SDK.
By incorporating PSPDFKit into your SharePoint application, you can streamline document management, automate form filling processes, and enhance the overall user experience. PSPDFKit’s robust features and seamless integration make it a valuable tool for organizations looking to optimize their PDF workflows within SharePoint Online.
To see a list of all web frameworks, contact our Sales team. Or, launch our demo to see our viewer in action.